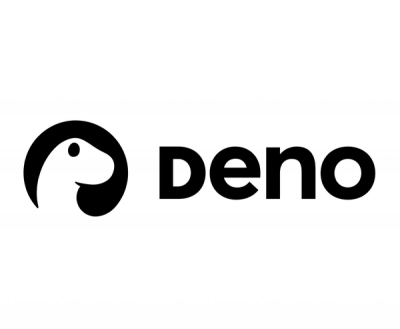
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@chainlink/gauntlet-core
Advanced tools
TODO: Explain the package and its components: Operations, Client, Dependencies, Operation Runner, etc.
TODO: Explain the package and its components: Operations, Client, Dependencies, Operation Runner, etc.
Interceptors are used to extract and treat information that goes through the Gauntlet Client.
The Gauntlet Client expects an optional interceptors
parameter, which is an optional list of implementations of the ClientInterceptor
interface. As ClientInterceptor
intercepts Gauntlet Client calls, is expected that the interface has similarities with the Gauntlet Client interface.
export interface ClientInterceptor {
execute: (input: InterceptorExecuteInput, next: Next<this, 'execute'>) => Promise<Report>
plan: (input: InterceptorPlanInput, next: Next<this, 'plan'>) => Promise<Report>
query: (input: InterceptorQueryInput, next: Next<this, 'query'>) => Promise<Report>
}
A simple implementation could just be for logging purposes:
export class LoggerClientInterceptor implements ClientInterceptor {
readonly log: Logger
constructor(logger: Logger) {
this.log = logger
}
async execute(
input: InterceptorExecuteInput,
next: Next<ClientInterceptor, 'execute'>,
): Promise<ReportAny> {
this.log.info(`Execution starting with plan: ${JSON.stringify(input.plan)}`)
const report = await next(input)
this.log.info(`Execution finished with report: ${JSON.stringify(report)}`)
return report
}
async plan(
input: InterceptorPlanInput,
next: Next<ClientInterceptor, 'plan'>,
): Promise<ReportAny> {
this.log.info(`Command plan stage starting with input: ${JSON.stringify(input.input)}`)
const report = await next(input)
this.log.info(`Plan stage finished with report: ${JSON.stringify(report)}`)
return report
}
// ...
}
The user can enable many Interceptors in the client by just providing implementations of ClientInterceptor
;(async () => {
// We configure the interceptors at building time in the client
const client = buildClient(packages, factories, [
new LoggerClientInterceptor(),
new TracingInterceptor(),
])
const report = client.plan(op.def, input, config, traceExtra) // we can expect this execution to have both logging and tracing interceptions
})()
TODO!
TODO!
The ctx.emit
function allows developers to add custom events with associated data when planning an operation, which can then be aggregated or reported in a structured format.
ctx.emit
The ctx.emit
function is defined as follows:
emit: (msg: string, payload?: EventPayload) => void
Emitting a Diff Event
When there's a change in state that needs to be captured:
ctx.emit('Would add a new owner to the safe', asDiffEvent(prev, head))
Emitting a Generic Event
For less structured events:
ctx.emit('Any event', { type: 'any', object: 5, a: { c: 2 } })
Emitting Without Payload
Simply sending a message without additional data:
ctx.emit('without payload')
FAQs
TODO: Explain the package and its components: Operations, Client, Dependencies, Operation Runner, etc.
We found that @chainlink/gauntlet-core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.