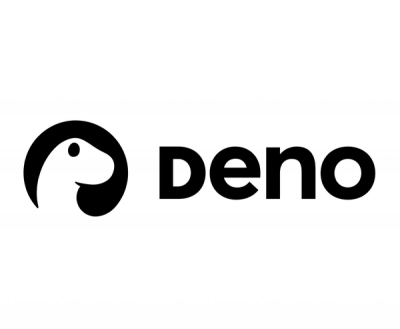
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@capacitor-firebase/analytics
Advanced tools
Unofficial Capacitor plugin for Firebase Analytics.1
npm install @capacitor-firebase/analytics firebase
npx cap sync
Add Firebase to your project if you haven't already (Android / iOS / Web).
This plugin will use the following project variables (defined in your app’s variables.gradle
file):
$firebaseAnalyticsVersion
version of com.google.firebase:firebase-analytics
(default: 21.2.2
)Add the CapacitorFirebaseAnalytics/Analytics
pod to your Podfile
(usually ios/App/Podfile
):
target 'App' do
capacitor_pods
# Add your Pods here
+ pod 'CapacitorFirebaseAnalytics/Analytics', :path => '../../node_modules/@capacitor-firebase/analytics'
end
Attention: Do not add the pod in the section def capacitor_pods
, but under the comment # Add your Pods here
(example).
See Disable Analytics data collection if you want to disable Analytics data collection.
If you want to install Firebase without any IDFA collection capability, use the CapacitorFirebaseAnalytics/AnalyticsWithoutAdIdSupport
pod in place of the CapacitorFirebaseAnalytics/Analytics
pod:
target 'App' do
capacitor_pods
# Add your Pods here
- pod 'CapacitorFirebaseAnalytics/Analytics', :path => '../../node_modules/@capacitor-firebase/analytics'
+ pod 'CapacitorFirebaseAnalytics/AnalyticsWithoutAdIdSupport', :path => '../../node_modules/@capacitor-firebase/analytics'
end
No configuration required for this plugin.
A working example can be found here: robingenz/capacitor-firebase-plugin-demo
import { FirebaseAnalytics } from '@capacitor-firebase/analytics';
const setUserId = async () => {
await FirebaseAnalytics.setUserId({
userId: '123',
});
};
const setUserProperty = async () => {
await FirebaseAnalytics.setUserProperty({
key: 'language',
value: 'en',
});
};
const setCurrentScreen = async () => {
await FirebaseAnalytics.setCurrentScreen({
screenName: 'Login',
screenClassOverride: 'LoginPage',
});
};
const logEvent = async () => {
await FirebaseAnalytics.logEvent({
name: 'sign_up',
params: { method: 'password' },
});
};
const setSessionTimeoutDuration = async () => {
await FirebaseAnalytics.setSessionTimeoutDuration({
duration: '120',
});
};
const setEnabled = async () => {
await FirebaseAnalytics.setEnabled({
enabled: true,
});
};
const isEnabled = async () => {
const { enabled } = await FirebaseAnalytics.isEnabled();
return enabled;
};
const resetAnalyticsData = async () => {
await FirebaseAnalytics.resetAnalyticsData();
};
getAppInstanceId()
setUserId(...)
setUserProperty(...)
setCurrentScreen(...)
logEvent(...)
setSessionTimeoutDuration(...)
setEnabled(...)
isEnabled()
resetAnalyticsData()
getAppInstanceId() => Promise<GetAppInstanceIdResult>
Retrieves the app instance id.
Only available for Android and iOS.
Returns: Promise<GetAppInstanceIdResult>
Since: 1.4.0
setUserId(options: SetUserIdOptions) => Promise<void>
Sets the user ID property.
Param | Type |
---|---|
options | SetUserIdOptions |
Since: 0.1.0
setUserProperty(options: SetUserPropertyOptions) => Promise<void>
Sets a custom user property to a given value.
Param | Type |
---|---|
options | SetUserPropertyOptions |
Since: 0.1.0
setCurrentScreen(options: SetCurrentScreenOptions) => Promise<void>
Sets the current screen name.
Param | Type |
---|---|
options | SetCurrentScreenOptions |
Since: 0.1.0
logEvent(options: LogEventOptions) => Promise<void>
Logs an app event.
Param | Type |
---|---|
options | LogEventOptions |
Since: 0.1.0
setSessionTimeoutDuration(options: SetSessionTimeoutDurationOptions) => Promise<void>
Sets the duration of inactivity that terminates the current session.
Only available for Android and iOS.
Param | Type |
---|---|
options | SetSessionTimeoutDurationOptions |
Since: 0.1.0
setEnabled(options: SetEnabledOptions) => Promise<void>
Enables/disables automatic data collection. The value does not apply until the next run of the app.
Param | Type |
---|---|
options | SetEnabledOptions |
Since: 0.1.0
isEnabled() => Promise<IsEnabledResult>
Returns whether or not automatic data collection is enabled.
Only available for Web.
Returns: Promise<IsEnabledResult>
Since: 0.1.0
resetAnalyticsData() => Promise<void>
Clears all analytics data for this app from the device. Resets the app instance id.
Only available for Android and iOS.
Since: 0.1.0
Prop | Type | Description | Since |
---|---|---|---|
appInstanceId | string | The app instance id. Not defined if FirebaseAnalytics.ConsentType.ANALYTICS_STORAGE has been set to FirebaseAnalytics.ConsentStatus.DENIED . | 1.4.0 |
Prop | Type | Since |
---|---|---|
userId | string | null | 0.1.0 |
Prop | Type | Since |
---|---|---|
key | string | 0.1.0 |
value | string | null | 0.1.0 |
Prop | Type | Description | Default | Since |
---|---|---|---|---|
screenName | string | null | 0.1.0 | ||
screenClassOverride | string | null | Only available for Android and iOS. | null | 0.1.0 |
Prop | Type | Description | Since |
---|---|---|---|
name | string | The event name. | 0.1.0 |
params | { [key: string]: any; } | The optional event params. | 0.1.0 |
Prop | Type | Description | Default | Since |
---|---|---|---|---|
duration | number | Duration in seconds. | 1800 | 0.1.0 |
Prop | Type | Since |
---|---|---|
enabled | boolean | 0.1.0 |
Prop | Type | Since |
---|---|---|
enabled | boolean | 0.1.0 |
Here you can find more information on how to test the Firebase Analytics implementation using the DebugView.
See CHANGELOG.md.
See LICENSE.
This project is not affiliated with, endorsed by, sponsored by, or approved by Google LLC or any of their affiliates or subsidiaries. ↩
FAQs
Capacitor plugin for Firebase Analytics.
We found that @capacitor-firebase/analytics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.