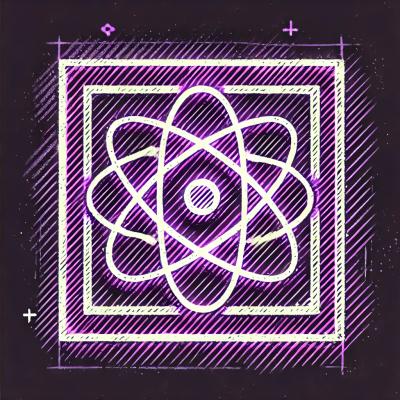
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@coinbase/agentkit
Advanced tools
AgentKit is a framework for easily enabling AI agents to take actions onchain. It is designed to be framework-agnostic, so you can use it with any AI framework, and wallet-agnostic, so you can use it with any wallet.
Prerequisites:
npm install @coinbase/agentkit
CdpWalletProvider
and WalletProvider
action provider.const agentKit = await AgentKit.from({
cdpApiKeyName: "CDP API KEY NAME",
cdpApiKeyPrivate: "CDP API KEY PRIVATE KEY",
});
import { CdpWalletProvider } from "@coinbase/agentkit";
const walletProvider = await CdpWalletProvider.configureWithWallet({
apiKeyName: "CDP API KEY NAME",
apiKeyPrivate: "CDP API KEY PRIVATE KEY",
networkId: "base-mainnet",
});
const agentKit = await AgentKit.from({
walletProvider,
});
import { cdpApiActionProvider, pythActionProvider } from "@coinbase/agentkit";
const agentKit = await AgentKit.from({
walletProvider,
actionProviders: [
cdpApiActionProvider({
apiKeyName: "CDP API KEY NAME",
apiKeyPrivate: "CDP API KEY PRIVATE KEY",
}),
pythActionProvider(),
],
});
Prerequisites:
OPENAI_API_KEY
environment variable.npm install @langchain @langchain/langgraph @langchain/openai
import { getLangChainTools } from "@coinbase/agentkit-langchain";
import { createReactAgent } from "@langchain/langgraph/prebuilt";
import { ChatOpenAI } from "@langchain/openai";
const tools = await getLangChainTools(agentKit);
const llm = new ChatOpenAI({
model: "gpt-4o-mini",
});
const agent = createReactAgent({
llm,
tools,
});
Action providers are used to define the actions that an agent can take. They are defined as a class that extends the ActionProvider
abstract class.
import { ActionProvider, WalletProvider, Network } from "@coinbase/agentkit";
// Define an action provider that uses a wallet provider.
class MyActionProvider extends ActionProvider<WalletProvider> {
constructor() {
super("my-action-provider", []);
}
// Define if the action provider supports the given network
supportsNetwork = (network: Network) => true;
}
Actions are defined as instance methods on the action provider class with the @CreateAction
decorator. Actions can use a wallet provider or not and always return a Promise that resolves to a string.
Creating actions with the @CreateAction
decorator requires the following compilerOptions to be included in your project's tsconfig.json
.
{
"compilerOptions": {
"experimentalDecorators": true,
"emitDecoratorMetadata": true
}
}
zod
library.import { z } from "zod";
export const MyActionSchema = z.object({
myField: z.string(),
});
import { ActionProvider, WalletProvider, Network, CreateAction } from "@coinbase/agentkit";
class MyActionProvider extends ActionProvider<WalletProvider> {
constructor() {
super("my-action-provider", []);
}
@CreateAction({
name: "my-action",
description: "My action description",
schema: MyActionSchema,
})
async myAction(args: z.infer<typeof MyActionSchema>): Promise<string> {
return args.myField;
}
supportsNetwork = (network: Network) => true;
}
export const myActionProvider = () => new MyActionProvider();
Actions that use a wallet provider can be defined as instance methods on the action provider class with the @CreateAction
decorator that have a WalletProvider
as the first parameter.
class MyActionProvider extends ActionProvider<WalletProvider> {
constructor() {
super("my-action-provider", []);
}
@CreateAction({
name: "my-action",
description: "My action description",
schema: MyActionSchema,
})
async myAction(walletProvider: WalletProvider, args: z.infer<typeof MyActionSchema>): Promise<string> {
return walletProvider.signMessage(args.myField);
}
supportsNetwork = (network: Network) => true;
}
This gives your agent access to the actions defined in the action provider.
const agentKit = new AgentKit({
cdpApiKeyName: "CDP API KEY NAME",
cdpApiKeyPrivate: "CDP API KEY PRIVATE KEY",
actionProviders: [myActionProvider()],
});
Wallet providers give an agent access to a wallet. AgentKit currently supports the following wallet providers:
EVM:
The CdpWalletProvider
is a wallet provider that uses the Coinbase Developer Platform (CDP) API Wallet.
The CdpWalletProvider
can be configured to use a specific network by passing the networkId
parameter to the configureWithWallet
method. The networkId
is the ID of the network you want to use. You can find a list of supported networks on the CDP API docs.
import { CdpWalletProvider } from "@coinbase/agentkit";
const walletProvider = await CdpWalletProvider.configureWithWallet({
apiKeyName: "CDP API KEY NAME",
apiKeyPrivate: "CDP API KEY PRIVATE KEY",
networkId: "base-mainnet",
});
If you already have a CDP API Wallet, you can configure the CdpWalletProvider
by passing the wallet
parameter to the configureWithWallet
method.
import { CdpWalletProvider } from "@coinbase/agentkit";
import { Wallet } from "@coinbase/coinbase-sdk";
const walletProvider = await CdpWalletProvider.configureWithWallet({
wallet,
apiKeyName: "CDP API KEY NAME",
apiKeyPrivate: "CDP API KEY PRIVATE KEY",
});
The CdpWalletProvider
can be configured from a mnemonic phrase by passing the mnemonicPhrase
parameter to the configureWithWallet
method.
import { CdpWalletProvider } from "@coinbase/agentkit";
const walletProvider = await CdpWalletProvider.configureWithWallet({
mnemonicPhrase: "MNEMONIC PHRASE",
});
The CdpWalletProvider
can export a wallet by calling the exportWallet
method.
import { CdpWalletProvider } from "@coinbase/agentkit";
const walletProvider = await CdpWalletProvider.configureWithWallet({
mnemonicPhrase: "MNEMONIC PHRASE",
});
const walletData = await walletProvider.exportWallet();
WalletData
JSON stringThe CdpWalletProvider
can import a wallet from a WalletData
JSON string by passing the cdpWalletData
parameter to the configureWithWallet
method.
import { CdpWalletProvider } from "@coinbase/agentkit";
const walletProvider = await CdpWalletProvider.configureWithWallet({
cdpWalletData: "WALLET DATA JSON STRING",
apiKeyName: "CDP API KEY NAME",
apiKeyPrivate: "CDP API KEY PRIVATE KEY",
});
The ViemWalletProvider
is a wallet provider that uses the Viem library. It is useful for interacting with any EVM-compatible chain.
import { ViemWalletProvider } from "@coinbase/agentkit";
import { privateKeyToAccount } from "viem/accounts";
import { baseSepolia } from "viem/chains";
import { http } from "viem/transports";
import { createWalletClient } from "viem";
const account = privateKeyToAccount(
"0x4c0883a69102937d6231471b5dbb6208ffd70c02a813d7f2da1c54f2e3be9f38",
);
const client = createWalletClient({
account,
chain: baseSepolia,
transport: http(),
});
const walletProvider = new ViemWalletProvider(client);
See CONTRIBUTING.md for more information.
FAQs
Coinbase AgentKit core primitives
The npm package @coinbase/agentkit receives a total of 0 weekly downloads. As such, @coinbase/agentkit popularity was classified as not popular.
We found that @coinbase/agentkit demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.