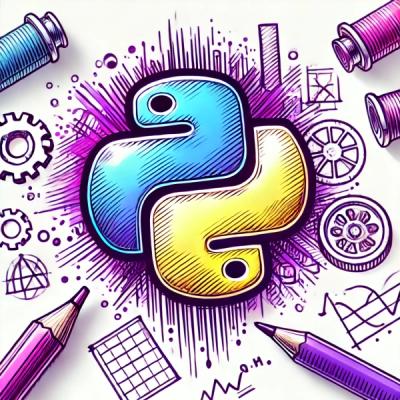
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
@cfworker/json-schema
Advanced tools
A JSON schema validator that will run on Cloudflare workers. Supports drafts 4, 7, 2019-09, and 2020-12.
@cfworker/json-schema is a lightweight and fast JSON schema validator and parser that is designed to work in both Node.js and browser environments. It is part of the Cloudflare Workers suite of tools, which are optimized for performance and efficiency.
Schema Validation
This feature allows you to validate JSON data against a defined schema. The code sample demonstrates creating a schema for an object with 'name' and 'age' properties, and then validating a data object against this schema.
const { Validator } = require('@cfworker/json-schema');
const schema = {
type: 'object',
properties: {
name: { type: 'string' },
age: { type: 'integer', minimum: 0 }
},
required: ['name', 'age']
};
const validator = new Validator(schema);
const data = { name: 'John Doe', age: 30 };
const result = validator.validate(data);
console.log(result.valid); // true
console.log(result.errors); // []
Schema Compilation
This feature allows you to compile a JSON schema into a validation function. The code sample shows how to compile a schema and use the resulting function to validate data.
const { compile } = require('@cfworker/json-schema');
const schema = {
type: 'object',
properties: {
email: { type: 'string', format: 'email' }
},
required: ['email']
};
const validate = compile(schema);
const data = { email: 'test@example.com' };
const valid = validate(data);
console.log(valid); // true
Ajv is a popular JSON schema validator that supports JSON Schema draft-04, draft-06, draft-07, and draft-2019-09. It is known for its high performance and extensive feature set, including support for asynchronous validation, custom keywords, and formats. Compared to @cfworker/json-schema, Ajv offers more features and flexibility but may be heavier in terms of bundle size.
Joi is a powerful schema description language and data validator for JavaScript objects. It is part of the hapi ecosystem and is known for its expressive and intuitive API. While Joi is not strictly a JSON schema validator, it provides similar functionality with a focus on server-side validation. Compared to @cfworker/json-schema, Joi offers a more expressive API but is not designed specifically for JSON Schema.
The jsonschema package is a simple and easy-to-use JSON schema validator for Node.js. It supports JSON Schema draft-04 and is suitable for basic validation needs. Compared to @cfworker/json-schema, jsonschema is simpler and may not offer the same level of performance or browser compatibility.
A JSON schema validator that will run on Cloudflare workers. Supports drafts 4, 7, 2019-09, and 2020-12.
This library is validated against the json-schema-test-suite, a series of approximately 4,500 assertions maintained along with the json-schema specification. A small set of test cases are intentionally not supported due to performance constraints or lack of feature use. These list of unsupported features are maintained in test/unsupported.ts. While this library is not the fastest due to lack of code generation, it's consistently among the most spec compliant.
Why another JSON schema validator?
Cloudflare workers do not have APIs required by Ajv schema compilation (eval
or new Function(code)
).
If possible use Ajv in a build step to precompile your schema. Otherwise this library could work for you.
import { Validator } from '@cfworker/json-schema';
const validator = new Validator({ type: 'number' });
const result = validator.validate(7);
const validator = new Validator({ type: 'number' }, '4'); // draft-4
const validator = new Validator({
$id: 'https://foo.bar/baz',
$ref: '/beep'
});
validator.addSchema({ $id: 'https://foo.bar/beep', type: 'boolean' });
By default the validator stops processing after the first error. Set the shortCircuit
parameter to false
to emit all errors.
const shortCircuit = false;
const draft = '2019-09';
const schema = {
type: 'object',
required: ['name', 'email', 'number', 'bool'],
properties: {
name: { type: 'string' },
email: { type: 'string', format: 'email' },
number: { type: 'number' },
bool: { type: 'boolean' }
}
};
const validator = new Validator(schema, draft, shortCircuit);
const result = validator.validate({
name: 'hello',
email: 5, // invalid type
number: 'Hello' // invalid type
bool: 'false' // invalid type
});
FAQs
A JSON schema validator that will run on Cloudflare workers. Supports drafts 4, 7, 2019-09, and 2020-12.
The npm package @cfworker/json-schema receives a total of 145,393 weekly downloads. As such, @cfworker/json-schema popularity was classified as popular.
We found that @cfworker/json-schema demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.