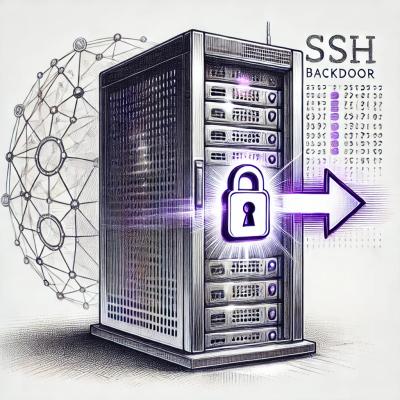
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@byteark/byteark-player-vue
Advanced tools
Table of Contents
You can try on the demo page.
Since Vue 3 is not backward compatible, please make sure to install the correct ByteArk Player Container version based on your Vue version.
Vue Version | Package Version | Branch |
---|---|---|
2.x | 3.x | master |
3.x | 4.x | main |
This library is distributed via NPM. You may install from NPM, Yarn, PNPM.
# For NPM
npm install --save @byteark/byteark-player-vue
# For Yarn
yarn add @byteark/byteark-player-vue
# For PNPM
pnpm add @byteark/byteark-player-vue
ByteArkPlayerContainer
in your project.<template>
<ByteArkPlayerContainer
:options="playerOptions"
/>
</template>
<script setup lang="ts">
import ByteArkPlayerContainer from '@byteark/byteark-player-vue';
import type { ByteArkPlayerOptions } from '@byteark/byteark-player-vue';
const playerOptions: ByteArkPlayerOptions = {
autoplay: 'any',
fluid: true,
aspectRatio: '16:9',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
sources: [{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/TZyZheqEJUwC/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'TZyZheqEJUwC',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
}]
};
</script>
// Inside your main SCSS file
@import '~@byteark/byteark-player-vue/dist/@byteark/byteark-player-vue.css';
// Or inside the component
<style lang="scss" scoped>
@import '~@byteark/byteark-player-vue/dist/@byteark/byteark-player-vue.css';
</style>
In case that you want to display videos inside a fix-sized container, use fill layout mode by passing fill: true
in the player options.
playerOptions: {
...options,
fill: true,
},
Following properties are the properties that can be updated to the ByteArkPlayerContainer.
Name | Type | Default | Description |
---|---|---|---|
options | ByteArkPlayerOptions | - | An options pass to ByteArk Player (See ByteArk Player Options Object) |
customClass | String | - | Add custom css class to ByteArkPlayerContainer component |
lazyload | Boolean | - | The player loads its asset files once it got rendered on the webpage. By passing this prop, the player then loads its asset files once the user clicked on the player instead. |
playerEndpoint | String | - | Endpoint to the video player (without version part). |
playerSlugId | String | - | SlugId of player created via api player server service |
playerVersion | String | v2 | Custom version of the player. |
playerJsFileName | String | File name of player's JS. | |
playerCssFileName | String | File name of player's CSS. | |
setupPlayerFunction | (options: ByteArkPlayerOptions, loadScriptOrStyleFunction: (id: string, url: string, type: 'script' | 'style') => Promise | - | This function call to before create player function |
createPlayerFunction | (node: HTMLMediaElement, options: ByteArkPlayerOptions, onReady: () => void) => ByteArkPlayer | - | This function should return a VideoJS's player instance. |
onPlayerLoaded | () => void | - | Callback function to be called when loaded player's resources. |
onPlayerLoadError | (error: ByteArkPlayerContainerError, originalError: ByteArkPlayerError | unknown) => void | - | Callback function to be called when there're an error about loading player. |
onPlayerSetup | () => void | - | Callback function to be called when player is setup. |
onPlayerSetupError | (error: ByteArkPlayerContainerError, originalError: ByteArkPlayerError | unknown) => void | - | Callback function to be called when there're an error when setup player. |
onPlayerCreated | (player: ByteArkPlayer) => void | - | Callback function to be called when a player instance is created. |
onPlayerReady | (player: ByteArkPlayer) => void | - | Callback function to be called when a player instance is ready. |
You have to pass options
object to ByteArkPlayerContainer
in order to create ByteArk Player instance.
Name | Type | Default | Description |
---|---|---|---|
autoplay | Boolean/String | - | Autoplay the video after the player is created. (Seee VideoJS's autoplay options) |
aspectRatio | String | - | Use with fluid layout mode, to inform expected video's aspect ratio |
controls | Boolean | true | Show the controls bar. |
fill | Boolean | - | Use fill layout mode. |
fluid | Boolean | - | Use fluid layout mode. |
loop | Boolean | - | Replay the video after ending |
muted | Boolean | - | Play the video without sounds. |
playbackRate | Number | 1.0 | Playback speed. 1.0 means original speed. |
playsinline | Boolean | true | Should be true so custom controls available on all platforms, including iOS. |
poster | String | - | Image to show before playing the video. |
preload | String | - | Preload the video before playing. (none / metadata / auto) |
responsive | Boolean | - | Auto show/hide controls depending on the screen size. |
seekButtons | Boolean | - | Show 10 seconds seek buttons and allow double-tap to seek on mobile. |
sources | Array | - | Array of video source object to be played. (See Source Object) |
volume | Number | - | Video's volume between 0 and 1. |
plugins | Array | - | Videojs's plugins |
You can also use other props not listed here, but appear as VideoJS's options. However, changing props will not effective after the player is created.
The sources
object has 2 required fields.
Name | Type | Required | Description |
---|---|---|---|
src | String | true | URL to the video. |
type | String | true | Video content type. |
title | String | false | Video title to display on the player. |
videoId | String | false | Video's ID, usually used by analytical applications. |
poster | String | false | Image to show before playing the video. |
To provide multiple version of sources, you can use array of source objects.
ByteArkPlayerContainer emits events that you can use to trigger your custom functions.
Event Name | Callback Parameters | Trigger Condition |
---|---|---|
loaded | - | When loaded player's resources. |
loaderror | (error: ByteArkPlayerContainerError, originalError: ByteArkPlayerError | unknown): void | When there're an error about loading player's resources. |
setup | - | When player is setup. |
setuperror | (error: ByteArkPlayerContainerError, originalError: ByteArkPlayerError | unknown): void | When there're an error when setup player. |
created | (player: ByteArkPlayer) | When the player instance was created. |
ready | (player: ByteArkPlayer) | When the player instance was ready to play. |
firstplay | (player: ByteArkPlayer) | When the video played for the first time. |
play | (player: ByteArkPlayer, currentTime: number) | When the video played or the user hit play. (Value of currentTime is a number in seconds) |
pause | (player: ByteArkPlayer, currentTime: number) | When the video played or the user hit pause. (Value of currentTime is a number in seconds) |
ended | (player: ByteArkPlayer) | When the video ended. |
timeupdate | (player: ByteArkPlayer, currentTime: number) | When the current playback time changed. (Value of currentTime is a number in seconds) |
seeking | (player: ByteArkPlayer, currentTime: number) | When the the user seeked the video. (Value of currentTime is a number in seconds) |
seeked | (player: ByteArkPlayer, currentTime: number) | When the the user seeked the video. (Value of currentTime is a number in seconds) |
waiting | (player: ByteArkPlayer) | When the player is waiting for the video. |
stalled | (player: ByteArkPlayer) | When the player is waiting for the video. |
fullscreenchange | (player: ByteArkPlayer, isFullscreen: boolean) | When the user entered or exited the full screen mode. (Value of isFullscreen is True or False) |
volumechange | (player: ByteArkPlayer, volume: number) | When the user adjusted the volume. (Value of volume is between 0 - 1) |
ratechange | (player: ByteArkPlayer, playbackSpeed: number) | When the user adjusted the playback speed. (Value of playbackSpeed is a number) |
enterpictureinpicture | (player: ByteArkPlayer) | When the user entered Picture-in-Picture mode. |
leavepictureinpicture | (player: ByteArkPlayer) | When the user exited Picture-in-Picture mode. |
error | (error: ByteArkPlayerError | MediaError | unknown | null) | When there was an error while loading player. |
For an example of implementing these events, please see Controlling Players Section.
You may access the player instance from onReady callback parameter.
<template>
<ByteArkPlayerContainer
@ready="onReady"
@firstplay="onFirstPlay"
@play="onPlay"
@pause="onPause"
@ended="onVideoEnded"
@timeupdate="onTimeUpdated"
@seeking="onVideoSeeking"
@seeked="onVideoSeeked"
@fullscreenchange="onToggleFullScreen"
@volumechange="onVolumeChange"
@ratechange="onPlaybackSpeedChanged"
@enterpictureinpicture="onPIPEntered"
@leavepictureinpicture="onPIPExited"
:options="playerOptions" />
<button @click="player.value?.play()">Play</button>
<button @click="player.value?.pause()">Pause</button>
</template>
<script setup lang="ts">
import { ref } from 'vue'
import ByteArkPlayerContainer from '@byteark/byteark-player-vue'
import type { ByteArkPlayerOptions, ByteArkPlayer } from '@byteark/byteark-player-vue'
const playerRef = ref<ByteArkPlayer | null>(null)
const playerOptions: ByteArkPlayerOptions = {
autoplay: 'any',
fluid: true,
aspectRatio: '16:9',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
sources: [{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/TZyZheqEJUwC/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'TZyZheqEJUwC',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
}]
}
const onReady = (player: ByteArkPlayer) => {
// The player is ready! save player instance
playerRef.value = player
}
const onFirstPlay = (player: ByteArkPlayer) => {
// Do something...
}
const onPlay = (player: ByteArkPlayer, currentTime: number) => {
// Do something...
}
const onPause = (player: ByteArkPlayer, currentTime: number) => {
// Do something...
}
const onVideoEnded = () => {
// Do something...
}
const onTimeUpdated = (player: ByteArkPlayer, currentTime: number) => {
// Do something...
}
const onVideoSeeking = (player: ByteArkPlayer, currentTime: number) => {
// Do something...
}
const onVideoSeeked = (player: ByteArkPlayer, currentTime: number) => {
// Do something...
}
const onToggleFullScreen = (player: ByteArkPlayer, isFullscreen: boolean) => {
// Do something...
}
const onVolumeChange = (player: ByteArkPlayer, volume: number) => {
// Do something...
}
const onPlaybackSpeedChanged = (player: ByteArkPlayer, playbackSpeed: number) => {
// Do something...
}
const onPIPEntered = (player: ByteArkPlayer,) => {
// Do something...
}
const onPIPExited = (player: ByteArkPlayer,) => {
// Do something...
}
</script>
<template>
<ByteArkPlayerContainer
@ready="onReady"
:options="playerOptions"
/>
</template>
<script setup lang="ts">
import ByteArkPlayerContainer from '@byteark/byteark-player-vue'
import type { ByteArkPlayerOptions, ByteArkPlayer } from '@byteark/byteark-player-vue'
const playerOptions: ByteArkPlayerOptions = {
autoplay: 'any',
fluid: true,
aspectRatio: '16:9',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
sources: [{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/TZyZheqEJUwC/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'TZyZheqEJUwC',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
}]
}
const onReady = (player: ByteArkPlayer) => {
// The player is ready! Initialize plugins here.
player.somePlugin()
}
</script>
<template>
<ByteArkPlayerContainer
:options="playerOptions"
/>
</template>
<script setup lang="ts">
import ByteArkPlayerContainer from '@byteark/byteark-player-vue'
import type { ByteArkPlayerOptions, ByteArkPlayer } from '@byteark/byteark-player-vue'
const playerOptions: ByteArkPlayerOptions = {
autoplay: 'any',
fluid: true,
aspectRatio: '16:9',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
sources: [{
src: 'https://byteark-playertzxedwv.stream-playlist.byteark.com/streams/TZyZheqEJUwC/playlist.m3u8',
type: 'application/x-mpegURL',
title: 'Big Buck Bunny',
videoId: 'TZyZheqEJUwC',
poster: 'https://stream-image.byteark.com/image/video-cover-480p/7/K/7KPloVWgN.png',
}],
html5: {
hlsjs: {
xhrSetup: function(xhr, url) {
xhr.withCredentials = true;
},
},
},
}
</script>
MIT © ByteArk Co. Ltd.
FAQs
ByteArk Player Container for Vue
We found that @byteark/byteark-player-vue demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.