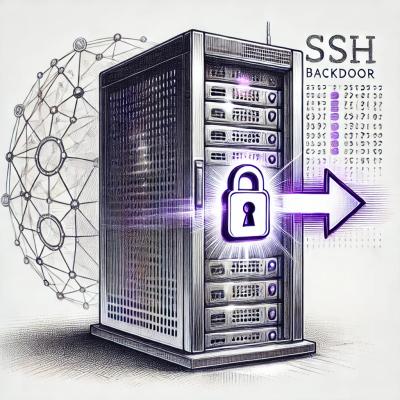
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@cocreators-ee/openapi-typescript-fetch-svelte
Advanced tools
A typed fetch client for openapi-typescript with Svelte support
A typed fetch client for openapi-typescript compatible with SvelteKit's custom fetch
npm install @cocreators-ee/openapi-typescript-fetch-svelte
Or
pnpm add @cocreators-ee/openapi-typescript-fetch-svelte
Features
Supports JSON request and responses
npx openapi-typescript https://petstore.swagger.io/v2/swagger.json --output petstore.ts
# 🔭 Loading spec from https://petstore.swagger.io/v2/swagger.json…
# 🚀 https://petstore.swagger.io/v2/swagger.json -> petstore.ts [650ms]
Configure SvelteFetcher instance and generate functions for making API calls:
// File: api.ts
import { SvelteFetcher } from '@cocreators-ee/openapi-typescript-fetch-svelte'
import { paths } from './petstore'
// declare fetcher for paths
const fetcher = Fetcher.for<paths>()
// global configuration
fetcher.configure({
// Base URL to your API, e.g. `/` if you're serving API from the same domain
baseUrl: 'https://petstore.swagger.io/v2',
// RequestInit options, e.g. default headers
init: {
// mode: 'cors'
// headers: {}
},
})
// create fetch operations
export const findPetsByStatus = fetcher
.path('/pet/findByStatus')
.method('get')
.create()
export const addPet = fetcher.path('/pet').method('post').create()
Each API call is represented as a request object that has the following properties:
{
// Svelte store that contains a promise for an API call.
// If you reload the requets using reload() function, this store will be updated
ready,
// Promise for the initial API call. Will not be updated by `reload()` function.
// Usefull for server code and places where you can't use the `ready` store.
isLoaded,
// Svelte store containing the response of the API call.
resp,
// Function that reloads the request with the same parameteres
reload,
}
Each response is a Svelte store returning either an undefined
, or the following object:
{
// HTTP status code
status,
// Boolean, whether the request was successful or not
ok,
// Typed object for a 200/201 status. Built from the OpenAPI spec
data
}
<script lang="ts">
import { findPetByStatus } from './api.ts'
const request = findPetByStatus({ status: 'sold' })
const petsReady = request.ready
</script>
<div>
{#await $petsReady}
<p>Loading..</p>
{:then resp}
{#if resp.ok}
{#each resp.data as pet}
<p>{pet.name}</p>
{/each}
{:else}
<p>Error while loading pets</p>
{/if}
{/await}
<button on:click={() => {request.reload()}}>
Reload pets
</button>
</div>
<script lang="ts">
import { findPetByStatus } from './api.ts'
const request = findPetByStatus({ status: 'sold' })
let names = []
request.resp.subscribe(resp => {
if (resp.ok) {
names = resp.data.map(pet => pet.name)
}
})
</script>
<div>
{#each resp.data as pet}
<p>{pet.name}</p>
{/each}
</div>
Fetch operations support SvelteKit's load function from +page.ts
and +page.server.ts
:
export async function load({ fetch }) {
const request = findPetByStatus({ status: 'sold' })
const resp = await request.isLoaded
if (resp.ok) {
return { pets: resp.data }
} else {
return { pets: [] }
}
}
If you work on non-Svelte project, then you can use Fetcher
instead:
import { Fetcher } from 'openapi-typescript-fetch'
import { paths } from './petstore'
// declare fetcher for paths
const fetcher = Fetcher.for<paths>()
// global configuration
fetcher.configure({
baseUrl: 'https://petstore.swagger.io/v2',
})
// create fetch operations
const findPetsByStatus = fetcher
.path('/pet/findByStatus')
.method('get')
.create()
const addPet = fetcher.path('/pet').method('post').create()
const resp = await findPetsByStatus({ status: 'available' })
console.log(resp.ok)
console.log(resp.data)
console.log(resp.status)
A non-ok fetch response throws a generic ApiError
But an Openapi document can declare a different response type for each status code, or a default error response type
These can be accessed via a discriminated union
on status, as in code snippet below
const findPetsByStatus = fetcher.path('/pet/findByStatus').method('get').create()
const addPet = fetcher.path('/pet').method('post').create()
try {
await findPetsByStatus({ ... })
await addPet({ ... })
} catch(e) {
// check which operation threw the exception
if (e instanceof addPet.Error) {
// get discriminated union { status, data }
const error = e.getActualType()
if (error.status === 400) {
error.data.validationErrors // only available for a 400 response
} else if (error.status === 500) {
error.data.errorMessage // only available for a 500 response
} else {
...
}
}
}
OpArgType
- Infer argument type of an operationOpReturnType
- Infer return type of an operationOpErrorType
- Infer error type of an operationFetchArgType
- Argument type of a typed fetch operationFetchReturnType
- Return type of a typed fetch operationFetchErrorType
- Error type of a typed fetch operationTypedFetch
- Fetch operation typeimport { paths, operations } from './petstore'
type Arg = OpArgType<operations['findPetsByStatus']>
type Ret = OpReturnType<operations['findPetsByStatus']>
type Err = OpErrorType<operations['findPetsByStatus']>
type Arg = OpArgType<paths['/pet/findByStatus']['get']>
type Ret = OpReturnType<paths['/pet/findByStatus']['get']>
type Err = OpErrorType<paths['/pet/findByStatus']['get']>
type FindPetsByStatus = TypedFetch<operations['findPetsByStatus']>
const findPetsByStatus = fetcher
.path('/pet/findByStatus')
.method('get')
.create()
type Arg = FetchArgType<typeof findPetsByStatus>
type Ret = FetchReturnType<typeof findPetsByStatus>
type Err = FetchErrorType<typeof findPetsByStatus>
arrayRequestBody
- Helper to merge params when request body is an array see issueconst body = arrayRequestBody([{ item: 1 }], { param: 2 })
// body type is { item: number }[] & { param: number }
Happy fetching! 👍
FAQs
A typed fetch client for openapi-typescript with Svelte support
The npm package @cocreators-ee/openapi-typescript-fetch-svelte receives a total of 0 weekly downloads. As such, @cocreators-ee/openapi-typescript-fetch-svelte popularity was classified as not popular.
We found that @cocreators-ee/openapi-typescript-fetch-svelte demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.