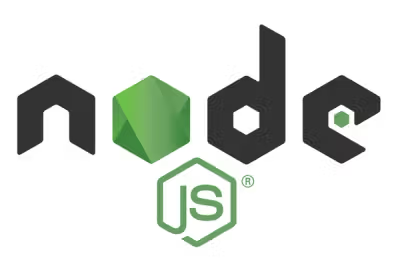
Security News
Node.js TSC Votes to Stop Distributing Corepack
Corepack will be phased out from future Node.js releases following a TSC vote.
@botmock/export
Advanced tools
@botmock/export
This package is used to export data into development platforms from Botmock projects.
The package is available to install on npm.
npm install @botmock/export
The following guides assumes a TypeScript environment with LTS Node.js installed.
Note: You can bootstrap such an environment by running
npx @botmock/cli
Note: Tokens and ids are created and accessed using the Botmock dashboard
@botmock/export
ships with a number of platform exporters, which generate file structures necessary for importing Botmock projects in popular development platforms.
Listed below are the named exports corresponding to development platforms currently supported by @botmock/export
.
BotFrameworkExporter
DialogflowExporter
LexExporter
LuisExporter
RasaExporter
SkillsKitExporter
WatsonExporter
Each can be accessed by through the following pattern.
import { BotFrameworkExporter } from "@botmock/export";
Let's walk through using the package's DialogflowExporter
.
We begin by adding an entry point to index.ts
.
// index.ts
import {
FileWriter,
Kind,
ProjectReference,
DialogflowExporter,
} from "@botmock/export";
const projectReference: ProjectReference = {
teamId: "YOUR_TEAM_ID",
projectId: "YOUR_PROJECT_ID",
boardId: "YOUR_BOARD_ID",
};
async function main(): Promise<void> {
const exporter = new DialogflowExporter({ token: "YOUR_TOKEN" });
}
main().catch((err: Error) => {
console.error(err);
});
The main
function creates a new instance of the DialogflowExporter
, and expects a valid token
.
If we add to this file, we can generate the file structure that the Dialogflow dashboard requires in order to restore a project.
// index.ts
async function main(): Promise<void> {
const exporter = new DialogflowExporter({ token: "YOUR_TOKEN" });
// The `data` property of the object returned by this method can be used by `FileWriter`, as we will see in the next step.
const { data } = await exporter.exportProjectUnderDataTransformations({ projectReference });
// Calling `writeAllResourcesToFiles` with `data` writes the files specificed in `data` to the filesystem.
const writeResult = await (new FileWriter({ directoryRoot: "./output" })).writeAllResourcesToFiles({ data });
if (writeResult.kind !== Kind.OK) {
console.error(writeResult.value);
} else {
console.log("done");
}
}
Running ts-node index.ts
should generate the .json
files that the Dialogflow dashboard requries to restore a project.
In the case that there is an error writing the files, an error value will be written to the console.
Let's walk through building a custom exporter by extending BaseExporter
in the package.
Extending the BaseExporter
is the approach taken by all platform exporters in the package.
We begin by adding an entry point to index.ts
.
// index.ts
const projectReference: ProjectReference = {
teamId: "YOUR_TEAM_ID",
projectId: "YOUR_PROJECT_ID",
boardId: "YOUR_BOARD_ID",
};
async function main() { }
main().catch(err => {
console.error(err);
});
Now, in a file named `exporter.ts
// exporter.ts
import {
BaseExporter,
Resources,
DataTransformation,
} from "@botmock/export";
export default class CustomExporter extends BaseExporter {
#rootTransformation = (resources: Resources): DataTransformation => {
return {
// Suppose our platform requires a file named `response-structure.json`
filename: "response-structure.json",
data: {},
}
};
dataTransformations = new Map([
["./path/to/output", this.#rootTransformation],
]);
}
exporter.ts
now exports CustomExporter
, which has dataTransformation
and #rootTransformation
.
dataTransformation
This public property must be a Map
. It is responsible for mapping relative output paths to functions that can turn Botmock project data into a specific object format.
#rootTransformation
This private field must return an object (or an array of objects) with the following structure.
{
// `filename` must be the name of a file with a `.json`, `.yml`, or `.md` extension.
filename: string;
// `data` can be an object that will later be mapped to `.json`, `.yml`, or `.md` file contents.
data: object;
}
Let's see how to make the #rootTransformation
do something useful.
The resources
parameter that is passed to this function is an object with the following properties.
Each of the following keys contains an object with payloads for each of the key names beloning to the project referenced in
index.ts
.
{
project,
team,
board,
intents,
entities,
variables,
}
In the transformation function itself, we have access to this entire resources
object, to say how the Botmock data should be transformed for the use of our platform.
Below, we assume our platform expects a nested object structure in response-structure.json
.
// exporter.ts
#rootTransformation = (resources: Resources): DataTransformation => {
const {
project,
board,
intents,
entities,
} = resources;
return {
filename: "response-structure.json",
data: {
name: resources.project.name,
responses: Object.values(board.board.messages).reduce((responses, response) => {
// Because the response block data is kept in a twice-nested object, the following code unpacks the underlying data to a flat array, for use in our platform.
const responseDataPerLocale = Object.values(response);
const responseDataPerPlatform = responseDataPerLocale.flatMap(data => Object.values(data).flatMap(value => value.blocks))
return {
...responses,
...responseDataPerPlatform,
}
}, {})
},
}
};
Jumping back to index.ts
, we can now do the following.
// index.ts
import {
FileWriter,
Kind,
ProjectReference,
} from "@botmock/export";
import { default as CustomExporter } from "./exporter.ts";
const projectReference: ProjectReference = {
teamId: "YOUR_TEAM_ID",
projectId: "YOUR_PROJECT_ID",
boardId: "YOUR_BOARD_ID",
};
async function main() {
const exporter = new CustomExporter({ token: "YOUR_TOKEN" });
// The `data` property of the object returned by this method can be used by `FileWriter`, as we will see in the next step.
const { data } = await exporter.exportProjectUnderDataTransformations({ projectReference });
}
main().catch(err => {
console.error(err);
});
Calling exporter.exportProjectUnderDataTransformations({ projectReference })
allows us to have access to the data
object, which can be used to write files to the local filesystem in a predictable way.
Let's see how FileWriter
works.
// index.ts
async function main() {
const exporter = new CustomExporter({ token: "YOUR_TOKEN" });
const { data } = await exporter.exportProjectUnderDataTransformations({ projectReference });
// Calling `writeAllResourcesToFiles` with `data` from the previous step writes the files specificed in `data` to the filesystem.
const writeResult = await (new FileWriter()).writeAllResourcesToFiles({ data });
// `writeResult` is an object with `kind` and `value` properties based on the success of the writes.
if (writeResult.kind !== Kind.OK) {
console.error(writeResult.value);
} else {
console.log("done");
}
}
Running ts-node index.ts
should generate the single response-structure.json
we outlined in our CustomExporter
.
BaseExporter
class
extended by platform exporters and custom exporters.
exportProjectUnderDataTransformations
Returns Promise
which resolves an object containing the data
property consumable by FileWriter
.
data
The data
property returned by exportProjectUnderDataTransformations
has the following shape.
Key name | Type | Description |
---|---|---|
[directoryPath] | array | an array of objects containing { filename: "file.json", data: {}, } , where data property in this object is a description of file contents that should belong in filename . |
This means that data
property can look like the following.
{
'./': [
{ filename: 'some-project.json', data: {} },
]
}
exportProjectUnderDataTransformations
takes a single options object as an argument.
The options object contains the following.
Key name | Type | Description |
---|---|---|
projectReference | object | object containing key, value pairs for "teamId", "projectId", and optionally, "boardId" |
dataTransformations
Required property on any BaseExporter
. This property should be a Map
that describes how Botmock project resources should be transformed for a given path.
import { BaseExporter, Resources, DataTransformation } from "@botmock/export";
class MyExporter extends BaseExporter {
#rootTransformation = (resources: Resources): DataTransformation => {
return {
filename: "file.json",
data: {
intents: resources.intents.map(intent => Object.values(intent.utterances)),
}
}
};
dataTransformations = new Map([
["../../some/relative/path", this.#rootTransformation],
]);
}
FileWriter
class
for parsing data
property generated by exportProjectUnderDataTransformations
and writing the files specified there to the correct locations in the local filesystem.
It can optionally take an options object, which has the following form.
Key name | Type | Description |
---|---|---|
directoryRoot | string | relative path to a path from which all paths in the dataTransformation should be joined |
jsonWriteOptions | object | object which is passed down to the fs-extra writeJSON method options |
import { FileWriter } from "@botmock/export";
import { MyExporter } from "./my-exporter";
(async () => {
const exporter = new MyExporter({ token: "YOUR_TOKEN" });
const { data } = await exporter.exportProjectUnderDataTransformations({ projectReference });
const writer = new FileWriter();
})();
writeAllResourcesToFiles
Returns a Promise
which resolves an object describing the success or failure of the file writing.
It takes a options argument with the following structure.
Key name | Type | Description |
---|---|---|
data | object | data property |
import { FileWriter } from "@botmock/export";
import { MyExporter } from "./my-exporter";
(async () => {
const exporter = new MyExporter({ token: "YOUR_TOKEN" });
const { data } = await exporter.exportProjectUnderDataTransformations({ projectReference });
const writer = new FileWriter();
const writeResult = await writer.writeAllResourcesToFiles({ data });
})();
FAQs
## Overview
The npm package @botmock/export receives a total of 232 weekly downloads. As such, @botmock/export popularity was classified as not popular.
We found that @botmock/export demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Corepack will be phased out from future Node.js releases following a TSC vote.
Research
Security News
Research uncovers Black Basta's plans to exploit package registries for ransomware delivery alongside evidence of similar attacks already targeting open source ecosystems.
Security News
Oxlint's beta release introduces 500+ built-in linting rules while delivering twice the speed of previous versions, with future support planned for custom plugins and improved IDE integration.