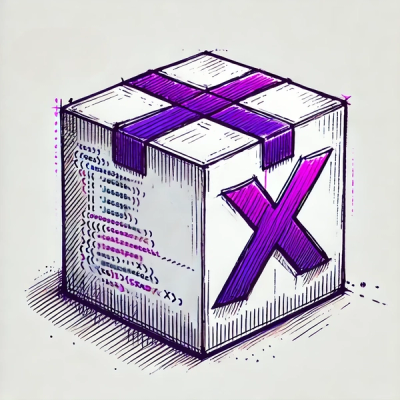
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@cosmograph/react
Advanced tools
User-friendly library for integrating the @cosmograph/cosmograph graph visualization library into your React applications. It provides a collection of ready-to-use components, that make it easy to build interactive network visualizations in your React applications.
Install the package:
npm install @cosmograph/react@beta
Get the data, configure the graph and render Cosmograph:
import React, { useEffect, useState } from 'react'
import { Cosmograph, CosmographConfig, prepareCosmographData } from '@cosmograph/react'
// Points and links data can be:
// - Array of objects
// - File (.csv/.tsv, .parquet/.pq, .arrow, .json)
// - URL string to a file
// - Apache Arrow Table (binary data Uint8Array/ArrayBuffer)
// - DuckDB table name if connection is passed into the Cosmograph constructor
const rawPoints = [{ id: 'a' }, { id: 'b' }, { id: 'c' }]
const rawLinks = [
{ source: 'a', target: 'b' },
{ source: 'b', target: 'c' },
{ source: 'c', target: 'a' },
]
export const component = (): JSX.Element => {
const [config, setConfig] = useState<CosmographConfig>({})
// Create a config to map your data into Cosmograph's internal format
const dataConfig = {
points: {
pointIdBy: 'id',
},
links: {
linkSourceBy: 'source',
linkTargetsBy: ['target'],
},
}
const loadData = async (): Promise<void> => {
// Prepare data and config for Cosmograph
const result = await prepareCosmographData(dataConfig, rawPoints, rawLinks)
if (result) {
// Update Cosmograph config with prepared output
const { points, links, cosmographConfig } = result
setConfig({ points, links, ...cosmographConfig })
}
}
// Load data when component is mounted
useEffect(() => { loadData() }, [])
return <Cosmograph {...config} />
}
CosmographProvider
CosmographProvider
connects Cosmograph
instance with companion components like CosmographTimeline
and CosmographHistogram
. Wrap your components with it to enable this connection:
import { CosmographProvider, CosmographHistogram, Cosmograph } from '@cosmograph/react'
function App() {
...
return (
<CosmographProvider>
<Cosmograph {...config} />
// Assuming you have a numeric column "value" in your points data
<CosmographHistogram accessor={"value"}>
</CosmographProvider>
)
}
⚠️ Note: Always place Cosmograph companion components inside
CosmographProvider
and make sure it hasCosmograph
instance inside.
useCosmograph
After surrounding your app with CosmographProvider
, the useCosmograph
hook can now be used to access the Cosmograph
instance.
import { useCosmograph, CosmographProvider } from '@cosmograph/react'
const FitButton = () => {
const { cosmograph } = useCosmograph()
return (
<button onClick={() => cosmograph.fitView()}>
Fit View
</button>
)
}
const App = () => {
...
return (
<CosmographProvider>
<Cosmograph {...config}/>
<FitButton />
</CosmographProvider>
)
}
❗ Note:
useCosmograph
must be called within a component that is a direct descendant of theCosmographProvider
. Attempting to use it outside of the provider will trigger an error.
Cosmograph
exposes a ref
property that can be used to access the underlying Cosmograph
instance, giving you direct access to its methods and properties.
import React, { useRef } from 'react'
import { CosmographRef, Cosmograph } from '@cosmograph/react'
function App () {
...
const cosmograph = useRef<CosmographRef>()
const onClick = (pointIndex: number | undefined) => {
cosmograph.current?.selectPoint(point)
}}
return (
<Cosmograph {...config} onClick={onClick} ref={cosmograph}/>
)
}
@cosmograph/react
provides few components ready for use:
Cosmograph
: The primary component.CosmographTimeline
: Offers a timeline feature for visualizing data over time.CosmographHistogram
: A fully customizable histogram component.CosmographSizeLegend
: A legend component for visualizing data sizes.CosmographTypeColorLegend
: A legend component for visualizing data types with colors.CosmographRangeColor
: A component for visualizing numerical data with colors.CosmographBars
: A component for visualizing data with categorical bars that allow to search, sort and filter these categories.✏️ Note: All the components included support refs and can be accessed via
useRef
oruseCallback
for developers to have better control and management. Read the docs for more details.
While currently closed source, we welcome your feedback! Help improve Cosmograph by submitting bug reports and feature ideas in our issues repository.
Cosmograph is licensed under the CC-BY-NC-4.0 license and free for any non-commercial usage. If you want to use it in a commercial project, please reach out to us.
FAQs
Cosmograph React Wrapper
The npm package @cosmograph/react receives a total of 665 weekly downloads. As such, @cosmograph/react popularity was classified as not popular.
We found that @cosmograph/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.