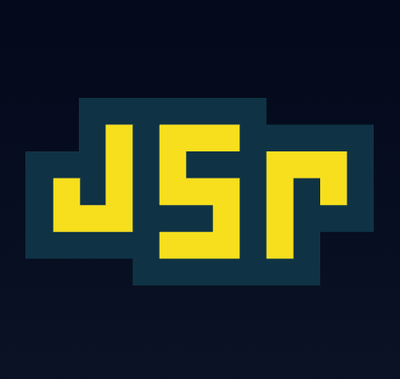
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@chakra-ui/menu
Advanced tools
@chakra-ui/menu is a component library for creating accessible and customizable menu components in React applications. It is part of the Chakra UI library, which provides a set of accessible, reusable, and composable React components.
Basic Menu
This code demonstrates how to create a basic menu with a button that triggers a dropdown list of menu items.
```jsx
import { Menu, MenuButton, MenuList, MenuItem, Button } from '@chakra-ui/react';
function BasicMenu() {
return (
<Menu>
<MenuButton as={Button}>
Actions
</MenuButton>
<MenuList>
<MenuItem>Download</MenuItem>
<MenuItem>Create a Copy</MenuItem>
<MenuItem>Mark as Draft</MenuItem>
<MenuItem>Delete</MenuItem>
<MenuItem>Attend a Workshop</MenuItem>
</MenuList>
</Menu>
);
}
```
Menu with Submenu
This code demonstrates how to create a menu with grouped items and a submenu, providing a more organized structure for menu options.
```jsx
import { Menu, MenuButton, MenuList, MenuItem, MenuGroup, MenuDivider, Button } from '@chakra-ui/react';
function MenuWithSubmenu() {
return (
<Menu>
<MenuButton as={Button}>
File
</MenuButton>
<MenuList>
<MenuGroup title="Profile">
<MenuItem>My Account</MenuItem>
<MenuItem>Payments </MenuItem>
</MenuGroup>
<MenuDivider />
<MenuGroup title="Help">
<MenuItem>Docs</MenuItem>
<MenuItem>FAQ</MenuItem>
</MenuGroup>
</MenuList>
</Menu>
);
}
```
Menu with Icons
This code demonstrates how to add icons to menu items, enhancing the visual appeal and usability of the menu.
```jsx
import { Menu, MenuButton, MenuList, MenuItem, Button } from '@chakra-ui/react';
import { FaDownload, FaCopy, FaTrash } from 'react-icons/fa';
function MenuWithIcons() {
return (
<Menu>
<MenuButton as={Button}>
Actions
</MenuButton>
<MenuList>
<MenuItem icon={<FaDownload />}>Download</MenuItem>
<MenuItem icon={<FaCopy />}>Create a Copy</MenuItem>
<MenuItem icon={<FaTrash />}>Delete</MenuItem>
</MenuList>
</Menu>
);
}
```
react-select is a flexible and customizable library for building dropdowns and select inputs in React. It offers a wide range of features including multi-select, async options, and customizable styles. Compared to @chakra-ui/menu, react-select is more focused on select inputs rather than general-purpose menus.
rc-menu is a React component library for creating menu components. It provides a set of features for building complex menus, including nested menus and keyboard navigation. While rc-menu offers similar functionalities to @chakra-ui/menu, it may require more customization to achieve the same level of accessibility and styling provided by Chakra UI.
react-burger-menu is a library for creating off-canvas side menus in React. It is highly customizable and supports various animations and styles. Unlike @chakra-ui/menu, which focuses on dropdown menus, react-burger-menu is designed for creating sidebar menus.
An accessible dropdown menu for the common dropdown menu button design pattern. Menu uses roving tabIndex for focus management.
yarn add @chakra-ui/menu
# or
npm i @chakra-ui/menu
import {
Menu,
MenuButton,
MenuList,
MenuItem,
MenuGroup,
MenuDivider,
MenuOptionGroup,
MenuItemOption,
} from "@chakra-ui/react"
<Menu>
<MenuButton>Actions</MenuButton>
<MenuList>
<MenuItem>Download</MenuItem>
<MenuItem>Create a Copy</MenuItem>
<MenuItem>Mark as Draft</MenuItem>
<MenuItem>Delete</MenuItem>
<MenuItem as="a" href="#">
Attend a Workshop
</MenuItem>
</MenuList>
</Menu>
To access the internal state of the Menu, use a function as a children
(commonly known as a render prop). You'll get access to the internal state
isOpen
and method onClose
.
<Menu>
{({ isOpen }) => (
<React.Fragment>
<MenuButton>{isOpen ? "Close" : "Open"}</MenuButton>
<MenuList>
<MenuItem>Download</MenuItem>
<MenuItem onClick={() => alert("Kagebunshin")}>Create a Copy</MenuItem>
</MenuList>
</React.Fragment>
)}
</Menu>
When focus is on the MenuButton
or within the MenuList
and you type a letter
key, a search begins. Focus will move to the first MenuItem
that starts with
the letter you typed.
Open the menu, try and type any letter, say "S" to see the focus movement.
<Menu>
<MenuButton
px={4}
py={2}
transition="all 0.2s"
borderRadius="md"
borderWidth="1px"
_hover={{ bg: "gray.100" }}
_expanded={{ bg: "red.200" }}
_focus={{ outline: 0, boxShadow: "outline" }}
>
File <ChevronDownIcon />
</MenuButton>
<MenuList>
<MenuItem>New File</MenuItem>
<MenuItem>New Window</MenuItem>
<MenuDivider />
<MenuItem>Open...</MenuItem>
<MenuItem>Save File</MenuItem>
</MenuList>
</Menu>
<Menu>
<MenuButton>Your Cats</MenuButton>
<MenuList>
<MenuItem minH="48px">
<Image
size="2rem"
borderRadius="full"
src="https://placekitten.com/100/100"
alt="Fluffybuns the destroyer"
mr="12px"
/>
<span>Fluffybuns the Destroyer</span>
</MenuItem>
<MenuItem minH="40px">
<Image
size="2rem"
borderRadius="full"
src="https://placekitten.com/120/120"
alt="Simon the pensive"
mr="12px"
/>
<span>Simon the pensive</span>
</MenuItem>
</MenuList>
</Menu>
To group related MenuItems
, use the MenuGroup
component and pass it a label
for the group name.
<Menu>
<MenuButton>Profile</MenuButton>
<MenuList>
<MenuGroup title="Profile">
<MenuItem>My Account</MenuItem>
<MenuItem>Payments </MenuItem>
</MenuGroup>
<MenuDivider />
<MenuGroup title="Help">
<MenuItem>Docs</MenuItem>
<MenuItem>FAQ</MenuItem>
</MenuGroup>
</MenuList>
</Menu>
You can compose a menu for table headers to help with sorting and filtering
options. Use the MenuOptionGroup
and MenuItemOption
components.
<Menu closeOnSelect={false}>
<MenuButton>MenuItem</MenuButton>
<MenuList minWidth="240px">
<MenuOptionGroup defaultValue="asc" title="Order" type="radio">
<MenuItemOption value="asc">Ascending</MenuItemOption>
<MenuItemOption value="desc">Descending</MenuItemOption>
</MenuOptionGroup>
<MenuDivider />
<MenuOptionGroup title="Country" type="checkbox">
<MenuItemOption value="email">Email</MenuItemOption>
<MenuItemOption value="phone">Phone</MenuItemOption>
<MenuItemOption value="country">Country</MenuItemOption>
</MenuOptionGroup>
</MenuList>
</Menu>
closeOnSelect
MenuItem
and MenuItemOption
can use the closeOnSelect
prop to override
their parent Menu
's behavior.
<Menu>
<MenuButton>Open Menu</MenuButton>
<MenuList>
<MenuGroup title="Profile">
{/* Clicking on those items will close the menu (default behavior) */}
<MenuItem>My Account</MenuItem>
<MenuItem>Payments</MenuItem>
</MenuGroup>
<MenuDivider />
<MenuOptionGroup title="Country" type="checkbox">
{/* Clicking on those items will keep the menu open */}
<MenuItemOption value="email" closeOnSelect={false}>
Email
</MenuItemOption>
<MenuItemOption value="phone" closeOnSelect={false}>
Phone
</MenuItemOption>
<MenuItemOption value="country" closeOnSelect={false}>
Country
</MenuItemOption>
</MenuOptionGroup>
</MenuList>
</Menu>
FAQs
A React component to render accessible menus
The npm package @chakra-ui/menu receives a total of 382,607 weekly downloads. As such, @chakra-ui/menu popularity was classified as popular.
We found that @chakra-ui/menu demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.