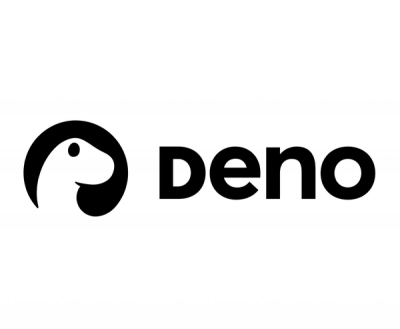
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@boilingdata/node-boilingdata
Advanced tools
You can use this SDK both on browser and with NodeJS.
See also BoilingData command line client tool: https://github.com/boilingdata/boilingdata-bdcli.
yarn add @boilingdata/node-boilingdata
execQueryPromise()
method can be used to await for the results directly.
import { BoilingData } from "@boilingdata/node-boilingdata";
async function main() {
const bdInstance = new BoilingData({ username: process.env["BD_USERNAME"], password: process.env["BD_PASSWORD"] });
await bdInstance.connect();
const sql = `SELECT COUNT(*) FROM parquet_scan('s3://boilingdata-demo/demo.parquet');`;
const rows = await bdInstance.execQueryPromise({ sql });
console.log(rows);
await bdInstance.close();
}
execQuery()
uses callbacks.
import { BoilingData, isDataResponse } from "@boilingdata/node-boilingdata";
async function main() {
const bdInstance = new BoilingData({ username: process.env["BD_USERNAME"], password: process.env["BD_PASSWORD"] });
await bdInstance.connect();
const sql = `SELECT COUNT(*) FROM parquet_scan('s3://boilingdata-demo/demo.parquet');`;
const rows = await new Promise<any[]>((resolve, reject) => {
let r: any[] = [];
bdInstance.execQuery({
sql,
callbacks: {
onData: (data: IBDDataResponse | unknown) => {
if (isDataResponse(data)) data.data.map(row => r.push(row));
},
onQueryFinished: () => resolve(r),
onLogError: (data: any) => reject(data),
},
});
});
console.log(rows);
await bdInstance.close();
}
This repository contains JS/TS BoilingData client SDK that can be used both with NodeJS and in browser. Please see the integration tests on tests/query.test.ts
for for more examples.
The SDK uses the BoilingData Websocket API in the background, meaning that events can arrive at any time. We use a range of global and query-specific callbacks to allow you to hook into the events that you care about.
All callbacks work in both the global scope and the query scope; i.e. global callbacks will always be executed when a message arrives, query callbacks will only be executed when messages relating to that query arrive.
Global callbacks can be set when creating the BoilingData instance.
new BoilingData({
username,
password,
globalCallbacks: {
onRequest: req => {
console.log("A new request has been made with ID", req.requestId);
},
onQueryFinished: req => {
console.log("Request complete!", req.requestId);
},
onLogError: message => {
console.error("LogError", message);
},
onSocketOpen: socketInstance => {
console.log("The socket has opened!");
},
onLambdaEvent: message => {
console.log("Change in status of dataset: ", message);
},
},
});
Query callbacks are set when creating the query
bdInstance.execQuery({
sql: `SELECT COUNT(*) AS count FROM parquet_scan('s3://boilingdata-demo/demo2.parquet');`,
callbacks: {
onData: data => {
console.log("Some data for this query arrived", data);
},
onQueryFinished: () => resolve(r),
onLogError: (data: any) => reject(data),
},
});
FAQs
BoilingData WebSocket client for Node and browser
The npm package @boilingdata/node-boilingdata receives a total of 29 weekly downloads. As such, @boilingdata/node-boilingdata popularity was classified as not popular.
We found that @boilingdata/node-boilingdata demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.