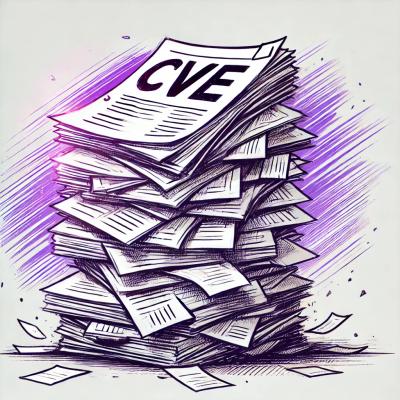
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@chargebee/chargebee-js-angular-wrapper
Advanced tools
Angular wrapper for Chargebee.js Components
Angular wrapper for Chargebee Components
For detailed examples: Click here
View live demo here
Install from npm:
npm install @chargebee/chargebee-js-angular-wrapper
Chargebee Components requires you to initialize chargebee js with site
and publishableKey
Wondering where to obtain your publishable API key? Refer here
In your index.html
:
<html>
<head>
...
<script src="https://js.chargebee.com/v2/chargebee.js"></script>
</head>
<body>
<div id="app"></div>
</body>
</html>
In your angular component
component.html
<div class="cell example example3" id="example-3" style="padding: 1em">
<form>
<div cbCardField id="card-field" (ready)="onReady($event)"></div>
<button (click)="onTokenize($event)">Submit</button>
</form>
</div>
component.ts
import { Component } from '@angular/core';
declare var Chargebee;
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
standalone: true,
imports: [
RouterOutlet,
CardFieldDirective,
CvvFieldDirective,
NumberFieldDirective,
ExpiryFieldDirective,
],
})
export class AppComponent {
cardComponent = null;
constructor(...) {
...
Chargebee.init({
site: 'your-site'
publishableKey: 'your-publishable-key'
})
}
onReady = (cardComponent) => {
this.cardComponent = cardComponent;
}
onTokenize = (event) => {
event.preventDefault();
this.cardComponent.tokenize().then(data => {
console.log('chargebee token', data.token)
});
}
}
component.html
<div class="cell example example3" id="example-3" style="padding: 1em">
<form>
<div cbCardField id="card-field" [fonts]="fonts" [styles]="styles" locale="en" [showTestCards]="true" [classes]="classes" (ready)="onReady($event)">
<div id="card-number" cbNumberField class="field empty" placeholder="4111 1111 1111 1111" (ready)="setFocus($event)" (change)="onChange($event)"></div>
<div id="card-expiry" cbExpiryField class="field empty" placeholder="MM / YY" (change)="onChange($event)"></div>
<div id="card-cvv" cbCvvField class="field empty" placeholder="CVV" (change)="onChange($event)"></div>
</div>
<div id="errors">{{errorMessage}}</div>
<button id="submit-button" (click)="onSubmit($event)">Pay 25$</button>
</form>
</div>
component.ts
import { Component, ChangeDetectorRef, HostListener } from "@angular/core";
declare var Chargebee;
@Component({
selector: "app-root",
templateUrl: "./app.component.html",
styleUrls: ["./app.component.css"],
standalone: true,
imports: [
RouterOutlet,
CardFieldDirective,
CvvFieldDirective,
NumberFieldDirective,
ExpiryFieldDirective,
],
})
export class AppComponent {
errorMessage = "";
changeDetectorRef: ChangeDetectorRef;
cardComponent = null;
constructor(changeDetectorRef: ChangeDetectorRef) {
this.changeDetectorRef = changeDetectorRef;
}
errors = {};
classes = {
focus: "focus",
complete: "complete-custom-class",
invalid: "invalid",
empty: "empty",
};
fonts = ["https://fonts.googleapis.com/css?family=Open+Sans"];
styles = {
base: {
color: "#fff",
fontWeight: 600,
fontFamily: "Quicksand, Open Sans, Segoe UI, sans-serif",
fontSize: "16px",
fontSmoothing: "antialiased",
":focus": {
color: "#424770",
},
"::placeholder": {
color: "#9BACC8",
},
":focus::placeholder": {
color: "#CFD7DF",
},
},
invalid: {
color: "#fff",
":focus": {
color: "#FA755A",
},
"::placeholder": {
color: "#FFCCA5",
},
},
};
onReady = (cardComponent) => {
this.cardComponent = cardComponent;
};
setFocus(field) {
field.focus();
}
onChange = (status) => {
let errors = {
...this.errors,
[status.field]: status.error,
};
this.errors = errors;
let { message } =
Object.values(errors)
.filter((message) => !!message)
.pop() || {};
this.errorMessage = message;
this.changeDetectorRef.detectChanges();
};
onSubmit = (event) => {
event.preventDefault();
this.cardComponent.tokenize().then((data) => {
console.log("chargebee token", data.token);
});
};
}
In your angular component
component.html
<div class="cell example example3" id="example-3" style="padding: 1em">
<form>
<div cbCardField id="card-field" (ready)="onReady($event)"></div>
<button (click)="authorize($event)">Submit</button>
</form>
</div>
component.ts
import { Component } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css'],
standalone: true,
imports: [
RouterOutlet,
CardFieldDirective,
CvvFieldDirective,
NumberFieldDirective,
ExpiryFieldDirective,
],
})
export class AppComponent {
cardComponent = null;
intent = null;
additionalData = {
// Additional data to improve the chances of frictionless flow
}
onReady = (cardComponent) => {
this.cardComponent = cardComponent;
}
createPaymentIntent() {
// make ajax call to your server to create a paymentIntent
...
this.intent = paymentIntent
}
authorize = (event) => {
event.preventDefault();
this.cardComponent.authorizeWith3ds(this.intent, this.additionalData).then(authorizedIntent => {
console.log('3DS Authorization success', authorizedIntent.id)
});
}
}
Attributes | Description | Datatype |
---|---|---|
fonts | An array of font faces or links | Fonts |
classes | Set of CSS classnames that get substituted for various events | Classes |
locale | Language code | Locale |
showTestCards | Add ability to show test cards on test sites | Boolean |
styles | Set of style customizations | Styles |
placeholder | Set of placeholders for the card fields | Placeholder |
Props | Description | Arguments |
---|---|---|
(ready) | Triggers when component is mounted and ready | Field |
(change) | Triggers for every state change | Field State |
(focus) | Triggers when component is focused | Field State |
(blur) | Triggers when component is blurred | Field State |
Props | Description | Datatype |
---|---|---|
styles | Styles for inidividual field | Styles |
placeholder | Placeholder for the field | String |
Props | Description | Arguments |
---|---|---|
(ready) | Triggers when component is mounted and ready | Field |
(change) | Triggers for every state change | Field State |
(focus) | Triggers when component is focused | Field State |
(blur) | Triggers when component is blurred | Field State |
Chargebee Components - JS Docs
Have any queries regarding the implementation? Reach out to support@chargebee.com
FAQs
Angular wrapper for Chargebee.js Components
The npm package @chargebee/chargebee-js-angular-wrapper receives a total of 1,401 weekly downloads. As such, @chargebee/chargebee-js-angular-wrapper popularity was classified as popular.
We found that @chargebee/chargebee-js-angular-wrapper demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.