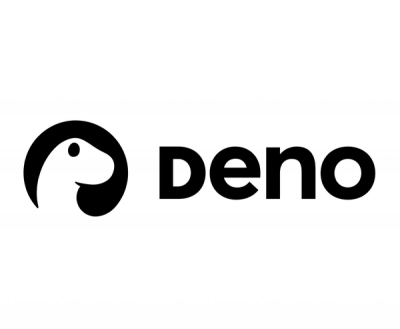
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@celo/contractkit
Advanced tools
Celo's ContractKit is a library to help developers and validators to interact with the Celo blockchain.
ContractKit supports the following functionality:
:::tip
You might not need the full ContractKit. Consider using @celo/connect
which powers much of ContractKit such as building and sending Transactions, signing, etc, but does not give access to any celo Contract Wrappers. Or if a subset of Wrappers, setting the feeCurrency and account info is all your dapp needs consider replacing your imports of Contractkit with @celo/contractkit/lib/mini-kit
:::
To install:
npm install @celo/contractkit
// or
yarn add @celo/contractkit
You will need Node.js v12.x. or greater.
To start working with contractkit you need a kit
instance:
import { newKit } from '@celo/contractkit' // or import { newKit } from '@celo/contractkit/lib/mini-kit'
// Remotely connect to the Alfajores testnet
const kit = newKit('https://alfajores-forno.celo-testnet.org')
To access balances:
// returns an object with {lockedGold, pending, cUSD, cEUR, cREAL}
const balances = await kit.getTotalBalance()
// returns an object with {cUSD, cEUR, cREAL}
const balances = await miniKit.getTotalBalance()
If you don't need the balances of all tokens use the balanceOf method
const stableTokenWrapper = await kit.getStableToken(StableToken.cREAL)
const cRealBalance = stableTokenWrapper.balanceOf(accountAddress)
kit
allows you to set default transaction options:
import { newKit, CeloContract } from '@celo/contractkit/lib/mini-kit'
async function getKit(myAddress: string, privateKey: string) {
const kit = newKit('https://alfajores-forno.celo-testnet.org')
// default from account
kit.defaultAccount = myAddress
// add the account private key for tx signing when connecting to a remote node
kit.connection.addAccount(privateKey)
// paid gas in celo dollars
await kit.setFeeCurrency(CeloContract.StableToken)
return kit
}
Celo has two initial coins: CELO and cUSD (stableToken). Both implement the ERC20 standard, and to interact with them is as simple as:
// get the CELO contract
const celoToken = await kit.contracts.getGoldToken()
// get the cUSD contract
const stableToken = await kit.contracts.getStableToken()
const celoBalance = await celoToken.balanceOf(someAddress)
const cusdBalance = await stableToken.balanceOf(someAddress)
To send funds:
const oneGold = kit.connection.web3.utils.toWei('1', 'ether')
const tx = await goldToken.transfer(someAddress, oneGold).send({
from: myAddress
})
const hash = await tx.getHash()
const receipt = await tx.waitReceipt()
If you would like to pay fees in cUSD, (or other cStables like cEUR, cUSD).
kit.setFeeCurrency(CeloContract.StableToken) // Default to paying fees in cUSD
const stableTokenContract = kit.contracts.getStableToken()
const tx = await stableTokenContract
.transfer(recipient, weiTransferAmount)
.send({ from: myAddress, gasPrice })
const hash = await tx.getHash()
const receipt = await tx.waitReceipt()
There are many core contracts.
When using the kit
you can access core contracts like
kit.contracts.get{ContractName}
E.G. kit.contracts.getAccounts()
, kit.contracts.getValidators()
You can also initialize contracts wrappers directly. They require a Connection
and their contract:
// MiniContractKit only gives access to a limited set of Contracts, so we import Multisig
import { newKit } from "@celo/contractkit/lib/mini-kit"
import { MultiSigWrapper } from '@celo/contractkit/lib/wrappers/MultiSig'
import { newMultiSig } from '@celo/contractkit/lib/generated/MultiSig'
const miniKit = newKit("https://alfajores-forno.celo-testnet.org/")
// Alternatively import { Connection } from '@celo/connect'
// const connection = new Connection(web3)
const contract = newMultiSig(web3)
const multisigWrapper = new MultiSigWrapper(miniKit.connection, contract)
MiniContractKit
does not provide access to the web3 contracts
Some user might want to access web3 native contract wrappers.
To do so, you can:
const web3Exchange = await kit._web3Contracts.getExchange()
We expose native wrappers for all Celo core contracts.
The complete list of Celo Core contracts is:
Celo Core Contracts addresses, can be obtained by looking at the Registry
contract.
That's how kit
obtains them.
We expose the registry API, which can be accessed by:
const goldTokenAddress = await kit.registry.addressFor(CeloContract.GoldToken)
Celo transaction object is not the same as Ethereum's. There are three new fields present:
:::note
The gatewayFeeRecipient
, and gatewayFee
fields are currently not used by the protocol.
:::
This means that using web3.eth.sendTransaction
or myContract.methods.transfer().send()
should be avoided to take advantage of paying transaction fees in alternative currencies.
Instead, kit
provides an utility method to send transaction in both scenarios. If you use contract wrappers, there is no need to use this.
For a raw transaction:
const tx = kit.sendTransaction({
from: myAddress,
to: someAddress,
value: oneGold,
})
const hash = await tx.getHash()
const receipt = await tx.waitReceipt()
When interacting with a web3 contract object:
const celoNativeToken = await kit._web3Contracts.getGoldToken()
const oneGold = kit.connection.web3.utils.toWei('1', 'ether')
const txo = await celoNativeToken.methods.transfer(someAddress, oneGold)
const tx = await kit.sendTransactionObject(txo, { from: myAddress })
const hash = await tx.getHash()
const receipt = await tx.waitReceipt()
You can find more information about the ContractKit in the Celo docs at https://docs.celo.org/developer-guide/contractkit.
If you need to debug kit
, we use the well known debug node library.
So set the environment variable DEBUG
as:
DEBUG="kit:*,
FAQs
Celo's ContractKit to interact with Celo network
The npm package @celo/contractkit receives a total of 21,531 weekly downloads. As such, @celo/contractkit popularity was classified as popular.
We found that @celo/contractkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.