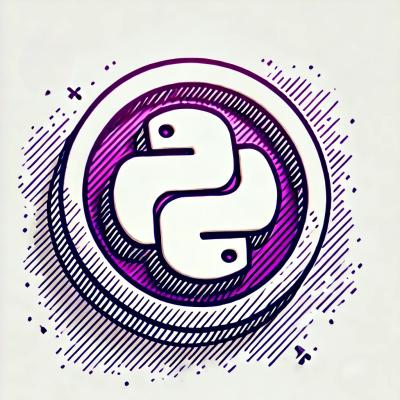
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@cubone/react-file-manager
Advanced tools
React File Manager is an open-source, user-friendly component designed to easily manage files and folders within applications. With smooth drag-and-drop functionality, responsive design, and efficient navigation, it simplifies file handling in any React p
An open-source React.js package for easy integration of a file manager into applications. It provides a user-friendly interface for managing files and folders, including viewing, uploading, and deleting, with full UI and backend integration.
To install React File Manager
, use the following command:
npm i @cubone/react-file-manager
Here’s a basic example of how to use the File Manager Component in your React application:
import { useState } from "react";
import { FileManager } from "@cubone/react-file-manager";
import "@cubone/react-file-manager/dist/style.css";
function App() {
const [files, setFiles] = useState([
{
name: "Documents",
isDirectory: true, // Folder
path: "/Documents", // Located in Root directory
updatedAt: "2024-09-09T10:30:00Z", // Last updated time
},
{
name: "Pictures",
isDirectory: true,
path: "/Pictures", // Located in Root directory as well
updatedAt: "2024-09-09T11:00:00Z",
},
{
name: "Pic.png",
isDirectory: false, // File
path: "/Pictures/Pic.png", // Located inside the "Pictures" folder
updatedAt: "2024-09-08T16:45:00Z",
size: 2048, // File size in bytes (example: 2 KB)
},
]);
return (
<>
<FileManager files={files} />
</>
);
}
export default App;
The files
prop accepts an array of objects, where each object represents a file or folder. You can
customize the structure to meet your application needs. Each file or folder object follows the
structure detailed below:
type File = {
name: string;
isDirectory: boolean;
path: string;
updatedAt?: string; // Optional: Last update timestamp in ISO 8601 format
size?: number; // Optional: File size in bytes (only applicable for files)
};
Name | Type | Description |
---|---|---|
acceptedFileTypes | string | (Optional) A comma-separated list of allowed file extensions for uploading specific file types (e.g., .txt, .png, .pdf ). If omitted, all file types are accepted. |
enableFilePreview | boolean | A boolean flag indicating whether to use the default file previewer in the file manager default: true . |
filePreviewPath | string | The base URL for file previews e.g.https://example.com , file path will be appended automatically to it i.e. https://example.com/yourFilePath . |
filePreviewComponent | (file: File) => React.ReactNode | (Optional) A callback function that provides a custom file preview. It receives the selected file as its argument and must return a valid React node, JSX element, or HTML. Use this prop to override the default file preview behavior. Example: Custom Preview Usage. |
fileUploadConfig | { url: string; method?: "POST" | "PUT"; headers?: { [key: string]: string } } | Configuration object for file uploads. It includes the upload URL (url ), an optional HTTP method (method , default is "POST" ), and an optional headers object for setting custom HTTP headers in the upload request. The method property allows only "POST" or "PUT" values. The headers object can accept any standard or custom headers required by the server. Example: { url: "https://example.com/fileupload", method: "PUT", headers: { Authorization: "Bearer " + TOKEN, "X-Custom-Header": "value" } } |
files | Array<File> | An array of file and folder objects representing the current directory structure. Each object includes name , isDirectory , and path properties. |
fontFamily | string | The font family to be used throughout the component. Accepts any valid CSS font family (e.g., 'Arial, sans-serif' , 'Roboto' ). You can customize the font styling to match your application's theme. default: 'Nunito Sans, sans-serif' . |
height | string | number | The height of the component default: 600px . Can be a string (e.g., '100%' , '10rem' ) or a number (in pixels). |
initialPath | string | The path of the directory to be loaded initially e.g. /Documents . This should be the path of a folder which is included in files array. Default value is "" |
isLoading | boolean | A boolean state indicating whether the application is currently performing an operation, such as creating, renaming, or deleting a file/folder. Displays a loading state if set true . |
layout | "list" | "grid" | Specifies the default layout style for the file manager. Can be either "list" or "grid". Default value is "grid". |
maxFileSize | number | For limiting the maximum upload file size in bytes. |
onCopy | (files: Array<File>) => void | (Optional) A callback function triggered when one or more files or folders are copied providing copied files as an argument. Use this function to perform custom actions on copy event. |
onCut | (files: Array<File>) => void | (Optional) A callback function triggered when one or more files or folders are cut, providing the cut files as an argument. Use this function to perform custom actions on the cut event. |
onCreateFolder | (name: string, parentFolder: File) => void | A callback function triggered when a new folder is created. Use this function to update the files state to include the new folder under the specified parent folder using create folder API call to your server. |
onDelete | (files: Array<File>) => void | A callback function is triggered when one or more files or folders are deleted. |
onDownload | (files: Array<File>) => void | A callback function triggered when one or more files or folders are downloaded. |
onError | (error: { type: string, message: string }, file: File) => void | A callback function triggered whenever there is an error in the file manager. Where error is an object containing type ("upload", etc.) and a descriptive error message . |
onFileOpen | (file: File) => void | A callback function triggered when a file or folder is opened. |
onFileUploaded | (response: { [key: string]: any }) => void | A callback function triggered after a file is successfully uploaded. Provides JSON response holding uploaded file details, use it to extract the uploaded file details and add it to the files state e.g. setFiles((prev) => [...prev, JSON.parse(response)]); |
onFileUploading | (file: File, parentFolder: File) => { [key: string]: any } | A callback function triggered during the file upload process. You can also return an object with key-value pairs that will be appended to the FormData along with the file being uploaded. The object can contain any number of valid properties. |
onLayoutChange | (layout: "list" | "grid") => void | A callback function triggered when the layout of the file manager is changed. |
onPaste | (files: Array<File>, destinationFolder: File, operationType: "copy" | "move") => void | A callback function triggered when when one or more files or folders are pasted into a new location. Depending on operationType , use this to either copy or move the sourceItem to the destinationFolder , updating the files state accordingly. |
onRefresh | () => void | A callback function triggered when the file manager is refreshed. Use this to refresh the files state to reflect any changes or updates. |
onRename | (file: File, newName: string) => void | A callback function triggered when a file or folder is renamed. |
onSelect | (files: Array<File>) => void | (Optional) A callback function triggered whenever a file or folder is selected. The function receives an array of selected files or folders, allowing you to handle selection-related actions, such as displaying file details, enabling toolbar actions, or updating the UI accordingly. |
primaryColor | string | The primary color for the component's theme. Accepts any valid CSS color format (e.g., 'blue' , '#E97451' , 'rgb(52, 152, 219)' ). This color will be applied to buttons, highlights, and other key elements. default: #6155b4 . |
width | string | number | The width of the component default: 100% . Can be a string (e.g., '100%' , '10rem' ) or a number (in pixels). |
Action | Shortcut |
---|---|
New Folder | Alt + Shift + N |
Upload Files | CTRL + U |
Cut | CTRL + X |
Copy | CTRL + C |
Paste | CTRL + V |
Rename | F2 |
Download | CTRL + D |
Delete | DEL |
Select All Files | CTRL + A |
Select Multiple Files | CTRL + Click |
Select Range of Files | Shift + Click |
Switch to List Layout | CTRL + Shift + 1 |
Switch to Grid Layout | CTRL + Shift + 2 |
Jump to First File in the List | Home |
Jump to Last File in the List | End |
Refresh File List | F5 |
Clear Selection | Esc |
The FileManager
component allows you to provide a custom file preview by passing the
filePreviewComponent
prop. This is an optional callback function that receives the selected file
as an argument and must return a valid React node, JSX element, or HTML.
const CustomImagePreviewer = ({ file }) => {
return <img src={`${file.path}`} alt={file.name} />;
};
<FileManager
// Other props...
filePreviewComponent={(file) => <CustomImagePreviewer file={file} />}
/>;
React File Manager is MIT Licensed.
FAQs
React File Manager is an open-source, user-friendly component designed to easily manage files and folders within applications. With smooth drag-and-drop functionality, responsive design, and efficient navigation, it simplifies file handling in any React p
The npm package @cubone/react-file-manager receives a total of 299 weekly downloads. As such, @cubone/react-file-manager popularity was classified as not popular.
We found that @cubone/react-file-manager demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.