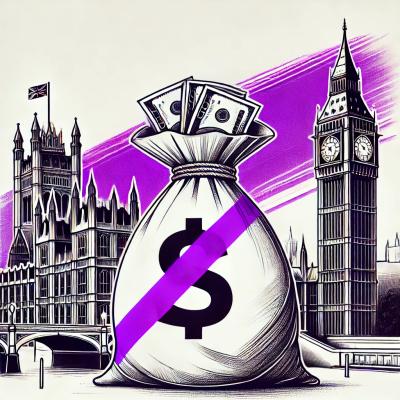
Security News
UK Officials Consider Banning Ransomware Payments from Public Entities
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
@bnaya/objectbuffer
Advanced tools
v0.10.0
.big refactor ongoing (allocator, hashmap)For Modern browsers and node. Zero direct dependencies.
Save, read and update plain javascript objects into ArrayBuffer
(And not only TypedArrays), using regular javascript object api, without serialization/deserialization.
Look at it as a simple implementation of javascript objects in user-land.
That's enables us to transfer
or share objects in-memory with WebWorker
without additional memory or serialization
The library is still not 1.0
, but already usable, and will never offer full compatibility with plain js (Symbol
and such)
For in-depth overview of how things are implemented, see implementation details document
import { createObjectBuffer, getUnderlyingArrayBuffer } from "@bnaya/objectbuffer";
const initialValue = {
foo: { bar: new Date(), arr: [1], nesting:{ WorksTM: true } }
};
// ArrayBuffer is created under the hood
const myObject = createObjectBuffer(
{
// available globally in the browser, or inside `util` in node
textEncoder: new TextEncoder(),
textDecoder: new TextDecoder()
},
// size in bytes
1024,
initialValue
);
const arrayBuffer = getUnderlyingArrayBuffer(myObject);
myObject.additionalProp = "new Value";
myObject.arr.push(2);
See also main.js for shared memory example.
to run it: clone the repo, yarn install
and yarn browser-playground
Exchanging plain objects with WebWorkers
is done by serializing and copying the data to the other side.
for some use-cases, it's slow and memory expensive.
ArrayBuffer
can be transferred
without a copy, and SharedArrayBuffer
can be directly shared, but out of the box, it's hard to use ArrayBuffer
as more than a TypedArray.
For many cases FlatBuffers is the right tool!
FlatBuffers requires full re-serialization when changing values. inside. The api is also more different than javascript objects.
I'm working on it mostly from personal interest, and i'm not using it for any project yet.
Before putting any eggs in the basket, please go over the implementation details document
foo.bar2 = foo.bar
will not create a copy)foo.bar === foo.bar
will be true)ArrayBuffer
. When exceed that size, exception will be thrown. (Can be extended with a utility function, but not automatically)bigint
bigger than 64 bitJSON.stringify
Symbol
There's a huge place for optimizations, code hygiene, and features!
Feel free to open issues and maybe implementing missing parts
FAQs
Object-like api, backed by an array buffer
The npm package @bnaya/objectbuffer receives a total of 17 weekly downloads. As such, @bnaya/objectbuffer popularity was classified as not popular.
We found that @bnaya/objectbuffer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.