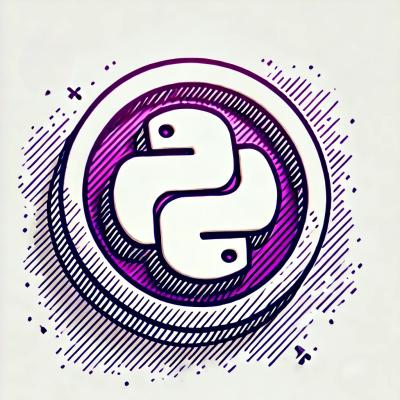
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@cevad-tokatli/slider-react
Advanced tools
React integration for Slider.
It is available as a package on NPM for use with a module bundler.
# NPM
$ npm install --save @cevad-tokatli/slider-react
# Yarn
$ yarn add @cevad-tokatli/slider-react
import '@cevad-tokatli/slider/style.css'
import React from 'react'
import Slider, { Element } from '@cevad-tokatli/slider-react'
export default class App extends React.Component {
render() {
return(
<div>
<Slider>
<Element>
<img src="img1.jpg" />
</Element>
<Element>
<img src="img2.jpg" />
</Element>
<Element>
<img src="img3.jpg" />
</Element>
</Slider>
</div>
)
}
}
Option | Type | Default | Description |
---|---|---|---|
timing | string | "ease" | Specifies the speed curve of an animation. Takes all the values CSS3 can take. (like ease, linear, cubic-bezier, step) |
duration | number | 800 | Defines how long an animation should take to complete one cycle. (as milliseconds) |
sliderType | SliderType | Carousel | Specifies the animation type. It can be customized for each image element by passing as prop to the element component. |
touchMove | boolean | true | Enables slide transitive with touch. |
list | boolean | true | Displays a list at the bottom of the slider. |
asList | string | HTMLUListElement | HTMLOListElement* | null | Declares the given list as the slider list. |
arrows | boolean | true | Displays right and left arrows to change the index. |
asPrevArrow | string | HTMLElement* | null | Declares the given element as the prev arrow. |
asNextArrow | string | HTMLElement* | null | Declares the given element as the next arrow. |
autoPlay | boolean | false | Enables auto play of slides. |
autoPlaySpeed | number | 5000 | Sets auto play interval. (as milliseconds) |
*: You can give an HTML element or a CSS selector (like #carousel
, .container > div:first-child
)
import Slider, { Element, SliderType } from '@cevad-tokatli/slider-react'
render() {
return (
<div>
<Slider
timing="linear"
sliderType={SliderType.Fade}
>
<Element
sliderType={SliderType.Flow}
>
<img src="img1.jpg" />
</Element>
<Element>
<img src="img2.jpg" />
</Element>
<Element
sliderType={SliderType.Carousel}
>
<img src="img3.jpg" />
</Element>
<Element>
<img src="img4.jpg" />
</Element>
</Slider>
</div>
)
}
It is a object which specifies one single slider element. It has the following attributes.
Specfies the slider animation type.
You can declare your list as a slider list.
before(current: SliderElement, next: SliderElement): Promise
It is invoked before animation runs. It returns a promise so that animation waits for the mehtod to complete.
after(current: SliderElement, prev: SliderElement): Promise
It is invoked after animation runs. It returns a promise so before the method completes running, another animation cannot run.
You can pass callbacks as props Slider component or to specify for one element to Element component.
before(current, next) {
return new Promise(resolve => {
// ...
resolve()
})
}
render() {
return (
<div>
<Slider
before={this.before}
>
<Element>
<img src="img1.jpg" />
</Element>
<Element>
<img src="img2.jpg" />
</Element>
<Element>
<img src="img3.jpg" />
</Element>
</Slider>
</div>
)
}
before(el:SliderElement, active:boolean): Promise
It is invoked before animation runs. It returns a promise so that animation waits for the mehtod to complete. It is only invoked when it is the current or next element. If it is the next element, active is true.
after(el:SliderElement, active:boolean): Promise
It is invoked after animation runs. It returns a promise so before the method completes runing, another animation cannot run. It is only invoked when it is the current or previous element. If it is the current element, active is true.
after(current, active) {
return new Promise(resolve => {
// ...
resolve()
})
}
render() {
return (
<div>
<Slider>
<Element
after={this.after}
>
<img src="img1.jpg" />
</Element>
<Element>
<img src="img2.jpg" />
</Element>
<Element>
<img src="img3.jpg" />
</Element>
</Slider>
</div>
)
}
Event | Description |
---|---|
touchStart | Fires when touching starts. |
touchEnd | Fires when touching ends. |
change | Fires when index changes. |
play | Fires when autoplay starts. |
stop | Fires when autoplay stops. |
destroy | Fires when the slider is destroyed. |
componentDidMount() {
this.slider.el.addEventListener('touchStart', () => {
console.log('touching starts')
})
this.slider.el.addEventListener('touchEnd', () => {
console.log('touching ends')
})
}
render() {
return (
<div>
<Slider ref={node => this.slider = node}>
// ...
</Slider>
</div>
)
}
Method | Params | Return | Description |
---|---|---|---|
prev | Promise<boolean> | Triggers the previous image. Returns false if the slider is in animation. | |
next | Promise<boolean> | Triggers the next image. Returns false if the slider is in animation. | |
play | void | Starts autoplay. | |
stop | void | Stops autoplay. | |
toggle | void | Toggles autoplay. | |
destroy | void | Destroys the slider. | |
getIndex | number | Returns index. | |
setIndex | index: number | Promise<boolean> | Sets index and animates the slider. Returns false if the slider is in animation. |
getTotal | number | Returns total number of images. | |
getCurrent | SliderElement | Returns the current element. | |
getTiming | string | Returns timing value. | |
setTiming | timing: string | void | Sets timing value. |
getDuration | number | Returns duration. | |
setDuration | duration: number | void | Sets duration. |
getAutoPlaySpeed | number | Returns auto play speed. | |
setAutoPlaySpeed | speed: number | void | Sets auto play speed. |
*: You can give an HTML element or a CSS selector (like #carousel
, .container > div:first-child
)
Slider React is provided under the MIT License.
FAQs
React version of Slider
The npm package @cevad-tokatli/slider-react receives a total of 0 weekly downloads. As such, @cevad-tokatli/slider-react popularity was classified as not popular.
We found that @cevad-tokatli/slider-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.