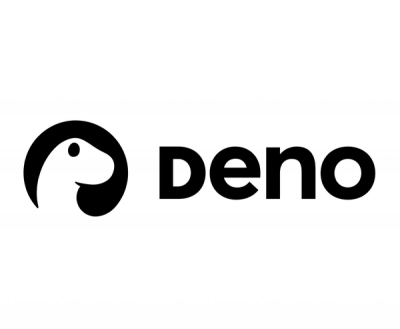
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@bruit/component
Advanced tools
BRuit is a User Issues Tool
Bruit.io is a WebComponents
Available on all frameworks that support WebComponents like
Bruit.io is simpliest tool (make on "web component" standard) for get your users feedbacks. Users feedbacks is sent directly to your favorite project management tools š .
Bruit.io gather an open source "webComponent" and a backend for format your feedback. and we do not do data retention about feedback š
Install
Usage
Configuration
Ā Ā Ā Ā BrtConfig
Ā Ā Ā Ā BrtField
Ā Ā Ā Ā BrtLabels
Ā Ā Ā Ā BrtColors
Ā Ā Ā Ā BrtLogLevels
Ā Ā Ā Ā BrtData
Ā Ā Ā Ā BrtError
Add data in feedback
Handle errors
Framework integrations
Contributing
Having troubles ?
npm install @bruit/component --save
Or
<script src="https://unpkg.com/@bruit/component/dist/bruit.js"></script>
<bruit-io> element to click </bruit-io>
with properties :
Framework integrations :
bruit-io
webComponent has aconfig
property.
config
property take a BrtConfig value.
BrtConfig is a JSON for configure and customize bruit-io
component
Attribute | Type | Description | Required | Default value |
---|---|---|---|---|
apiKey | string | your personal api key (create my api key) | yes | - |
form | array<BrtField> | input list for generate form | yes | - |
closeModalOnSubmit | boolean | true for close modal directly on submit form and send feedback in background | no | false |
labels | BrtLabels | labels of the modal (title/button/...) | no | see |
logLevels | BrtLogLevels | type and number of log to send | no | see |
maxLogLines | number | number of log to send | no | 100 |
colors | BrtColors | modal theming | no | see |
apiUrl | string | if you want use your own api for send feedback | no | https://api.bruit.io/feedback |
import { BrtConfig } from '@bruit/component';
BrtConfig.form
must be contains minimum one BrtField
with id="agreement"
and type="checkbox"
.
This field is used to check if the user agrees to send his personal data.
Special ids :
agreement
id is used for determinate the field to use for check if user agrees to send his personal datatitle
id is used for determinate the field tu use for make the title of feedbackFormat :
interface BrtField {
id?: string;
label: string;
type: string;
required?: boolean;
value?: any;
}
import { BrtField } from '@bruit/component';
This values is used in modal only, the title is show in the header, introduction under the header, and button in submit button.
interface BrtLabels {
title?: string;
introduction?: string;
button?: string;
}
{
"title": "bruit.io",
"introduction": "send a feedback",
"button": "send"
}
import { BrtLabels } from '@bruit/component';
šØ If you are an artist, use BrtColors for the modal theming.
It's possible to change the header, body, background, errors and focus colors.
Use hexadecimal values only.
interface BrtColors {
header?: string;
body?: string;
background?: string;
errors?: string;
focus?: string;
}
{
"header": "#2D8297",
"body": "#eee",
"background": "#444444ee",
"errors": "#c31313",
"focus": "#1f5a69"
}
import { BrtColors } from '@bruit/component';
By default, all your log types (log, warn, errors, ...) are send in feedback. BrtLogLevels
allows to disable specific type.
For disable a type, just set logLevels with this type to false :
{
"apiKey": "xxxxxxxxxx",
"form": ["..."],
"logLevels": {
"log": false
}
}
special types:
network
type is for your fetch and xmlHttpRequest calls.click
type is for mouse click event.url
type log all url changingFormat :
interface BrtLogLevels {
log?: boolean;
debug?: boolean;
info?: boolean;
warn?: boolean;
error?: boolean;
network?: boolean;
click?: boolean;
url?: boolean;
}
{
"log": true,
"debug": true,
"info": true,
"warn": true,
"error": true,
"network": true,
"click": true,
"url": true
}
import { BrtLogLevels } from '@bruit/component';
You can add data in feedback (example: a version number, a user id, ...).
If you have data that can be retrieved synchronously, you can use data
.
For asynchronous data, you can use dataFn
.
data
property is used for send synchronous data.
Just give him an BrtData
array.
dataFn
property is used for send asynchronous data.
Give him an Promise
of BrtData
array. Your promise will be called upon to submit the feedback.
interface BrtData {
label: string;
type?: string;
value: any;
id?: string;
}
import { BrtData } from '@bruit/component';
bruit-io
emit onError
event when an error occured.
An error is composed by a code
and a text
. it is of type BrtError
.
interface BrtError {
code: number;
text: string;
}
import { BrtError } from '@bruit/component';
Integrating bruit-io
component to a project without a JavaScript framework is straight forward. If you're using a simple HTML page, you can add bruit component via a script tag.
<!DOCTYPE html>
<html lang="en">
<head>
<script src="https://unpkg.com/@bruit/component/dist/test-components.js"></script>
</head>
<body>
<bruit-io></bruit-io>
<script>
var bruitCmp = document.querySelector('bruit-io');
bruitCmp.config = {
apiKey: 'xxxxxxxxxxxxxxxxx',
form: [...]
};
</script>
</body>
</html>
Using bruit-io
component within an Angular CLI project is a two-step process. We need to:
CUSTOM_ELEMENTS_SCHEMA
in the modules that use the componentsdefineCustomElements(window)
from main.ts
(or some other appropriate place)Including the CUSTOM_ELEMENTS_SCHEMA
in the module allows the use of the web components in the HTML markup without the compiler producing errors. Here is an example of adding it to AppModule
:
import { BrowserModule } from '@angular/platform-browser';
import { CUSTOM_ELEMENTS_SCHEMA, NgModule } from '@angular/core';
import { AppComponent } from './app.component';
@NgModule({
declarations: [AppComponent],
imports: [BrowserModule],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA]
})
export class AppModule {}
The CUSTOM_ELEMENTS_SCHEMA
needs to be included in any module that uses custom elements.
Bruit component include a main function that is used to load the components in the collection. That function is called defineCustomElements()
and it needs to be called once during the bootstrapping of your application. One convenient place to do this is in main.ts
as such:
import { enableProdMode } from '@angular/core';
import { platformBrowserDynamic } from '@angular/platform-browser-dynamic';
import { AppModule } from './app/app.module';
import { environment } from './environments/environment';
import { defineCustomElements } from '@bruit/component/dist/loader';
if (environment.production) {
enableProdMode();
}
platformBrowserDynamic()
.bootstrapModule(AppModule)
.catch(err => console.log(err));
defineCustomElements(window);
<bruit-io [config]="bruitConfig" [data]="bruitData" [dataFn]="bruitDataPromise()" (onError)="handleBruitError($event)"></bruit-io>
public bruitConfig: BrtConfig = {
apiKey:"xxxxxxxxxxx",
form:[...]
};
public bruitData: Array<BrtData> = [
{
label:"version",
value: environment.version
}
];
constructor(private api : ApiService){}
bruitDataPromise():Promise<Array<BrtData>>{
return this.api.getUser().then( user =>
[
{
label: "user id",
value: user.id
},
{
label: "user email",
value: user.email
}
]
);
}
handleBruitError(error: BrtError){
...
}
With an application built using the create-react-app
script the easiest way to include the bruit-io
component is to call defineCustomElements(window)
from the index.js
file.
import React from 'react';
import ReactDOM from 'react-dom';
import './index.css';
import App from './App';
import registerServiceWorker from './registerServiceWorker';
import { defineCustomElements } from '@bruit/component/dist/loader';
ReactDOM.render(<App />, document.getElementById('root'));
registerServiceWorker();
defineCustomElements(window);
In order to use the bruit-io
component within the Vue app, the application must be modified to define the custom elements and to inform the Vue compiler which elements to ignore during compilation. This can all be done within the main.js
file. For example:
import Vue from 'vue';
import App from './App.vue';
import { defineCustomElements } from '@bruit/component/dist/loader';
Vue.config.productionTip = false;
Vue.config.ignoredElements = [/bruit-\w*/];
defineCustomElements(window);
new Vue({
render: h => h(App)
}).$mount('#app');
xxx
FAQs
send your feedbacks with bruit.io
The npm package @bruit/component receives a total of 187 weekly downloads. As such, @bruit/component popularity was classified as not popular.
We found that @bruit/component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Ā It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.