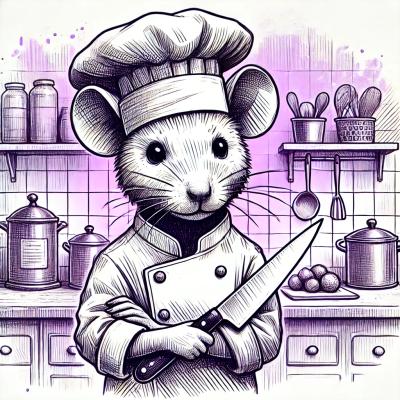
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@burstjs/monitor
Advanced tools
A monitor to watch for specific changes on the Burst blockchain
Due to average blocktime of 240 seconds, transactions stay pending for a certain time. It is a repeating pattern to watch for such changes and waiting for confirmation. This package simplifies this task.
As additional feature, a monitor is serializable, that means it can be stored e restored. This is especially useful for web applications, as it allows reloading pages without losing the ability to check whether a transaction is still pending or already concluded.
@burstjs/monitor
can be used with NodeJS or Web. Two formats are available
Install using npm:
npm install @burstjs/monitor
or using yarn:
yarn add @burstjs/monitor
import {Monitor} from '@burstjs/monitor'
import {composeApi} from "@burstjs/core";
// A method that checks if an account exists
// > IMPORTANT: Do not use closures, when you need to serialize the monitor
async function tryFetchAccount() {
const BurstApi = composeApi({ nodeHost: 'https://testnet.burstcoin.network:6876/'})
try{
const {account} = await BurstApi.account.getAccount('1234')
return account;
}catch (e){
// ignore error
return null;
}
}
// A comparing function to check if a certain condition for the returned data from fetch function
// is true. If it's true the monitor stops
function checkIfAccountExists(account) {
return account !== null;
}
// Create your monitor
const monitor = new Monitor({
asyncFetcherFn: tryFetchAccount,
compareFn: checkIfAccountExists,
intervalSecs: 10, // polling interval in seconds
key: 'monitor-account',
timeoutSecs: 2 * 240 // when reached timeout the monitor stops
});
// starts monitor
monitor.start();
// called when `checkIfAccountExists` returns true
monitor.onFulfilled(() => {
console.log('Yay, account active');
});
// called when `timeoutSecs` is reached
monitor.onTimeout(() => {
console.log('Hmm, something went wrong');
});
<script>
Each package is available as bundled standalone library using IIFE.
This way burstJS can be used also within <script>
-Tags.
This might be useful for Wordpress and/or other PHP applications.
Just import the package using the HTML <script>
tag.
<script src='https://cdn.jsdelivr.net/npm/@burstjs/monitor/dist/burstjs.monitor.min.js'></script>
const monitor = new b$monitor.Monitor({
//...
});
monitor.start()
monitor.onFulFilled(() => {
//...
})
TO DO
See more here:
@burstjs/monitor Online Documentation
The generic monitor class.
A monitor can be used to check periodically for a certain situation, e.g. confirmation of a transaction, activation on an account, or even something completely different.
Example: (checking for the existence of an account aka account activation)
// A method that checks if an account exists // > IMPORTANT: Do not use closures, when you need to serialize the monitor async function tryFetchAccount() { const BurstApi = composeApi({ nodeHost: 'https://testnet.burstcoin.network:6876/'}) try{ const {account} = await BurstApi.account.getAccount('1234') return account; }catch (e){ // ignore error return null; } }
// A comparing function to check if a certain condition for the returned data from fetch function // is true. If it's true the monitor stops function checkIfAccountExists(account) { return account !== null; }
// Create your monitor const monitor = new Monitor<Account>({ asyncFetcherFn: tryFetchAccount, compareFn: checkIfAccountExists, intervalSecs: 10, // polling interval in seconds key: 'monitor-account', timeoutSecs: 2 * 240 // when reached timeout the monitor stops }) .onFulfilled(() => { // called when
checkIfAccountExists
returns true console.log('Yay, account active'); }) .onTimeout(() => { // called whentimeoutSecs
is reached console.log('Hmm, something went wrong'); }).start();
Kind: inner class of monitor
The monitors constructor
Param | Description |
---|---|
args | The arguments |
The start timestamp if started, or -1
Kind: instance property of Monitor
The interval
Kind: instance property of Monitor
The key aka identifier
Kind: instance property of Monitor
The timeout
Kind: instance property of Monitor
Serializes the monitor, such it can be stored.
This serializes also the asyncFetcher
and compareFn
It is important that these functions are not closures, i.e. the must not reference
outer data/variables, otherwise the behavior on deserialization is not deterministic
Kind: instance method of Monitor
Kind: instance method of Monitor
Returns:
true, if monitor was started and is running
Kind: instance method of Monitor
Returns:
true, if a started monitor timed out.
Starts the monitor
Kind: instance method of Monitor
Stops the monitor
Kind: instance method of Monitor
Callback function for timeout event. You can add multiple event listener if you want
Kind: instance method of Monitor
Param | Description |
---|---|
fn | The callback |
Callback function for fulfilled event. You can add multiple event listener if you want
Kind: instance method of Monitor
Param | Description |
---|---|
fn | The callback |
Deserializes a serialized monitor
Kind: static method of Monitor
Returns:
The monitor instance
[[Monitor.serialize]]
Param | Default | Description |
---|---|---|
serializedMonitor | The serialized monitor | |
autoStart | true | If monitor was started on serialization the monitor starts automatically, if set true (default) |
FAQs
Monitor transactions on Burst blockchain
The npm package @burstjs/monitor receives a total of 0 weekly downloads. As such, @burstjs/monitor popularity was classified as not popular.
We found that @burstjs/monitor demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.