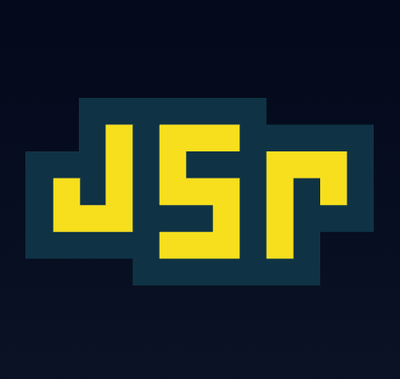
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@callstack/react-theme-provider
Advanced tools
@callstack/react-theme-provider is a library that allows you to easily manage and apply themes in your React applications. It provides a context-based solution for theming, enabling you to define and switch between different themes dynamically.
ThemeProvider
The ThemeProvider component is used to wrap your application or a part of it, providing the theme to all the components within its tree. This allows you to define a theme object and make it available throughout your application.
import { ThemeProvider } from '@callstack/react-theme-provider';
const theme = {
colors: {
primary: '#6200ee',
background: '#ffffff',
surface: '#ffffff',
accent: '#03dac4',
error: '#b00020',
text: '#000000',
onPrimary: '#ffffff',
onBackground: '#000000',
onSurface: '#000000',
onAccent: '#000000',
onError: '#ffffff',
},
};
const App = () => (
<ThemeProvider theme={theme}>
<YourComponent />
</ThemeProvider>
);
useTheme Hook
The useTheme hook allows you to access the current theme within your functional components. This hook returns the theme object, which you can then use to style your components dynamically based on the current theme.
import { useTheme } from '@callstack/react-theme-provider';
const ThemedComponent = () => {
const theme = useTheme();
return (
<div style={{ backgroundColor: theme.colors.background, color: theme.colors.text }}>
This component is themed!
</div>
);
};
withTheme Higher-Order Component
The withTheme higher-order component (HOC) is used to inject the theme into your class components. This HOC wraps your component and passes the theme as a prop, allowing you to access and use the theme within your class components.
import { withTheme } from '@callstack/react-theme-provider';
const ThemedComponent = ({ theme }) => (
<div style={{ backgroundColor: theme.colors.background, color: theme.colors.text }}>
This component is themed!
</div>
);
export default withTheme(ThemedComponent);
styled-components is a popular library for styling React applications using tagged template literals. It allows you to define styled components with dynamic theming capabilities. Compared to @callstack/react-theme-provider, styled-components offers a more integrated approach to styling and theming, combining both in a single library.
emotion is a performant and flexible CSS-in-JS library that provides powerful theming capabilities. It allows you to define and apply themes using its ThemeProvider and useTheme hook. Emotion is similar to @callstack/react-theme-provider in terms of theming functionality but also includes a comprehensive set of styling utilities.
theme-ui is a library for building themeable user interfaces based on constraint-based design principles. It provides a ThemeProvider, useTheme hook, and other utilities for managing themes. Compared to @callstack/react-theme-provider, theme-ui focuses on a more design-system-oriented approach, making it suitable for building consistent and scalable design systems.
@callstack/react-theme-provider
is a set of utilities that help you create your own theming system in few easy steps.
You can use it to customize colors, fonts, etc.
ThemeProvider
- componentwithTheme
- Higher Order ComponentcreateTheming(defaultTheme)
- factory returns ThemeProvider
component and withTheme
HOC with default theme injected.npm install --save @callstack/react-theme-provider
or using yarn
yarn add @callstack/react-theme-provider
To use, simply wrap your code into ThemeProvider
component and pass your theme as a theme
prop.
<ThemeProvider theme={{ primaryColor: 'red', background: 'gray'}}>
<App />
</ThemeProvider>
You could access theme data inside every component by wraping it into withTheme
HOC. Just like this:
class App extends React.Component {
render() {
return (
<div style={{ color: props.theme.primaryColor }}>
Hello
</div>
);
}
}
export default withTheme(App);
ThemeProvider
type:
type ThemeProviderType<T> = React.ComponentType<{
children?: any,
theme: T,
}>
Component you have to use to provide the theme to any component wrapped in withTheme
HOC.
-theme
- your theme object
withTheme
type:
type WithThemeType<T, S> = <C: React.ComponentType<*>>(
Comp: C
) => C &
React.ComponentType<
$Diff<React.ElementConfig<C>, { theme: T }> & { theme?: S }
>;
Classic Higher Order Component which takes your component as an argument and injects theme
prop into it.
const App = ({ theme }) => (
<div style={{ color: theme.primaryColor }}>
Hello
</div>
);
export withTheme(App);
It will inject the following props to the component:
theme
- our theme object.getWrappedInstance
- exposed by some HOCs like react-redux's connect
.
Use it to get the ref of the underlying element.You can also override theme
provided by ThemeProvider
by setting theme
prop on the component wrapped in withTheme
HOC.
Just like this:
const Button = withTheme(({ theme }) => (
<div style={{ color: theme.primaryColor }}>
Click me
</div>
));
const App = () => (
<ThemeProvider theme={{ primaryColor: 'red' }}>
<Button theme= {{ primaryColor: 'green' }}/>
</ThemeProvider>
)
In this example Button will have green text.
createTheming
type:
<T, S>(defaultTheme: T) => {
ThemeProvider: ThemeProviderType<T>,
withTheme: WithThemeType<T, S>,
}
This is more advanced replacement to classic importing ThemeProvider
and withTheme
directly from the library.
Thanks to it you can create your own ThemeProvider with any default theme.
Returns instance of ThemeProvider
component and withTheme
HOC.
You can use this factory to create a singleton with your instances of ThemeProvider
and withTheme
.
Note:
ThemeProvider
andwithTheme
generated bycreateTheming
always will use different context so make sure you are using matchingwithTheme
!
If you acidentially importwithTheme
from@callstack/react-theme-provider
instead of your theming instance it won't work.
defaultTheme
- default theme objectflow
types for your themeThemeProvider
s in your app without any conflicts.// theming.js
import { createTheming } from '@callstack/react-theme-provider';
const { ThemeProvider, withTheme } = createTheming({
primaryColor: 'red',
secondaryColor: 'green',
});
export { ThemeProvider, withTheme };
//App.js
import { ThemeProvider, withTheme } from './theming';
If you want to change the theme for a certain component, you can directly pass the theme prop to the component. The theme passed as the prop is merged with the theme from the Provider.
import * as React from 'react';
import MyButton from './MyButton';
export default function ButtonExample() {
return (
<MyButton theme={{ roundness: 3 }}>
Press me
</MyButton>
);
}
The ThemeProvider
exposes the theme to the components via React's context API,
which means that the component must be in the same tree as the ThemeProvider
. Some React Native components will render a
different tree such as a Modal
, in which case the components inside the Modal
won't be able to access the theme. The work
around is to get the theme using the withTheme
HOC and pass it down to the components as props, or expose it again with the
exported ThemeProvider
component.
FAQs
Theme provider for react and react-native applications
The npm package @callstack/react-theme-provider receives a total of 214,991 weekly downloads. As such, @callstack/react-theme-provider popularity was classified as popular.
We found that @callstack/react-theme-provider demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.