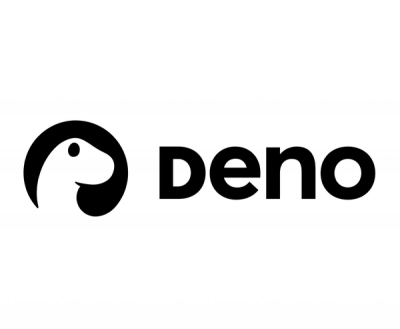
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@codemirror/lang-css
Advanced tools
@codemirror/lang-css is a language support package for CodeMirror 6 that provides syntax highlighting, code folding, and other editing features for CSS. It is designed to be used with the CodeMirror 6 editor, which is a versatile text editor implemented in JavaScript for the browser.
Syntax Highlighting
This code sets up a CodeMirror editor with basic syntax highlighting for CSS. The `css` function from `@codemirror/lang-css` is used to provide the CSS language support.
import { css } from '@codemirror/lang-css';
import { EditorState } from '@codemirror/state';
import { EditorView, basicSetup } from '@codemirror/basic-setup';
const state = EditorState.create({
doc: 'body {\n background-color: #f0f0f0;\n}',
extensions: [basicSetup, css()]
});
const view = new EditorView({
state,
parent: document.body
});
Code Folding
This code sets up a CodeMirror editor with code folding capabilities for CSS. The `foldGutter` extension is used alongside the `css` function to enable folding of CSS code blocks.
import { css } from '@codemirror/lang-css';
import { foldGutter } from '@codemirror/fold';
import { EditorState } from '@codemirror/state';
import { EditorView, basicSetup } from '@codemirror/basic-setup';
const state = EditorState.create({
doc: 'body {\n background-color: #f0f0f0;\n color: #333;\n}',
extensions: [basicSetup, css(), foldGutter()]
});
const view = new EditorView({
state,
parent: document.body
});
Linting
This code sets up a CodeMirror editor with linting capabilities for CSS. The `linter` and `lintGutter` extensions are used alongside the `css` function to provide linting support.
import { css } from '@codemirror/lang-css';
import { linter, lintGutter } from '@codemirror/lint';
import { EditorState } from '@codemirror/state';
import { EditorView, basicSetup } from '@codemirror/basic-setup';
const cssLinter = linter(view => {
// Custom linting logic
return [];
});
const state = EditorState.create({
doc: 'body {\n background-color: #f0f0f0;\n color: #333;\n}',
extensions: [basicSetup, css(), lintGutter(), cssLinter]
});
const view = new EditorView({
state,
parent: document.body
});
The `monaco-css` package provides CSS language support for the Monaco Editor, which is the editor that powers Visual Studio Code. It offers advanced features like syntax highlighting, code folding, and linting, similar to `@codemirror/lang-css` but is tailored for the Monaco Editor.
The `ace-builds` package includes CSS language support for the Ace Editor. It provides features like syntax highlighting and code folding for CSS, similar to `@codemirror/lang-css`, but is designed for the Ace Editor.
[ WEBSITE | ISSUES | FORUM | CHANGELOG ]
This package implements CSS language support for the CodeMirror code editor.
The project page has more information, a number of examples and the documentation.
This code is released under an MIT license.
We aim to be an inclusive, welcoming community. To make that explicit, we have a code of conduct that applies to communication around the project.
css() → LanguageSupport
Language support for CSS.
cssLanguage: LRLanguage
A language provider based on the Lezer CSS parser, extended with highlighting and indentation information.
cssCompletionSource: CompletionSource
CSS property and value keyword completion source.
6.1.1 (2023-03-08)
Provide better completions when completing directly in a Styles
top node.
FAQs
CSS language support for the CodeMirror code editor
The npm package @codemirror/lang-css receives a total of 562,506 weekly downloads. As such, @codemirror/lang-css popularity was classified as popular.
We found that @codemirror/lang-css demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.