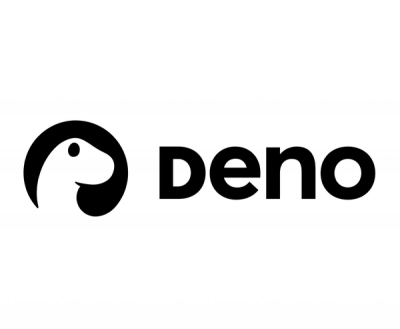
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@brighthustle/adonis-transmit
Advanced tools
This package makes it easy for developers to implement sse service in the AdonisJS 5 application.
Install the package using npm or yarn:
npm i @brighthustle/adonis-transmit
# or
yarn add @brighthustle/adonis-transmit
Then, configure the package using the configure command:
node ace configure @brighthustle/adonis-transmit
The module exposes a transmit
instance, which can be used to send events to the client.
import transmit from '@ioc:Adonis/Addons/Transmit'
// Anywhere in your code
transmit.broadcast('channelName', { username: 'lanz' })
Channels are a way to group events. For example, you can have a channel for users
and another for posts
. The client can subscribe to one or more channels to receive events.
Channels names must be a string and must not contain any special characters except /
. The following are valid channel names.
transmit.broadcast('users', { username: 'lanz' })
transmit.broadcast('users/1', { username: 'lanz' })
transmit.broadcast('users/1/posts', { username: 'lanz' })
You can mark a channel as private and then authorize the client to subscribe to it. The authorization is done using a callback function.
import type { HttpContextContract } from '@ioc:Adonis/Core/HttpContext'
transmit.authorizeChannel<{ id: string }>('users/:id', (ctx: HttpContextContract, { id }) => {
return ctx.auth.user?.id === +id
})
When a client tries to subscribe to a private channel, the callback function is invoked with the channel params and the HTTP context. The callback function must return a boolean value to allow or disallow the subscription.
Transmit uses Emittery to emit any lifecycle events. You can listen for events using the on
method.
transmit.on('connect', ({ uid }) => {
console.log(`Connected: ${uid}`)
})
transmit.on('disconnect', ({ uid }) => {
console.log(`Disconnected: ${uid}`)
})
transmit.on('broadcast', ({ channel }) => {
console.log(`Broadcasted to channel ${channel}`)
})
transmit.on('subscribe', ({ uid, channel }) => {
console.log(`Subscribed ${uid} to ${channel}`)
})
transmit.on('unsubscribe', ({ uid, channel }) => {
console.log(`Unsubscribed ${uid} from ${channel}`)
})
Please see the CHANGELOG for more information on what has changed recently.
The MIT License (MIT). Please see LICENSE file for more information.
This package is not officially maintained by Adonis. This page is migrated for Adonis v5 from original author plugin for Adonis v6, Inc.
FAQs
SSE Wrapper for adonis v5
We found that @brighthustle/adonis-transmit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.