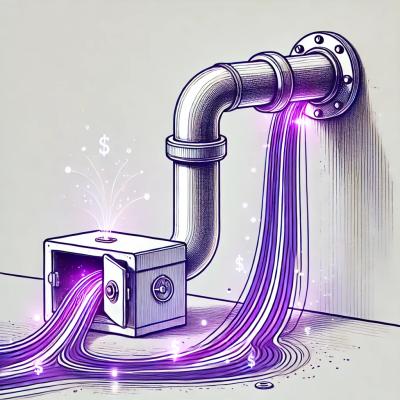
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@datafire/ebay_sell_listing
Advanced tools
Client library for Listing API
npm install --save @datafire/ebay_sell_listing
let ebay_sell_listing = require('@datafire/ebay_sell_listing').create({
access_token: "",
refresh_token: "",
client_id: "",
client_secret: "",
redirect_uri: ""
});
.then(data => {
console.log(data);
});
Enables a seller adding an ad or item on a Partner's site to automatically create an eBay listing draft using the item details from the Partner's site.
Exchange the code passed to your redirect URI for an access_token
ebay_sell_listing.oauthCallback({
"code": ""
}, context)
object
string
object
string
string
string
string
string
Exchange a refresh_token for an access_token
ebay_sell_listing.oauthRefresh(null, context)
This action has no parameters
object
string
string
string
string
string
This call gives Partners the ability to create an eBay draft of a item for their seller using information from their site. This lets the Partner increase the exposure of items on their site and leverage the eBay user listing experience seamlessly. This experience provides guidance on pricing, aspects, etc. and recommendations that help create a listing that is complete and improves the exposure of the listing in search results. After the listing draft is created, the seller logs into their eBay account and uses the listing experience to finish the listing and publish the item on eBay.
ebay_sell_listing.createItemDraft({
"X-EBAY-C-MARKETPLACE-ID": ""
}, context)
object
string
: Use this header to specify the natural language of the seller. For details, see Content-Language in HTTP request headers. Required: For EBAY_CA in French. (Content-Language = fr-CA)string
: Use this header to specify an eBay marketplace ID. For a list of supported sites, see API Restrictions in the Listing API overview.object
: The type that defines the fields for the currency and a monetary amount.
string
: The three-letter ISO 4217 code representing the currency of the amount in the value field. Restriction: Only the currency of the marketplace is supported. For example, on the US marketplace the only currency supported is USD. For implementation help, refer to eBay API documentationstring
: The monetary amount, in the currency specified by the currency field.object
: The type that defines the fields for the item aspects.
string
: The name of an aspect, such and Brand.array
: A list of potential values for this aspect.
string
object
: This type is used to identify the charitable organization that will receive a percentage of sale proceeds for each sale generated by the listing. This container also includes the donation percentage, which is the percentage of the sale proceeds that the charitable organization will get. In order to receive a percentage of the sales proceeds, the non-profit organization must be registered with the PayPal Giving Fund, which is a partner of eBay for Charity.
string
: The eBay-assigned unique identifier of the charitable organization that will receive a percentage of the sales proceeds. The charitable organization must be reqistered with the PayPal Giving Fund in order to receive sales proceeds through eBay listings. This field is conditionally required if a seller is planning on donating a percentage of the sale proceeds to a charitable organization. The eBay-assigned unique identifier of a charitable organization can be found using the GetCharities call of the Trading API. In the GetCharities call response, this unique identifier is shown in the id attribute of the Charity container.string
: This field sets the percentage of the purchase price that the charitable organization (identified in the charityId field) will receive for each sale that the listing generates. This field is conditionally required if a seller is planning on donating a percentage of the sale proceeds to a charitable organization. This numeric value can range from 10 to 100, and in any 5 (percent) increments in between this range (e.g. 10, 15, 20...95,... 100). The seller would pass in 10 for 10 percent, 15 for 15 percent, 20 for 20 percent, and so on, all the way to 100 for 100 percent. Note: For this field, createItemDraft will only validate that a positive integer value is supplied, so the listing draft will still be successfully created (with no error or warning message) if a non-supported value is specified. However, if the seller attempted to publish this listing draft with an unsupported value, the charity information would just be dropped from the listing.object
: This type defines the fields that can be returned in an error.
array
: An array of name/value pairs that describe details the error condition. These are useful when multiple errors are returned.
string
: Identifies the type of erro.string
: Name for the primary system where the error occurred. This is relevant for application errors.integer
: A unique number to identify the error.array
: An array of request elements most closely associated to the error.
string
string
: A more detailed explanation of the error.string
: Information on how to correct the problem, in the end user's terms and language where applicable.array
: An array of request elements most closely associated to the error.
string
string
: Further helps indicate which subsystem the error is coming from. System subcategories include: Initialization, Serialization, Security, Monitoring, Rate Limiting, etc.object
string
: The object of the error.string
: The value of the object.object
: The type that defines the fields for the listing details.
string
: The ID of the leaf category associated with this item. A leaf category is the lowest level in that category and has no children. Note: If you submit both a category ID and an EPID, eBay determines the best category based on the EPID and uses that. The category ID will be ignored.string
: An enumeration value representing the condition of the item, such as NEW. Note: In the US and Australian marketplaces, Condition ID 2000 now maps to an item condition of 'Certified Refurbished', but this item condition is only available for use for a select number of US and Australian sellers. Other sellers on these two marketplaces will get an error when attempting to create an item draft with the Listing API using the 'Manufacturer Refurbished' item condition. For all other marketplaces besides the US and Australia, Condition ID 2000 still maps to 'Manufacturer Refurbished'. Any US or Australian seller who is interested in eligibility requirements to list with 'Certified Refurbished' should see the Certified refurbished program page in Seller Center. For implementation help, refer to eBay API documentationstring
: The format of the listing. Valid Values: FIXED_PRICE and AUCTION For implementation help, refer to eBay API documentationobject
: The type that defines the field for the createItemDraft response.
string
: The eBay generated ID of the listing draft.string
: The URI the Partner uses to send the seller to their listing draft that was created on the eBay site. From there the seller can change, update, and publish the item on eBay. This is returned when the seller is using a mobile app.string
: The web URL the Partner uses to send the seller to the listing draft that was created on the eBay site. From there the seller can change, update, and publish the item on eBay. This is returned when the seller is using mobile web (mweb) or the desktop web. Note: You must construct the URL using the URL returned in this field and a session token. For example: sellFlowUrl?id_token=session_tokenobject
: The type that defines the fields for the price details for an item.
object
: The type that defines the fields for the aspects of a product.
array
: The list of item aspects that describe the item (such as size, color, capacity, model, brand, etc.)
string
: The name brand of the item, such as Nike, Apple, etc.string
: The description of the item that was created by the seller. This field supports plain text or rich content within HTML tags. Note: Active content is not supported. Active content includes animation or video via JavaScript, Flash, plug-ins, or form actions. Max Length: 500,000string
: An EPID is the eBay product identifier of a product from the eBay product catalog. Note: If you submit both a category ID and an EPID, eBay determines the best category based on the EPID and uses that. The category ID will be ignored.array
: The image URLs of the item. The first URL will be the primary image, which appears on the View Item page in the eBay listing. The URL can be from the following: The eBay Picture Services (images previously uploaded). A server outside of eBay (self-hosted). For more details, see PictureURL and Introduction to Pictures in Listings. Maximum: 12 URLs (for most categories and marketplaces) Restrictions: You cannot mix self-hosted and EPS-hosted URLs in the same listing. All image URLs must be 'https'.
string
string
: The seller-created title of the item. This should include unique characteristics of the item, such as brand, model, color, size, capacity, etc. For example: Levi's 501 size 10 black jeansFAQs
DataFire integration for Listing API
We found that @datafire/ebay_sell_listing demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.