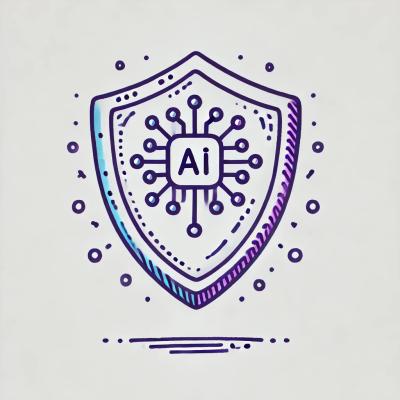
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@coderspirit/nominal
Advanced tools
This library provides some powerful types to implement nominal typing and values tainting with zero runtime overhead. This can be very useful to enforce complex pre-conditions and post-conditions, and to make your code more secure against malicious inputs.
npm install @coderspirit/nominal
or
yarn install @coderspirit/nominal
To define a new nominal type based on a previous type, we can do:
import { WithTag } from '@coderspirit/nominal'
type Email = WithTag<string, 'Email'>
const email: Email = 'coyote@ac.me' as Email
WithTag
has been implemented in a way that allows us to easily compose many
nominal types for a same value:
import { WithTag, WithTags } from '@coderspirit/nominal'
type Integer = WithTag<number, 'Integer'>
type Even = WithTag<number, 'Even'>
type Positive = WithTag<number, 'Positive'>
// The first way is by adding "tags" to an already "tagged" type
type EvenInteger = WithTag<Integer, 'Even'>
// The second way is by adding many "tags" at the same time
type EvenPositive = WithTags<number, ['Even', 'Positive']>
WithTag
and WithTags
are additive, commutative and idempotent.This feature can be very useful when we need to verify many properties for the same value and we don't want to lose this information along the way as the value is passed from one function to another.
function throwIfNotEven<T extends number>(v: T): WithTag<T, 'Even'> {
if (v % 2 == 1) throw new Error('Not Even!')
return v as WithTag<T, 'Even'>
}
function throwIfNotPositive<T extends number>(v: T): WithTag<T, 'Positive'> {
if (v <= 0) throw new Error('Not positive!')
return v as WithTag<T, 'Positive'>
}
const v1 = 42
// typeof v2 === WithTag<number, 'Even'>
const v2 = throwIfNotEven(v1)
// typeof v3 === WithTags<number, ['Even', 'Positive']>
const v3 = throwIfNotPositive(v2)
If needed, we can easily remove "tags" from our types:
import { WithTag, WithoutTag } from '@coderspirit/nominal'
type Email = WithTag<string, 'Email'>
type NotEmail = WithoutTag<string, 'Email'>
type NotAnEmailAnymore = WithoutTag<Email, 'Email'>
const email: Email = 'coyote@ac.me' as Email
// The type checked accepts this
const untypedEmail: string = email
// The type checker will error with any of the following two lines
const notEmail1: NotEmail = email
const notEmail2: NotAnEmailAnymore = email
// NotEmail & NotAnEmailAnymore are still compatible with string
const notEmail3: NotEmail = 'not an email'
const notEmail4: string = notEmail3 // This is OK :)
const notEmail5: NotAnEmailAnymore = 'not an email anymore'
const notEmail6: string = notEmail5 // This is also OK :)
This can be a powerful building block to implement values tainting, although we already provide an out-of-box solution for that.
While using nominal types in the typical way is often enough, sometimes it can be handy to mark all the values coming from the "external world" as tainted (and therfore "dangerous"), independently of whether we took the time to assign them a specific nominal type or not.
@coderspirit/nominal
provides the types Tainted<T>
and Untainted<T>
, both
of them operate recursively on T
.
import { Tainted, Untainted } from '@coderspirit/nominal'
interface LoginRequest {
username: string
password: string
}
type TaintedLoginRequest = Tainted<LoginRequest>
type UntaintedLoginRequest = Untainted<LoginRequest>
function validateLoginRequest(req: TaintedLoginRequest): UntaintedLoginRequest {
// throw Error if `req` is invalid
return req as UntaintedLoginRequest
}
// This function accepts LoginRequest and UntaintedLoginRequest,
// but not TaintedLoginRequest
function login(req: UntaintedLoginRequest): void {
// Do stuff
}
// When req is tainted, we cannot pass req.password to this function, as all
// req's fields are tainted as well.
function doStuffWithPassword(password: Untainted<string>): void {
// Do stuff
}
While this specific use case is far from being exciting and quite simplistic, this idea can be applied to much more sensitive and convoluted scenarios.
If you really want to use the tainting feature, it's better to rely on these two
types, rather than trying to do it with WithTag
and WithoutTags
, as there
are some complicated details to take into account, like nested tainting.
We also have a handy shortcut to combine (un)tainting with other nominal types:
import { Safe, Tainted } from '@coderspirit/nominal'
// Compared with WithTag, it imposes slightly stricter conditions when used in
// function signatures.
type Email = Safe<string, 'Email'>
type InsecureString = Tainted<string>
// It can be applied to "tainted types" too.
type PostalCode = Safe<InsecureString, 'PostalCode'>
While it's difficult to imagine how a value might be tainted in multiple ways, it's not unheard of. This could be useful when managing sensitive information, if we need/want to statically enforce that some data won't cross certain boundaries.
import { GenericTainted, GenericUntainted, GenericSafe } from '@coderspirit/nominal'
type BlueTaintedNumber = GenericTainted<number, 'Blue'>
type RedTaintedNumber = GenericTainted<number, 'Red'>
// Double-tainted type!
type BlueRedTaintedNumber = GenericTainted<BlueTaintedNumber, 'Red'>
// We removed again the 'Blue' taint
type OnlyBlueTaintedNumber = GenericUntainted<BlueRedTaintedNumber, 'Red'>
// We removed the 'Red' taint, and added a "type tag"
type SafeOnBlueZoneNumber = GenericSafe<BlueRedTaintedNumber, 'SafeOnBlue', 'Blue'>
FAQs
Powerful nominal types for your project
The npm package @coderspirit/nominal receives a total of 0 weekly downloads. As such, @coderspirit/nominal popularity was classified as not popular.
We found that @coderspirit/nominal demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.