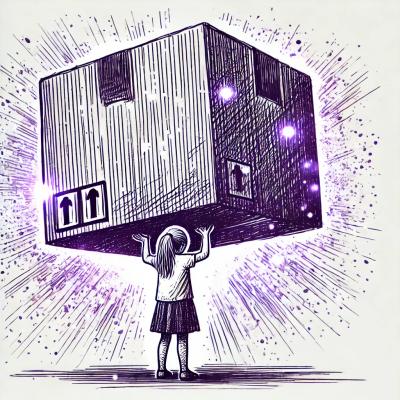
Security News
The Unpaid Backbone of Open Source: Solo Maintainers Face Increasing Security Demands
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
@chakra-ui/toast
Advanced tools
@chakra-ui/toast is a part of the Chakra UI library that provides a simple and customizable way to display toast notifications in a React application. It allows developers to show brief messages to users, such as success, error, warning, or informational messages, in a non-intrusive manner.
Basic Toast
This feature allows you to display a basic toast notification with a title, description, status, duration, and closable option.
import { useToast } from '@chakra-ui/react';
function Example() {
const toast = useToast();
return (
<button
onClick={() =>
toast({
title: 'Account created.',
description: 'We've created your account for you.',
status: 'success',
duration: 5000,
isClosable: true,
})
}
>
Show Toast
</button>
);
}
Custom Toast
This feature allows you to create a custom toast notification by rendering a custom component inside the toast.
import { useToast, Box, Button } from '@chakra-ui/react';
function CustomToast() {
const toast = useToast();
return (
<Button
onClick={() =>
toast({
position: 'bottom-left',
render: () => (
<Box color='white' p={3} bg='blue.500'>
Hello World
</Box>
),
})
}
>
Show Custom Toast
</Button>
);
}
Positioning Toasts
This feature allows you to position the toast notification at different locations on the screen, such as top-right, bottom-left, etc.
import { useToast, Button } from '@chakra-ui/react';
function PositionedToast() {
const toast = useToast();
return (
<Button
onClick={() =>
toast({
title: 'Notification',
description: 'This is a toast notification.',
status: 'info',
position: 'top-right',
duration: 3000,
isClosable: true,
})
}
>
Show Positioned Toast
</Button>
);
}
React-Toastify is a popular library for adding toast notifications to React applications. It offers a wide range of customization options and is known for its ease of use. Compared to @chakra-ui/toast, React-Toastify provides more built-in features like progress bars and different transition animations.
Notistack is a highly customizable notification library for React. It allows stacking of notifications and provides a flexible API for managing them. Compared to @chakra-ui/toast, Notistack offers more advanced features like stacking and dismissing notifications programmatically.
React-Hot-Toast is a lightweight and customizable toast notification library for React. It focuses on simplicity and performance. Compared to @chakra-ui/toast, React-Hot-Toast is more minimalistic and offers a simpler API for quick integration.
The toast is used to show alerts on top of an overlay.
The toast will close itself when the close button is clicked, or after a timeout — the default is 5 seconds.
Toasts can be configured to appear at either the top or the bottom of an application window, and it is possible to have more than one toast onscreen at a time.
import useToast from "@chakra-ui/toast"
function ToastExample() {
const toast = useToast()
return (
<Button
onClick={() =>
toast({
title: "Account created.",
description: "We've created your account for you.",
status: "success",
duration: 9000,
isClosable: true,
})
}
>
Show Toast
</Button>
)
}
By default, all the toasts will be positioned on the top-right
of your
browser.
The following values are allowed: top-right, top, top-left, bottom-right, bottom, bottom-left
import * as React from "react"
import useToast from "@chakra-ui/toast"
const Position = () => {
const toast = useToast()
const notify = () => {
toast({ title: "Success Notification !", status: "success" })
}
return <button onClick={notify}>Notify</button>
}
To change the show delay for any toast, simply pass the duration
prop when
invoking the toast
function.
Note 🚨: If you pass
null
as the duration, the toast will always remain on screen.
import * as React from "react"
import useToast from "@chakra-ui/toast"
const Duration = () => {
const toast = useToast()
const notify = () => {
toast({
duration: 5000,
title: "I will close after 5 secs",
})
}
return <button onClick={notify}>Notify</button>
}
Display a custom component instead of the default Toast UI.
We provide 2 key props
to your component, id
, and onClose
to close the
toast (to build your custom close button).
function Example() {
const toast = useToast()
return (
<Button
onClick={() => {
toast({
position: "bottom-left",
render: (props) => (
<Box m={3} color="white" p={3} bg="blue.500">
Hello World
</Box>
),
})
}}
>
Show Toast
</Button>
)
}
A custom id
can be used to replace the one internal auto-generated toast id
.
You can use a number
or a string
.
This is mostly useful when you need to prevent duplication of a specific toast.
To prevent duplicates, you can check if a given toast is active by calling
toast.isActive(id)
like the snippet below. Or, you can use a custom toastId
:
import * as React from "react"
import useToast from "@chakra-ui/toast"
const Example = () => {
const toast = useToast()
const id = "login-toast"
const notify = () => {
if (!toast.isActive(id)) {
toast({ title: "Dude! I cannot be duplicated" })
}
}
return (
<div>
<button onClick={notify}>Notify</button>
</div>
)
}
When you update a toast, the toast options and the content are inherited but don't worry you can update them.
import * as React from "react"
import useToast from "@chakra-ui/toast"
const Update = () => {
const toast = useToast()
const id = React.useRef(null)
const notify = () => {
id.current = toast({
title: "Chidori is not available!",
duration: null,
})
}
const update = () => {
toast.update(id.current, {
title: "Sharingan is all you have!",
status: "success",
duration: 5000,
})
}
return (
<div>
<button onClick={notify}>Notify</button>
<button onClick={update}>Update</button>
</div>
)
}
FAQs
description
The npm package @chakra-ui/toast receives a total of 546,159 weekly downloads. As such, @chakra-ui/toast popularity was classified as popular.
We found that @chakra-ui/toast demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Solo open source maintainers face burnout and security challenges, with 60% unpaid and 60% considering quitting.
Security News
License exceptions modify the terms of open source licenses, impacting how software can be used, modified, and distributed. Developers should be aware of the legal implications of these exceptions.
Security News
A developer is accusing Tencent of violating the GPL by modifying a Python utility and changing its license to BSD, highlighting the importance of copyleft compliance.