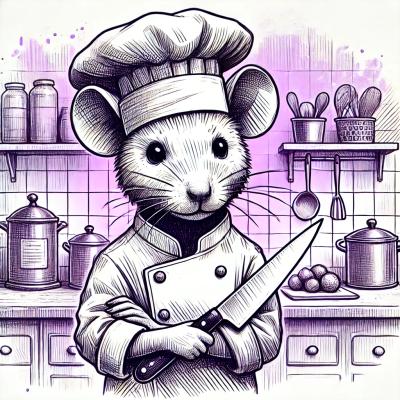
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@datadog/native-metrics
Advanced tools
The @datadog/native-metrics package is designed to provide native system metrics for Node.js applications. It allows Datadog users to collect and report metrics such as garbage collection, heap statistics, and other Node.js process-level metrics to the Datadog platform, where they can be visualized and monitored.
Garbage Collection Metrics
This feature allows you to monitor garbage collection events in your Node.js application. The event listener logs information about each garbage collection event.
const NativeMetrics = require('@datadog/native-metrics');
const metrics = new NativeMetrics();
metrics.on('gc', (info) => {
console.log('GC event info:', info);
});
Heap Statistics
This feature provides heap statistics from the V8 engine at regular intervals. It can be used to monitor the memory usage of your Node.js application.
const NativeMetrics = require('@datadog/native-metrics');
const metrics = new NativeMetrics();
setInterval(() => {
const stats = metrics.getHeapStatistics();
console.log('Heap stats:', stats);
}, 10000);
Event Loop Lag
This feature measures the lag in the Node.js event loop, which can be an indicator of the application's responsiveness.
const NativeMetrics = require('@datadog/native-metrics');
const metrics = new NativeMetrics();
setInterval(() => {
const lag = metrics.getLoopDelay();
console.log('Event loop lag:', lag);
}, 10000);
Appmetrics is a package that provides performance monitoring for Node.js applications. It includes features like CPU profiling, HTTP monitoring, and memory tracking. While it offers a broader range of metrics, it is not tailored specifically for integration with Datadog.
Node-gyp is a cross-platform command-line tool written in Node.js for compiling native addon modules for Node.js. It is not a direct alternative to @datadog/native-metrics but is often used to build the native components that packages like @datadog/native-metrics rely on.
Clinic is an open-source Node.js performance profiling tool. It provides a suite of tools to help diagnose and pinpoint performance bottlenecks. Unlike @datadog/native-metrics, Clinic is more focused on profiling and analysis rather than continuous monitoring and integration with a platform like Datadog.
Native metrics collector for libuv and v8.
FAQs
Native metrics collector for libuv and v8
The npm package @datadog/native-metrics receives a total of 745,934 weekly downloads. As such, @datadog/native-metrics popularity was classified as popular.
We found that @datadog/native-metrics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.