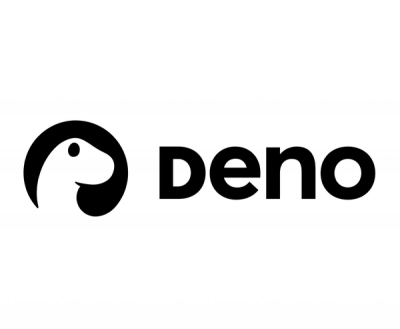
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@bucketco/node-sdk
Advanced tools
Node.js, JavaScriptS/Typescript feature flag and tracking client for [Bucket.co](https://bucket.co).
Node.js, JavaScriptS/Typescript feature flag and tracking client for Bucket.co.
Install using yarn
or npm
with:
yarn add -s @bucketco/node-sdk
ornpm install -s @bucketco/node-sdk
.
Other supported languages/frameworks are in the Supported languages documentation pages.
You can also use the HTTP API directly
To get started you need to obtain your secret key from the environment settings in Bucket.
{% hint style="danger" %} Secret keys are meant for use in server side SDKs only. Secret keys offer the users the ability to obtain information that is often sensitive and thus should not be used in client-side applications. {% endhint %}
Bucket will load settings through the various environment variables automatically (see Configuring below).
BUCKET_SECRET_KEY
in your .env
filebucket.ts
file containing the following:import { BucketClient } from "@bucketco/node-sdk";
// Create a new instance of the client with the secret key. Additional options
// are available, such as supplying a logger and other custom properties.
//
// We recommend that only one global instance of `client` should be created
// to avoid multiple round-trips to our servers.
export const bucketClient = new BucketClient();
// Initialize the client and begin fetching feature targeting definitions.
// You must call this method prior to any calls to `getFeatures()`,
// otherwise an empty object will be returned.
bucketClient.initialize().then({
console.log("Bucket initialized!")
})
Once the client is initialized, you can obtain features along with the isEnabled
status to indicate whether the feature is targeted for this user/company:
Note: If user.id
or company.id
is not given, the whole user
or company
object is ignored.
// configure the client
const boundClient = bucketClient.bindClient({
user: {
id: "john_doe",
name: "John Doe",
email: "john@acme.com",
avatar: "https://example.com/users/jdoe",
},
company: {
id: "acme_inc",
name: "Acme, Inc.",
avatar: "https://example.com/companies/acme",
},
});
// get the huddle feature using company, user and custom context to
// evaluate the targeting.
const { isEnabled, track } = boundClient.getFeature("huddle");
if (isEnabled) {
// this is your feature gated code ...
// send an event when the feature is used:
track();
// CAUTION: if you plan to use the event for automated feedback surveys
// call `flush` immediately after `track`. It can optionally be awaited
// to guarantee the sent happened.
boundClient.flush();
}
You can also use the getFeatures()
method which returns a map of all features:
// get the current features (uses company, user and custom context to
// evaluate the features).
const features = boundClient.getFeatures();
const bothEnabled =
features.huddle?.isEnabled && features.voiceHuddle?.isEnabled;
The Bucket Node SDK contacts the Bucket servers when you call initialize()
and downloads the features with their targeting rules.
These rules are then matched against the user/company information you provide
to getFeatures()
(or through bindClient(..).getFeatures()
). That means the
getFeatures()
call does not need to contact the Bucket servers once
initialize()
has completed. BucketClient
will continue to periodically
download the targeting rules from the Bucket servers in the background.
The Bucket Node.js
SDK can be configured through environment variables,
a configuration file on disk or by passing options to the BucketClient
constructor. By default, the SDK searches for bucketConfig.json
in the
current working directory.
Option | Type | Description | Env Var |
---|---|---|---|
secretKey | string | The secret key used for authentication with Bucket's servers. | BUCKET_SECRET_KEY |
logLevel | string | The log level for the SDK (e.g., "DEBUG" , "INFO" , "WARN" , "ERROR" ). Default: INFO | BUCKET_LOG_LEVEL |
offline | boolean | Operate in offline mode. Default: false , except in tests it will default to true based off of the TEST env. var. | BUCKET_OFFLINE |
apiBaseUrl | string | The base API URL for the Bucket servers. | BUCKET_API_BASE_URL |
featureOverrides | Record<string, boolean> | An object specifying feature overrides for testing or local development. See example/app.test.ts for how to use featureOverrides in tests. | BUCKET_FEATURES_ENABLED, BUCKET_FEATURES_DISABLED |
configFile | string | Load this config file from disk. Default: bucketConfig.json | BUCKET_CONFIG_FILE |
Note: BUCKET_FEATURES_ENABLED, BUCKET_FEATURES_DISABLED are comma separated lists of features which will be enabled or disabled respectively.
bucketConfig.json
example:
{
"secretKey": "...",
"logLevel": "warn",
"offline": true,
"apiBaseUrl": "https://proxy.slick-demo.com",
"featureOverrides": {
"huddles": true,
"voiceChat": false
}
}
When using a bucketConfig.json
for local development, make sure you add it to your
.gitignore
file. You can also set these options directly in the BucketClient
constructor. The precedence for configuration options is as follows, listed in the
order of importance:
To get type checked feature flags, add the list of flags to your bucket.ts
file.
Any feature look ups will now be checked against the list you maintain.
import { BucketClient } from "@bucketco/node-sdk";
// Extending the Features interface to define the available features
declare module "@bucketco/node-sdk" {
interface Features {
"show-todos": boolean;
"create-todos": boolean;
"delete-todos": boolean;
}
}
export const bucketClient = new BucketClient();
bucketClient.initialize().then({
console.log("Bucket initialized!")
bucketClient.getFeature("invalid-feature") // feature doesn't exist
})
A popular way to integrate the Bucket Node.js SDK is through an express middleware.
import bucket from "./bucket";
import express from "express";
import { BoundBucketClient } from "@bucketco/node-sdk";
// Augment the Express types to include a `boundBucketClient` property on the
// `res.locals` object.
// This will allow us to access the BucketClient instance in our route handlers
// without having to pass it around manually
declare global {
namespace Express {
interface Locals {
boundBucketClient: BoundBucketClient;
}
}
}
// Add express middleware
app.use((req, res, next) => {
// Extract the user and company IDs from the request
// You'll want to use a proper authentication and identification
// mechanism in a real-world application
const user = {
id: req.user?.id,
name: req.user?.name
email: req.user?.email
}
const company = {
id: req.user?.companyId
name: req.user?.companyName
}
// Create a new BoundBucketClient instance by calling the `bindClient`
// method on a `BucketClient` instance
// This will create a new instance that is bound to the user/company given.
const boundBucketClient = bucket.bindClient({ user, company });
// Store the BoundBucketClient instance in the `res.locals` object so we
// can access it in our route handlers
res.locals.boundBucketClient = boundBucketClient;
next();
});
// Now use res.locals.boundBucketClient in your handlers
app.get("/todos", async (_req, res) => {
const { track, isEnabled } = res.locals.bucketUser.getFeature("show-todos");
if (!isEnabled) {
res.status(403).send({"error": "feature inaccessible"})
return
}
...
}
See example/app.ts for a full example.
If you don't want to provide context each time when evaluating feature flags but
rather you would like to utilize the attributes you sent to Bucket previously
(by calling updateCompany
and updateUser
) you can do so by calling getFeaturesRemote
(or getFeatureRemote
for a specific feature) with providing just userId
and companyId
.
These methods will call Bucket's servers and feature flags will be evaluated remotely
using the stored attributes.
// Update user and company attributes
client.updateUser("john_doe", {
attributes: {
name: "John O.",
role: "admin",
},
});
client.updateCompany("acme_inc", {
attributes: {
name: "Acme, Inc",
tier: "premium"
},
});
...
// This will evaluate feature flags with respecting the attributes sent previously
const features = await client.getFeaturesRemote("acme_inc", "john_doe");
NOTE: User and company attribute updates are processed asynchronously, so there might be a small delay between when attributes are updated and when they are available for evaluation.
There are use cases in which you not want to be sending user
, company
and
track
events to Bucket.co. These are usually cases where you could be impersonating
another user in the system and do not want to interfere with the data being
collected by Bucket.
To disable tracking, bind the client using bindClient()
as follows:
// binds the client to a given user and company and set `enableTracking` to `false`.
const boundClient = client.bindClient({ user, company, enableTracking: false });
boundClient.track("some event"); // this will not actually send the event to Bucket.
// the following code will not update the `user` nor `company` in Bucket and will
// not send `track` events either.
const { isEnabled, track } = boundClient.getFeature("user-menu");
if (isEnabled) {
track();
}
Another way way to disable tracking without employing a bound client is to call getFeature()
or getFeatures()
by supplying enableTracking: false
in the arguments passed to
these functions.
[!NOTE] Note, however, that calling
track()
,updateCompany()
orupdateUser()
in theBucketClient
will still send tracking data. As such, it is always recommended to usebindClient()
when using this SDK.
It is highly recommended that users of this SDK manually call flush()
method on process shutdown. The SDK employs a batching technique to minimize
the number of calls that are sent to Bucket's servers. During process shutdown,
some messages could be waiting to be sent, and thus, would be discarded if the
buffer is not flushed.
By default, the SDK automatically subscribes to process exit signals and attempts to flush
any pending events. This behavior is controlled by the flushOnExit
option in the client configuration:
const client = new BucketClient({
batchOptions: {
flushOnExit: false, // disable automatic flushing on exit
},
});
[!NOTE] If you are creating multiple client instances in your application, it's recommended to disable
flushOnExit
to avoid potential conflicts during process shutdown. In such cases, you should implement your own flush handling.
When you bind a client to a user/company, this data is matched against the targeting rules. To get accurate targeting, you must ensure that the user/company information provided is sufficient to match against the targeting rules you've created. The user/company data is automatically transferred to Bucket. This ensures that you'll have up-to-date information about companies and users and accurate targeting information available in Bucket at all time.
Tracking allows events and updating user/company attributes in Bucket. For example, if a customer changes their plan, you'll want Bucket to know about it, in order to continue to provide up-do-date targeting information in the Bucket interface.
The following example shows how to register a new user, associate it with a company and finally update the plan they are on.
// registers the user with Bucket using the provided unique ID, and
// providing a set of custom attributes (can be anything)
client.updateUser("user_id", {
attributes: { longTimeUser: true, payingCustomer: false },
});
client.updateCompany("company_id", { userId: "user_id" });
// the user started a voice huddle
client.track("user_id", "huddle", { attributes: { voice: true } });
It's also possible to achieve the same through a bound client in the following manner:
const boundClient = client.bindClient({
user: { id: "user_id", longTimeUser: true, payingCustomer: false },
company: { id: "company_id" },
});
boundClient.track("huddle", { attributes: { voice: true } });
Some attributes are used by Bucket to improve the UI, and are recommended to provide for easier navigation:
name
-- display name for user
/company
,email
-- the email of the user.avatar
-- the URL for user
/company
avatar image.Attributes cannot be nested (multiple levels) and must be either strings, integers or booleans.
Last seen
By default updateUser
/updateCompany
calls automatically update the given
user/company Last seen
property on Bucket servers.
You can control if Last seen
should be updated when the events are sent by setting
meta.active = false
. This is often useful if you
have a background job that goes through a set of companies just to update their
attributes but not their activity.
Example:
client.updateUser("john_doe", {
attributes: { name: "John O." },
meta: { active: true },
});
client.updateCompany("acme_inc", {
attributes: { name: "Acme, Inc" },
meta: { active: false },
});
bindClient()
updates attributes on the Bucket servers but does not automatically
update Last seen
.
The Bucket SDK doesn't collect any metadata and HTTP IP addresses are not being stored. For tracking individual users, we recommend using something like database ID as userId, as it's unique and doesn't include any PII (personal identifiable information). If, however, you're using e.g. email address as userId, but prefer not to send any PII to Bucket, you can hash the sensitive data before sending it to Bucket:
import { sha256 } from 'crypto-hash';
client.updateUser({ userId: await sha256("john_doe"), ... });
Types are bundled together with the library and exposed automatically when importing through a package manager.
MIT License Copyright (c) 2025 Bucket ApS
FAQs
Node.js, JavaScriptS/Typescript feature flag and tracking client for [Bucket.co](https://bucket.co).
The npm package @bucketco/node-sdk receives a total of 2,755 weekly downloads. As such, @bucketco/node-sdk popularity was classified as popular.
We found that @bucketco/node-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.