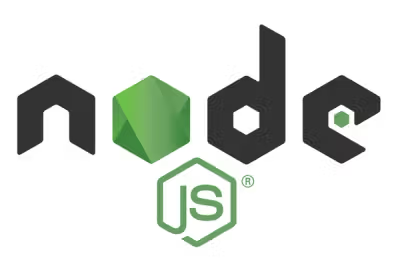
Security News
require(esm) Backported to Node.js 20, Paving the Way for ESM-Only Packages
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
@charged-particles/charged-js-sdk
Advanced tools
Charged Particles Javascript SDK v0.1.8 (beta)
params
ChargedConstructor Charged parameter object.const charged = new Charged({providers: window.ethereum});
const allStateAddresses = await charged.utils.getStateAddress();
const polygonProvider = [
{
network: 137,
service: {alchemy: process.env.ALCHEMY_POLYGON_KEY}
}
];
const charged = new Charged({providers: polygonProvider})
Charged class constructor object parameter.
Type: Object
providers
(Array<NetworkProvider> | providers.Provider | providers.ExternalProvider)? Provider for connection to the Ethereum network.signer
Signer? Needed to send signed transactions to the Ethereum Network to execute state changing operations.config
ConfigurationParametersconst charged = new Charged({providers: window.ethereum});
const nft = charged.NFT( '0xd1bce91a13089b1f3178487ab8d0d2ae191c1963', 43);
const creatorAnnuities = await nft.getCreatorAnnuities();
Returns NftService Instance of the NFT connected to the charged particle protocol
Gets the amount of asset tokens that have been deposited into the Particle.
walletManagerId
ManagerId The ID of the wallet manager to check.assetToken
string The address of the asset token to check.Returns BigNumber The Amount of underlying assets held within the token.
Gets the amount of interest that the particle has generated.
walletManagerId
ManagerId The ID of the Wallet Manager.assetToken
string The address of the asset Token to check.Returns BigNumber The amount of interest generated.
Gets the amount of LP Tokens that the Particle has generated.
walletManagerId
ManagerId The ID of the Wallet Manager.assetToken
string The Address of the Asset Token to check.Returns BigNumber The amount of LP tokens that have been generated.
Gets the total amount of ERC721 tokens that the Particle holds.
basketManagerId
string The ID of the BasketManager to check.Returns BigNumber The total amount of ERC721 tokens that are held within the Particle.
Gets the amount of creator annuities reserved for the creator for the specified NFT.
Returns address The address of the creator.
Returns number The percentage amount of annuities reserved for the creator.
Get the address that receives creator annuities for a given Particle/ Defaults to creator address if it has not been redirected.
Returns address The address of the creator.
Returns number The percentage amount of annuities reserved for the creator.
Gets the tokenUri using the tokenId and contractAddress of the Particle.
Returns string Token metadata URI.
Gets the Discharge timelock state of the Particle.
sender
string The address approved for Discharging assets from the Particle.Returns [boolean, boolean, BigNumber, BigNumber] [allowFromAll, isApproved, timelock, empLockExpiry]
Gets the Discharge timelock state of the Particle.
sender
string The address approved for Releasing assets from the Particle.Returns [boolean, boolean, BigNumber, BigNumber] [allowFromAll, isApproved, timelock, empLockExpiry]
Gets the Bonds Timelock state of the Particle.
sender
string The address approved for removing Bond assets from the Particle.Returns boolean allowFromAll
Returns boolean isApproved
Returns BigNumber timelock
Returns BigNumber empLockExpiry
Fund particle with asset token Must be called by the account providing the asset. Account must also approve THIS contract as operator as asset.
If you are getting gas limit errors this may be because you forgot to approve the contract as operator of asset
walletManagerId
ManagerId The Asset-Pair to Energize the Token withassetToken
string The Address of the Asset Token being usedassetAmount
BigNumberish The Amount of Asset Token to Energize the Token withchainId
number? Optional parameter that allows for the user to specify which network to write toreferrer
string?// Asset amount expects a big numberish type. If you do not supply a
// big number object, ethers will assume you are working in wei.
// Deposits 20 USDC tokens into our particle that will accrue interest.
const USDCoinAddress = '0xUSDC';
const result = await nft.energize(
'aave.B',
USDCoinAddress,
ethers.utils.parseUnits("20", 6),
);
// Or, deposit assets that will not accrue interest
// or assets that are not supported by our yield generating protocols (e.g. aave)
// For example, we will energize our particle with 20 monkey coins
// This will not generate interest.
const monkeyCoinAddress = '0xMONKEY';
const result = await nft.energize(
'generic.B',
monkeyCoinAddress,
ethers.utils.parseUnits("20")
);
Returns Promise<ContractTransaction> A contract receipt from the transaction.Solidity Contract Method
Allows the owner or operator of the token to collect or transfer the interest generated from the token without removing the underlying asset that is held within the token.
receiver
string The address to receive the discharged asset tokens.walletManagerId
ManagerId The wallet manager of that assets to discharge from the token.assetToken
string The address of the asset token being discharged.chainId
number? Optional parameter that allows for the user to specify which network to write to.jconst myWallet = '0xWALLET';
const rocketPoolAddress = '0xRPL';
const result = await nft.discharge(
myWallet,
'aave.B',
rocketPoolAddress,
);
// You can also discharge to any arbitrary wallet!
// Let's send all of our interest accrued by our DAI to Vitalik.
const vitaliksWallet = '0xCOOLGUY';
const daiAddress = '0xDAI';
const result = await nft.discharge(
vitaliksWallet,
'aave.B',
daiAddress,
)
Returns Promise<ContractTransaction> A receipt from the contract transaction.Solidity Contract Method
Allows the owner or operator of the Token to collect or transfer a specific amount of the interest generated from the token without removing the underlying Asset that is held within the token.
receiver
string The address to receive the discharged asset tokens.walletManagerId
ManagerId The wallet manager of the assets to discharge from the token.assetToken
string The address of the asset token being discharged.assetAmount
BigNumberish The specific amount of asset token to discharge from the particle.chainId
number? Optional parameter that allows for the user to specify which network to write to.Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Allows the Creator of the Token to collect or transfer a their portion of the interest (if any) generated from the token without removing the underlying Asset that is held within the token.
receiver
string The address to receive the discharged asset tokenswalletManagerId
ManagerId The wallet manager of the assets to discharge from the tokenassetToken
string The address of the asset token being dischargedassetAmount
BigNumberish The specific amount of asset token to discharge from the particlechainId
number? Optional parameter that allows for the user to specify which network to write toReturns Promise<ContractTransaction> A receipt from the transactionSolidity Contract Method
Releases the full amount of asset + interest held within the particle by LP of the assets. To release NFT assets from your particle, see break bond.
receiver
string The address to receive the released asset tokens.walletManagerId
ManagerId The wallet manager of the assets to release from the token.assetToken
string The address of the asset token being released.chainId
number? Optional parameter that allows for the user to specify which network to write to.// Release the DAI from our particle. Withdraws the interest (if any) as well!
const receiver = '0xMYWALLET';
const daiAddress = '0xDAI';
const result = nft.release(
receiver,
'aave.B',
daiAddress,
);
Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Releases a partial amount of asset + interest held within the particle by LP of the assets.
receiver
string The address to receive the released asset tokenswalletManagerId
ManagerId The wallet manager of the assets to release from the tokenassetToken
string The address of the asset token being releasedassetAmount
BigNumberish The specific amount of asset token to release from the particlechainId
number? Optional parameter that allows for the user to specify which network to write toReturns Promise<ContractTransaction> A receipt from the transactionSolidity Contract Method
Deposit other NFT assets into the particle. Must be called by the account providing the asset. Account must approve THIS contract as operator of asset.
basketManagerId
string The basket to deposit the NFT into.nftTokenAddress
string The address of the NFT token being deposited.nftTokenId
string The ID of the NFT token being deposited.nftTokenAmount
number The amount of tokens to deposit (ERC1155-specific).chainId
number? Optional parameter that allows for the user to specify which network to write to.const nftTokenAddress = '0xMOONBIRDS';
const tokenId = '12';
const result = await nft.bond(
'generic.B',
nftTokenAddress,
tokenId,
1,
);
// We have 12 erc-1155 nfts that we want to bond to the particle.
const nftTokenAddress = '0xCOOLGAME';
const tokenId = '78';
const result = await nft.bond(
'generic.B',
nftTokenAddress,
tokenId,
12
);
Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Release NFT assets from the particle.
receiver
string The address to receive the released asset tokens.basketManagerId
string The basket to release the NFT from.nftTokenAddress
string The address of the NFT token being released.nftTokenId
string The ID of the NFT token being released.nftTokenAmount
Number The amount of tokens to deposit (ERC1155-specific).chainId
number? Optional parameter that allows for the user to specify which network to write to.// We bonded 14 erc-1155 nfts to our particle. We want to release 3.
// When working with erc-721 use 1 for nftTokenAmount.
const receiver = '0xMYWALLET';
const nftTokenAddress = '0xNFTS';
const tokenId = '35';
const result = await nft.breakBond(
receiver,
'generic.B',
nftTokenAddress,
tokenId,
3
);
Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Sets a timelock on the ability to release the assets of a particle.
unlockBlock
number The Ethereum block number to timelock until (~15 seconds per block).chainId
number? Optional parameter that allows for the user to specify which network to write to.Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Sets a timelock on the ability to discharge the assets of a particle
unlockBlock
number The Ethereum block number to timelock until (~15 seconds per block).chainId
number? Optional parameter that allows for the user to specify which network to write to.Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Sets a timelock on the ability to break the covalent bond of a particle
unlockBlock
number The Ethereum block number to timelock until (~15 seconds per block).chainId
number? Optional parameter that allows for the user to specify which network to write to.Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Sets the custom configuration for creators of proton-based NFTs Must be called by account that created and owns the particle
creator
string The creator's address of the proton-based NFT.annuityPercent
BigNumberish The percentage of interest-annuities to reserve for the creator. In decimal this can range from 0 - 10000. 5712 would be 57.12%..chainId
number? Optional parameter that allows for the user to specify which network to write to.Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
Sets a custom receiver address for the creator annuities Must be called by account that created and owns the particle
receiver
string The receiver of the creator interest annuities.chainId
number? Optional parameter that allows for the user to specify which network to write to.Returns Promise<ContractTransaction> Details from the transaction.Solidity Contract Method
const charged = new Charged({providers: window.ethereum});
const creatorAnnuities = await charged.utils.getStateAddress();
Returns UtilsService
Get the address of the chargedState contract.
Returns string state contract address
Get the address of the chargedSettings contract.
Returns string settings contract address
Get the address of the chargedManagers contract.
Returns string manager contract address
User specified custom network provider.
Type: Object
network
number? Configure the SDK.const providers = [
{
network: 1,
service: { 'alchemy': process.env.ALCHEMY_MAINNET_KEY }
},
{
network: 42,
service: { 'infura': process.env.INFURA_KOVAN_KEY }
}
];
const rpcUrlProvider = [
{
network: 42,
service: { 'rpc': `https://kovan.infura.io/v3/${process.env.INFURA_PROJECT_SECRET}`}
}
];
A string enum that identifies which wallet manager to use. Used in functions like release
and discharge
Type: ManagerId
ManagerId
string possible values: aave
, aave.B
, generic
, generic.B
Charged class constructor object parameter.
Type: Object
sdk
SdkConfiguration? Configure the SDK.transactionOverride
TransactionOverride? Override transaction default values.SDK configuration.
Type: Object
NftBridgeCheck
boolean? Verifies that the signer network matches the chainId of the contract interaction.Overrides ethers transaction default parameters.
Type: Object
Get the deposit fee of the protocol.
Returns string protocol fee amount.
FAQs
Charged Particles - Javascript SDK
The npm package @charged-particles/charged-js-sdk receives a total of 11 weekly downloads. As such, @charged-particles/charged-js-sdk popularity was classified as not popular.
We found that @charged-particles/charged-js-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
require(esm) backported to Node.js 20, easing the transition to ESM-only packages and reducing complexity for developers as Node 18 nears end-of-life.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.