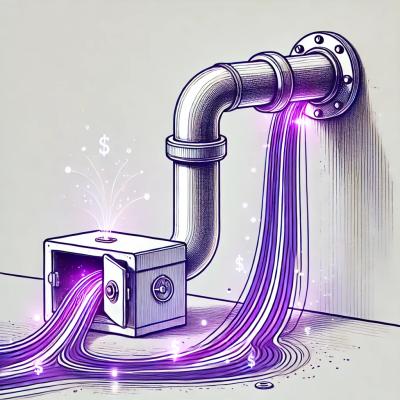
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@datafire/ebay_developer_analytics
Advanced tools
DataFire integration for Progress to Rate Limit API
Client library for Progress to Rate Limit API
npm install --save @datafire/ebay_developer_analytics
let ebay_developer_analytics = require('@datafire/ebay_developer_analytics').create({
access_token: "",
refresh_token: "",
client_id: "",
client_secret: "",
redirect_uri: ""
});
.then(data => {
console.log(data);
});
The Analytics API retrieves call-limit data and the quotas that are set for the RESTful APIs and their associated resources.
Responses from calls made to getRateLimits and getUerRateLimits include a list of the applicable resources and the "call limit", or quota, that is set for each resource. In addition to quota information, the response also includes the number of remaining calls available before the limit is reached, the time remaining before the quota resets, and the length of the "time window" to which the quota applies.
The getRateLimits and getUserRateLimits methods retrieve call-limit information for either an application or user, respectively, and each method must be called with an appropriate OAuth token. That is, getRateLimites requires an access token generated with a client credentials grant and getUserRateLimites requires requires an access token generated with an authorization code grant. For more information, see OAuth tokens.
Users can analyze the response data to see whether or not a limit might be reached, and from that determine if any action needs to be taken (such as programmatically throttling their request rate). For more on call limits, see Compatible Application Check.
Exchange the code passed to your redirect URI for an access_token
ebay_developer_analytics.oauthCallback({
"code": ""
}, context)
object
string
object
string
string
string
string
string
Exchange a refresh_token for an access_token
ebay_developer_analytics.oauthRefresh(null, context)
This action has no parameters
object
string
string
string
string
string
This method retrieves the call limit and utilization data for an application. The data is retrieved for all RESTful APIs and resources. The response from getRateLimits includes a list of the applicable resources and the "call limit", or quota, that is set for each resource. In addition to quota information, the response also includes the number of remaining calls available before the limit is reached, the time remaining before the quota resets, and the length of the "time window" to which the quota applies. By default, this method returns utilization data for all RESTful API resources. Use the api_name and api_context query parameters to filter the response to only the desired APIs. For more on call limits, see Compatible Application Check.
ebay_developer_analytics.getRateLimits({}, context)
object
string
: This optional query parameter filters the result to include only the specified API context. Acceptable values for the parameter are buy, sell, commerce, and developer.string
: This optional query parameter filters the result to include only the APIs specified. Example values are browse for the Buy APIs context, inventory for the Sell APIs context, and taxonomy for the Commerce APIs context.This method retrieves the call limit and utilization data for an application user. The call-limit data is returned for all RESTful APIs and resources that limit calls on a per-user basis. The response from getUserRateLimits includes a list of the applicable resources and the "call limit", or quota, that is set for each resource. In addition to quota information, the response also includes the number of remaining calls available before the limit is reached, the time remaining before the quota resets, and the length of the "time window" to which the quota applies. By default, this method returns utilization data for all RESTful API resources that limit request access by user. Use the api_name and api_context query parameters to filter the response to only the desired APIs. For more on call limits, see Compatible Application Check.
ebay_developer_analytics.getUserRateLimits({}, context)
object
string
: This optional query parameter filters the result to include only the specified API context. Acceptable values for the parameter are buy, sell, commerce, and developer.string
: This optional query parameter filters the result to include only the APIs specified. Example values are browse for the Buy APIs context, inventory for the Sell APIs context, and taxonomy for the Commerce APIs context.object
: This type defines the fields that can be returned in an error.
array
: An array of name/value pairs that describe details the error condition. These are useful when multiple errors are returned.
string
: Identifies the type of erro.string
: Name for the primary system where the error occurred. This is relevant for application errors.integer
: A unique number to identify the error.array
: An array of request elements most closely associated to the error.
string
string
: A more detailed explanation of the error.string
: Information on how to correct the problem, in the end user's terms and language where applicable.array
: An array of request elements most closely associated to the error.
string
string
: Further helps indicate which subsystem the error is coming from. System subcategories include: Initialization, Serialization, Security, Monitoring, Rate Limiting, etc.object
string
: The object of the error.string
: The value of the object.object
: This complex type defines a "rate" as the quota of calls that can be made to a resource per time window, the remaining number of calls before the threshold is met, the amount of time until the time window resets, and the length of the time window (in seconds).
integer
: The maximum number of requests that can be made to this resource during a set time period. The length of time to which the limit is applied is defined by the associated timeWindow value. This value is often referred to as the "call quota" for the resource.integer
: The remaining number of requests that can be made to this resource before the associated time window resets.string
: The data and time the time window and accumulated calls for this resource reset. When the reset time is reached, the remaining value is reset to the value of limit, and this reset value is reset to the current time plus the number of seconds defined by the timeWindow value. The time stamp is formatted as an ISO 8601 string, which is based on the 24-hour Universal Coordinated Time (UTC) clock. Format: YYYY-MM-DDTHH:MM:SS.SSSZ Example: 2018-08-04T07:09:00.000Zinteger
: A period of time, expressed in seconds. The call quota for a resource is applied to the period of time defined by the value of this field.object
: This complex types defines the resource (such as an API method) for which the rate-limit data is returned. A method is included in an API, and an API is part of an API context for the API version specified.
string
: The context of the API for which rate-limit data is returned. For example buy, sell, commerce, or developer.string
: The name of the API for which rate-limit data is returned. For example browse for the Buy API, inventory for the Sell API, or taxonomy for the Commerce API.string
: The version of the API for which rate-limit data is returned. For example v1 or v2.array
: A list of the methods for which rate-limit data is returned. For example item for the Feed API, getOrder for the Fulfillment API, and getProduct for the Catalog API.
object
: This complex type defines a list of rate-limit data as it pertains to a method within the specified version of an API.
array
: The rate-limit data for the specified APIs. The rate-limit data is returned for all the methods in the specified APIs and data pertains to the current time window.
object
: This complex type defines the resource (API method) and the current rate-limit data for that resource.
string
: The name of the resource (an API or an API method) to which the rate-limit data applies.array
: A list of rate-limit data, where each list element represents the rate-limit data for a specific resource.
FAQs
DataFire integration for Progress to Rate Limit API
The npm package @datafire/ebay_developer_analytics receives a total of 0 weekly downloads. As such, @datafire/ebay_developer_analytics popularity was classified as not popular.
We found that @datafire/ebay_developer_analytics demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.