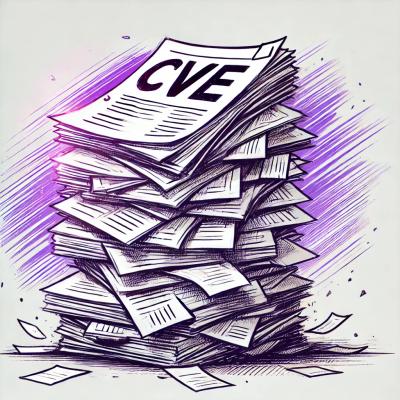
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@commercetools-uikit/creatable-select-input
Advanced tools
An input component getting a selection from the user, and where options can also be created by the user.
An input component getting a selection from the user, and where options can also be created by the user.
yarn add @commercetools-uikit/creatable-select-input
npm --save install @commercetools-uikit/creatable-select-input
Additionally install the peer dependencies (if not present)
yarn add react react-dom react-intl
npm --save install react react-dom react-intl
import PropTypes from 'prop-types';
import CreatableSelectInput from '@commercetools-uikit/creatable-select-input';
const Example = (props) => (
<CreatableSelectInput
name="form-field-name"
value={props.value}
onChange={() => {}}
options={[
{ value: 'one', label: 'One' },
{ value: 'two', label: 'Two' },
]}
/>
);
Example.propTypes = {
value: PropTypes.string,
};
export default Example;
Props | Type | Required | Default | Description |
---|---|---|---|---|
horizontalConstraint | union Possible values: , 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16, 'scale', 'auto' | Horizontal size limit of the input fields. | ||
hasError | boolean | Indicates the input field has an error | ||
hasWarning | boolean | Indicates the input field has a warning | ||
isReadOnly | boolean | Disables the select input as it is read-only | ||
iconLeft | ReactNode | Icon to display on the left of the placeholder text and selected value. Has no effect when isMulti is enabled. | ||
aria-label | CreatableProps['aria-label'] | Aria label (for assistive tech)
Props from React select was used | ||
aria-labelledby | CreatableProps['aria-labelledby'] | HTML ID of an element that should be used as the label (for assistive tech)
Props from React select was used | ||
aria-invalid | CreatableProps['aria-invalid'] | Indicate if the value entered in the input is invalid.
Props from React select was used | ||
aria-errormessage | CreatableProps['aria-errormessage'] | HTML ID of an element containing an error message related to the input.
Props from React select was used | ||
isAutofocussed | boolean | Focus the control when it is mounted | ||
backspaceRemovesValue | CreatableProps['backspaceRemovesValue'] | Remove the currently focused option when the user presses backspace
Props from React select was used | ||
components | CreatableProps['components'] | Map of components to overwrite the default ones, see what components you can override
Props from React select was used | ||
filterOption | CreatableProps['filterOption'] | Custom method to filter whether an option should be displayed in the menu
Props from React select was used | ||
id | CreatableProps['inputId'] | The id of the search input
Props from React select was used | ||
inputValue | CreatableProps['inputValue'] | The value of the search input
Props from React select was used | ||
containerId | CreatableProps['id'] | The id to set on the SelectContainer component
Props from React select was used | ||
isClearable | CreatableProps['isClearable'] | Is the select value clearable
Props from React select was used | ||
isDisabled | CreatableProps['isDisabled'] | Is the select disabled
Props from React select was used | ||
isOptionDisabled | CreatableProps['isOptionDisabled'] | Override the built-in logic to detect whether an option is disabled
Props from React select was used | ||
isMulti | CreatableProps['isMulti'] | Support multiple selected options
Props from React select was used | ||
isSearchable | CreatableProps['isSearchable'] | true | Whether to enable search functionality
Props from React select was used | |
maxMenuHeight | CreatableProps['maxMenuHeight'] | Maximum height of the menu before scrolling
Props from React select was used | ||
menuPortalTarget | CreatableProps['menuPortalTarget'] | Dom element to portal the select menu to
Props from React select was used | ||
menuPortalZIndex | number | 1 | z-index value for the menu portal
Use in conjunction with menuPortalTarget | |
menuShouldBlockScroll | CreatableProps['menuShouldBlockScroll'] | whether the menu should block scroll while open
Props from React select was used | ||
closeMenuOnSelect | CreatableProps['closeMenuOnSelect'] | Whether the menu should close after a value is selected. Defaults to true .
Props from React select was used | ||
name | CreatableProps['name'] | Name of the HTML Input (optional - without this, no input will be rendered)
Props from React select was used | ||
noOptionsMessage | CreatableProps['noOptionsMessage'] | Can be used to render a custom value when there are no options (either because of no search results, or all options have been used, or there were none in the first place). Gets called with { inputValue: String } . inputValue will be an empty string when no search text is present.
Props from React select was used | ||
onBlur | Function See signature. | Handle blur events on the control | ||
onChange | Function See signature. | Called with a fake event when value changes. The event's target.name will be the name supplied in props. The event's target.value will hold the value. The value will be the selected option, or an array of options in case isMulti is true . | ||
onFocus | CreatableProps['onFocus'] | Handle focus events on the control
Props from React select was used | ||
onInputChange | CreatableProps['onInputChange'] | Handle change events on the input
Props from React select was used | ||
options | union Possible values: TValue[] , { options: TValue[] }[] | Array of options that populate the select menu | ||
showOptionGroupDivider | boolean | Determines if option groups will be separated by a divider | ||
placeholder | CreatableProps['placeholder'] | Placeholder text for the select value
Props from React select was used | ||
isCondensed | boolean | Use this property to reduce the paddings of the component for a ui compact variant | ||
tabIndex | CreatableProps['tabIndex'] | Sets the tabIndex attribute on the input
Props from React select was used | ||
tabSelectsValue | CreatableProps['tabSelectsValue'] | Select the currently focused option when the user presses tab
Props from React select was used | ||
value | CreatableProps['value'] | null | The value of the select; reflected by the selected option
Props from React select was used | |
allowCreateWhileLoading | CreatableProps['allowCreateWhileLoading'] | Allow options to be created while the isLoading prop is true. Useful to prevent the "create new ..." option being displayed while async results are still being loaded.
Props from React select was used | ||
formatCreateLabel | CreatableProps['formatCreateLabel'] | Gets the label for the "create new ..." option in the menu. Is given the current input value.
Props from React select was used | ||
isValidNewOption | CreatableProps['isValidNewOption'] | Determines whether the "create new ..." option should be displayed based on the current input value, select value and options array.
Props from React select was used | ||
getNewOptionData | CreatableProps['getNewOptionData'] | Returns the data for the new option when it is created. Used to display the value, and is passed to onChange.
Props from React select was used | ||
onCreateOption | CreatableProps['onCreateOption'] | If provided, this will be called with the input value when a new option is created, and onChange will not be called. Use this when you need more control over what happens when new options are created.
Props from React select was used | ||
createOptionPosition | CreatableProps['createOptionPosition'] | Sets the position of the createOption element in your options list.
Props from React select was used |
onBlur
(event: TCustomEvent) => void
onChange
(event: TCustomEvent, info: ActionMeta<unknown>) => void
This input is built on top of react-select
v2.
It supports mostly same properties as react-select
. Behaviour for some props was changed, and support for others was dropped.
In case you need one of the currently excluded props, feel free to open a PR adding them.
options
The options support a isDisabled
property which will render the option with a disabled style and will prevent users from selecting it.
isTouched(touched)
Returns truthy value for the Formik touched
value of this input field.
It is possible to customize CreatableSelectInput
by passing the components
property.
CreatableSelectInput
exports the default underlying components as static exports.
Components available as static exports are:
ClearIndicator
Control
CrossIcon
DownChevron
DropdownIndicator
Group
GroupHeading
IndicatorsContainer
IndicatorSeparator
Input
LoadingIndicator
LoadingMessage
Menu
MenuList
MenuPortal
MultiValue
MultiValueContainer
MultiValueLabel
MultiValueRemove
NoOptionsMessage
Option
Placeholder
SelectContainer
SingleValue
ValueContainer
See the official documentation for more information about the props they receive.
FAQs
An input component getting a selection from the user, and where options can also be created by the user.
We found that @commercetools-uikit/creatable-select-input demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.