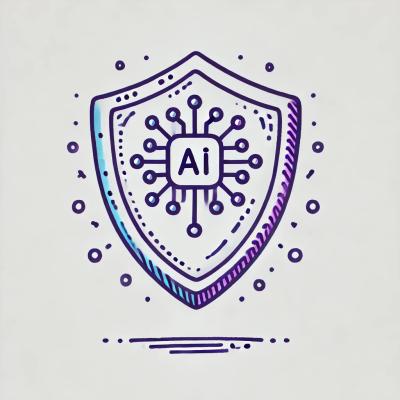
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
@datafire/ebay_commerce_charity
Advanced tools
Client library for Charity API
npm install --save @datafire/ebay_commerce_charity
let ebay_commerce_charity = require('@datafire/ebay_commerce_charity').create({
access_token: "",
refresh_token: "",
client_id: "",
client_secret: "",
redirect_uri: ""
});
.then(data => {
console.log(data);
});
The Charity API allows third-party developers to search for and access details on supported charitable organizations.
Exchange the code passed to your redirect URI for an access_token
ebay_commerce_charity.oauthCallback({
"code": ""
}, context)
object
string
object
string
string
string
string
string
Exchange a refresh_token for an access_token
ebay_commerce_charity.oauthRefresh(null, context)
This action has no parameters
object
string
string
string
string
string
This call is used to search for supported charitable organizations. It allows users to search for a specific charitable organization, or for multiple charitable organizations, from a particular charitable domain and/or geographical region, or by using search criteria. The call returns paginated search results containing the charitable organizations that match the specified criteria.
ebay_commerce_charity.getCharityOrgs({
"X-EBAY-C-MARKETPLACE-ID": ""
}, context)
object
string
: The number of items, from the result set, returned in a single page. Valid Values: 1-100 Default: 20string
: The number of items that will be skipped in the result set. This is used with the limit field to control the pagination of the output. For example, if the offset is set to 0 and the limit is set to 10, the method will retrieve items 1 through 10 from the list of items returned. If the offset is set to 10 and the limit is set to 10, the method will retrieve items 11 through 20 from the list of items returned. Valid Values: 0-10,000 Default: 0string
: A query string that matches the keywords in name, mission statement, or description.string
: A comma-separated list of charitable organization registration IDs. Note: Do not specify this parameter for query-based searches. Specify either the q or registration_ids parameter, but not both. Maximum Limit: 20string
: A header used to specify the eBay marketplace ID. Valid Values: EBAY_GB and EBAY_USThis call allows users to retrieve the details for a specific charitable organization using its legacy charity ID, which has also been referred to as the charity number, external ID, and PayPal Giving Fund ID. The legacy charity ID is separate from eBay’s generic charity ID.
ebay_commerce_charity.getCharityOrgByLegacyId({
"X-EBAY-C-MARKETPLACE-ID": "",
"legacy_charity_org_id": ""
}, context)
object
string
: A header used to specify the eBay marketplace ID. Valid Values: EBAY_GB and EBAY_USstring
: The legacy ID of the charitable organization. Note: The legacy charity ID is the identifier assigned to an organization upon registration with the PayPal Giving Fund (PPGF). It has also been referred to as the external ID/charity number.This call is used to retrieve detailed information about supported charitable organizations. It allows users to retrieve the details for a specific charitable organization using its charity organization ID. The call returns the full details for the charitable organization that matches the specified ID.
ebay_commerce_charity.getCharityOrg({
"charity_org_id": "",
"X-EBAY-C-MARKETPLACE-ID": ""
}, context)
object
string
: The unique ID of the charitable organization.string
: A header used to specify the eBay marketplace ID. Valid Values: EBAY_GB and EBAY_USobject
: The physical location of the item.
string
: The city of the charitable organization.string
: The two-letter ISO 3166 standard of the country of the address. For implementation help, refer to eBay API documentationstring
: The postal code of the charitable organization.string
: The state or province of the charitable organization.object
: The full location, ID, logo and other details of the charity organization.
string
: The ID of the charitable organization.string
: The description of the charitable organization.string
: The mission statement of the charitable organization.string
: The name of the charitable organization.string
: The registration ID for the charitable organization. For the US marketplace, this is the EIN.string
: The link to the website for the charitable organization.object
: A single set of search results, with information for accessing other sets.
array
: The list of charitable organizations that match the search criteria.
string
: The relative path to the current set of results.integer
: The number of items, from the result set, returned in a single page. Valid Values: 1-100 Default: 20string
: The relative path to the next set of results.integer
: The number of items that will be skipped in the result set. This is used with the limit field to control the pagination of the output. For example, if the offset is set to 0 and the limit is set to 10, the method will retrieve items 1 through 10 from the list of items returned. If the offset is set to 10 and the limit is set to 10, the method will retrieve items 11 through 20 from the list of items returned. Valid Values: 0-10,000 Default: 0string
: The relative path to the previous set of results.integer
: The total number of matches for the search criteria.object
: This type defines the fields that can be returned in an error.
array
: An array of name/value pairs that describe details the error condition. These are useful when multiple errors are returned.
string
: Identifies the type of erro.string
: Name for the primary system where the error occurred. This is relevant for application errors.integer
: A unique number to identify the error.array
: An array of request elements most closely associated to the error.
string
string
: A more detailed explanation of the error.string
: Information on how to correct the problem, in the end user's terms and language where applicable.array
: An array of request elements most closely associated to the error.
string
string
: Further helps indicate which subsystem the error is coming from. System subcategories include: Initialization, Serialization, Security, Monitoring, Rate Limiting, etc.object
string
: The object of the error.string
: The value of the object.object
: Defines the format of a geographic coordinate.
number
: The latitude component of the geographic coordinate.number
: The longitude component of the geographic coordinate.object
: The logo of the charitable organization.
string
: The height of the logo image.string
: The URL to the logo image location.string
: The width of the logo image.object
FAQs
DataFire integration for Charity API
The npm package @datafire/ebay_commerce_charity receives a total of 1 weekly downloads. As such, @datafire/ebay_commerce_charity popularity was classified as not popular.
We found that @datafire/ebay_commerce_charity demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.