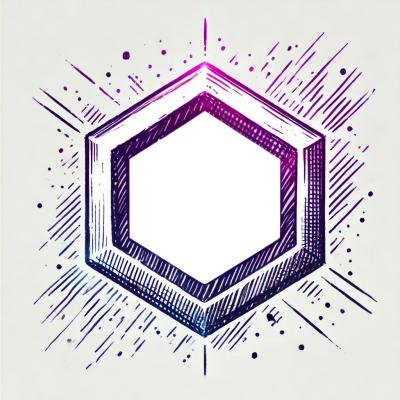
Security News
Maven Central Adds Sigstore Signature Validation
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
@costlydeveloper/ngx-awesome-popup
Advanced tools
Gives new functionality to Angular 9+, generates beautiful popups, dialogs, ConfirmBoxes, AlertBoxes, ToastNotifications. Also gives the ability of opening dynamic components directly from typescript!
Gives new functionality to Angular 9+, generates beautiful popups, dialogs, ConfirmBoxes, AlertBoxes, ToastNotifications. Also gives the ability of opening dynamic components directly from typescript!
Use this popup generator to easily generate beautiful, highly scalable popups. From regular Angular component it renders dynamic component view in popup directly from typescript, including toast notifications, alert box or confirm box.
Install the library with:
npm install @costlydeveloper/ngx-awesome-popup
Then import it in your AppModule
:
import {NgModule} from '@angular/core';
import {BrowserModule} from '@angular/platform-browser';
import {AppComponent} from './app.component';
// Import your library
import {ConfirmBoxConfigModule, DialogConfigModule, NgxAwesomePopupModule, ToastNotificationConfigModule} from '@costlydeveloper/ngx-awesome-popup';
@NgModule({
declarations: [
AppComponent
],
imports : [
BrowserModule,
// Importing @costlydeveloper/ngx-awesome-popup modules
NgxAwesomePopupModule.forRoot(),
DialogConfigModule.forRoot(),
ConfirmBoxConfigModule.forRoot(),
ToastNotificationConfigModule.forRoot()
],
providers : [],
bootstrap : [AppComponent]
})
export class AppModule {
}
API documentation:
Check The API Documentation for more advance setup.
Simply open toast notification from any component or any custom typescript class:
import {Component, OnInit} from '@angular/core';
// import library classes
import {DialogLayoutDisplay, ToastNotificationInitializer} from '@costlydeveloper/ngx-awesome-popup';
@Component({
selector : 'app-root',
templateUrl: './app.component.html',
styleUrls : ['./app.component.scss']
})
export class AppComponent implements OnInit {
ngOnInit() {
// Call the method
this.toastNotification();
}
// Create the method
toastNotification() {
const newToastNotification = new ToastNotificationInitializer();
newToastNotification.setTitle('Warning!');
newToastNotification.setMessage('Form is not valid!');
// Choose layout color type
newToastNotification.setConfig({
LayoutType: DialogLayoutDisplay.WARNING // SUCCESS | INFO | NONE | DANGER | WARNING
});
// Simply open the popup
newToastNotification.openToastNotification$();
}
}
API documentation:
It is very easy to open Confirm Box or Alert Box from any component or any custom typescript class:
import {Component, OnInit} from '@angular/core';
// import library classes
import {DialogLayoutDisplay, ConfirmBoxInitializer} from '@costlydeveloper/ngx-awesome-popup';
@Component({
selector : 'app-root',
templateUrl: './app.component.html',
styleUrls : ['./app.component.scss']
})
export class AppComponent implements OnInit {
ngOnInit() {
// Call the method
this.confirmBox();
}
// Create the method
confirmBox() {
const confirmBox = new ConfirmBoxInitializer();
confirmBox.setTitle('Are you sure?');
confirmBox.setMessage('Confirm to delete user: John Doe!');
confirmBox.setButtonLabels('YES', 'NO');
// Choose layout color type
confirmBox.setConfig({
LayoutType: DialogLayoutDisplay.DANGER // SUCCESS | INFO | NONE | DANGER | WARNING
});
// Simply open the popup and listen which button is clicked
const subscription = confirmBox.openConfirmBox$().subscribe(resp => {
// IConfirmBoxPublicResponse
console.log('Clicked button response: ', resp);
subscription.unsubscribe();
});
}
}
API documentation:
Simply open any Angular component from any typescript file without HTML selector.
import {Component, OnInit} from '@angular/core';
// import desired angular component for DialogInitializer
import {AnyAngularComponent} from './any-angular-component/any-angular.component';
// import library classes
import {DialogLayoutDisplay, DialogInitializer, ButtonLayoutDisplay, ButtonMaker} from '@costlydeveloper/ngx-awesome-popup';
@Component({
selector : 'app-root',
templateUrl: './app.component.html',
styleUrls : ['./app.component.scss']
})
export class AppComponent implements OnInit {
ngOnInit() {
// Call the method.
this.dialog();
}
// Create the method.
dialog() {
// Instance of DialogInitializer includes any valid angular component as argument.
const dialogPopup = new DialogInitializer(AnyAngularComponent);
// Any data can be sent to AnyAngularComponent.
dialogPopup.setCustomData({name: 'John', surname: 'Doe', id: 1});
// Set some configuration.
dialogPopup.setConfig({
Width : '500px',
LayoutType: DialogLayoutDisplay.NONE // SUCCESS | INFO | NONE | DANGER | WARNING
});
// Set some custom buttons as list.
// SUCCESS | INFO | NONE | DANGER | WARNING | PRIMARY | SECONDARY | LINK | DARK | LIGHT
dialogPopup.setButtons([
new ButtonMaker('Edit', 'edit', ButtonLayoutDisplay.WARNING),
new ButtonMaker('Submit', 'submit', ButtonLayoutDisplay.SUCCESS),
new ButtonMaker('Cancel', 'cancel', ButtonLayoutDisplay.SECONDARY)
]);
// Simply open the popup and listen which button is clicked and,
// receive optional payload from AnyAngularComponent.
const subscription = dialogPopup.openDialog$().subscribe(resp => {
// IDialogPublicResponse
console.log('dialog response: ', resp);
subscription.unsubscribe();
});
}
}
API documentation:
The child dynamic component represents AnyAngularComponent from example above.
import { Component, OnInit, OnDestroy } from '@angular/core';
import {Subscription} from 'rxjs';
import {DialogBelonging} from '@costlydeveloper/ngx-awesome-popup';
@Component({
selector: 'app-any-angular-component',
templateUrl: './any-angular.component.html',
styleUrls: ['./any-angular.component.scss']
})
export class AnyAngularComponent implements OnInit, OnDestroy{
subscriptions: Subscription[] = [];
// Dependency Injection of the dialogBelonging in constructor is crucial.
constructor(private dialogBelonging: DialogBelonging) {}
ngOnInit(): void {
// Check recived data and other available features.
console.log(this.dialogBelonging);
// Subscribe to button listeners.
this.subscriptions.push(
// IDialogEventsController
this.dialogBelonging.EventsController.onButtonClick$.subscribe((_Button) => {
if (_Button.ID === 'edit') {
// Do some logic for example edit user.
} else if (_Button.ID === 'submit') {
// Do some logic and close popup.
this.dialogBelonging.EventsController.close();
}
else if (_Button.ID === 'cancel') {
// Do some logic and close popup.
this.dialogBelonging.EventsController.close();
}
})
);
// Timeout emulates asyinc data.
setTimeout(() => {
// Close the loader after some data is ready.
// IDialogEventsController
this.dialogBelonging.EventsController.closeLoader();
}, 1000);
}
ngOnDestroy(): void {
// Care about memory and close all subscriptions.
this.subscriptions.forEach(sub => sub.unsubscribe());
}
}
API documentation:
Licensed under MIT.
FAQs
The world's easiest, most powerful Angular dialog modal framework. It generates beautiful popups, dialogs, Confirm Boxes, Alert Boxes, Toast Notifications. Also gives the ability of opening dynamic components directly from typescript!
The npm package @costlydeveloper/ngx-awesome-popup receives a total of 0 weekly downloads. As such, @costlydeveloper/ngx-awesome-popup popularity was classified as not popular.
We found that @costlydeveloper/ngx-awesome-popup demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Maven Central now validates Sigstore signatures, making it easier for developers to verify the provenance of Java packages.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.