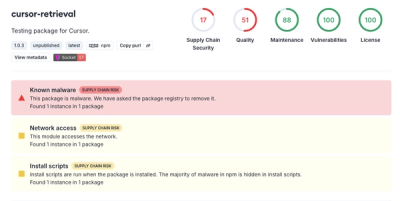
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@datastructures-js/linked-list
Advanced tools
a javascript implementation of LinkedList & DoublyLinkedList
a javascript implementation of LinkedList & DoublyLinkedList.
Linked List |
![]() |
Doubly Linked List |
![]() |
npm install --save @datastructures-js/linked-list
const {
LinkedListNode,
LinkedList,
DoublyLinkedListNode,
DoublyLinkedList,
} = require('@datastructures-js/linked-list');
import {
LinkedListNode,
LinkedList,
DoublyLinkedListNode,
DoublyLinkedList
} from '@datastructures-js/linked-list';
const linkedList = new LinkedList();
const doublyLinkedList = new DoublyLinkedList();
inserts a node at the beginning of the list.
params | return | runtime |
---|---|---|
value: any | LinkedList | DoublyLinkedList | O(1) |
console.log(linkedList.insertFirst(2).head().getValue()); // 2
console.log(linkedList.insertFirst(1).head().getValue()); // 1
inserts a node at the end of the list.
params | return | runtime |
---|---|---|
value: any | LinkedList | DoublyLinkedList |
LinkedList: O(n)
DoublyLinkedList: O(1) |
linkedList.insertLast(3);
const last1 = linkedList.insertLast(4).find(4);
console.log(last1.getValue()); // 4
console.log(last1.getNext()); // null
doublyLinkedList.insertLast(3);
const last2 = doublyLinkedList.insertLast(4).find(4);
console.log(last2.getValue()); // 4
console.log(last2.getPrev().getValue()); // 3
inserts a node at specific position of the list. First (head) node is at position 0.
params | return | runtime |
---|---|---|
position: number
value: any | LinkedList | DoublyLinkedList | O(n) |
const node1 = linkedList.insertAt(2, 5).find(5); // node1.getValue() = 5
const node2 = doublyLinkedList.insertAt(2, 5).find(5); // node2.getValue() = 5
Loop on the linked list from beginning to end, and pass each node to the callback.
params | runtime |
---|---|
cb: function | O(n) |
linkedList.forEach((node, position) => console.log(node.getValue(), position));
/*
2 0
1 1
5 2
3 3
4 4
*/
doublyLinkedList.forEach((node, position) => console.log(node.getValue(), position));
/*
2 0
1 1
5 2
3 3
4 4
*/
Only in DoublyLinkedList. Loop on the doubly linked list from end to beginning, and pass each node to the callback.
params | runtime |
---|---|
cb: function | O(n) |
doublyLinkedList.forEachReverse((node, position) => console.log(node.getValue(), position));
/*
4 4
3 3
5 2
1 1
2 0
*/
returns the first node that return true from the callback or null if nothing found.
params | return | runtime |
---|---|---|
cb: function | LinkedListNode | DoublyLinkedListNode | O(n) |
const node1 = linkedList.find((node) => node.getValue() === 5);
console.log(node1.getValue()); // 5
console.log(node1.getNext().getValue()); // 3
const node2 = doublyLinkedList.find((node) => node.getValue() === 5);
console.log(node2.getValue()); // 5
console.log(node2.getNext().getValue()); // 3
console.log(node2.getPrev().getValue()); // 1
returns a filtered linked list of all the nodes that returns true from the callback.
params | return | runtime |
---|---|---|
cb: function | LinkedList | DoublyLinkedList | O(n) |
const filterLinkedList = linkedList.filter((node, position) => node.getValue() > 2);
filterLinkedList.forEach((node) => console.log(node.getValue()));
/*
5
3
4
*/
const filteredDoublyLinkedList = doublyLinkedList.filter((node, position) => node.getValue() > 2);
filteredDoublyLinkedList.forEach((node) => console.log(node.getValue()));
/*
5
3
4
*/
converts the linked list into an array.
return | runtime |
---|---|
array | O(n) |
console.log(linkedList.toArray()); // [2, 1, 5, 3, 4]
console.log(doublyLinkedList.toArray()); // [2, 1, 5, 3, 4]
checks if the linked list is empty.
return | runtime |
---|---|
boolean | O(1) |
console.log(linkedList.isEmpty()); // false
console.log(doublyLinkedList.isEmpty()); // false
returns the head node in the linked list.
return | runtime |
---|---|
LinkedListNode | DoublyLinkedListNode | O(1) |
console.log(linkedList.head().getValue()); // 2
console.log(doublyLinkedList.head().getValue()); // 2
returns the tail node of the doubly linked list.
return | runtime |
---|---|
DoublyLinkedListNode | O(1) |
console.log(doublyLinkedList.tail().getValue()); // 4
returns the number of nodes in the linked list.
return | runtime |
---|---|
number | O(1) |
console.log(linkedList.count()); // 5
console.log(doublyLinkedList.count()); // 5
removes the first node in the list.
return | runtime |
---|---|
boolean | O(1) |
linkedList.removeFirst(); // true
doublyLinkedList.removeFirst(); // true
removes the last node in the list.
return | runtime |
---|---|
boolean |
LinkedList: O(n)
DoublyLinkedList: O(1) |
linkedList.removeLast(); // true
doublyLinkedList.removeLast(); // true
removes a node at a specific position. First (head) node is at position 0.
params | return | runtime |
---|---|---|
position: number | boolean | O(1) |
linkedList.removeAt(1); // true
doublyLinkedList.removeAt(1); // true
Loop on the linked list from beginning to end, removes the nodes that returns true from the callback.
params | return | runtime |
---|---|---|
cb: function | number (number of removed nodes) | O(n) |
linkedList.removeEach((node, position) => node.getValue() > 1); // 1
console.log(linkedList.toArray()); // [1]
doublyLinkedList.removeEach((node, position) => node.getValue() > 1); // 1
console.log(doublyLinkedList.toArray()); // [1]
clears the linked list.
runtime |
---|
O(1) |
linkedList.clear();
console.log(linkedList.count()); // 0
console.log(linkedList.head()); // null
doublyLinkedList.clear();
console.log(linkedList.count()); // 0
console.log(doublyLinkedList.head()); // null
console.log(doublyLinkedList.tail()); // null
sets the node's value.
params |
---|
value: any |
returns the node's value.
return |
---|
any |
sets the node's next connected node.
params |
---|
next: LinkedListNode |
returns the next connected node or null if it's the last node.
return |
---|
LinkedListNode |
sets the node's value.
params |
---|
value: any |
returns the node's value.
return |
---|
any |
sets the node's previous connected node.
params |
---|
prev: DoublyLinkedListNode |
returns the previous connected node or null if it's the first node.
return |
---|
DoublyLinkedListNode |
sets the node's next connected node.
params |
---|
next: DoublyLinkedListNode |
returns the next connected node or null if it's the last node.
return |
---|
DoublyLinkedListNode |
grunt build
The MIT License. Full License is here
[3.0.0] - 2020-12-30
.insertAt(position, value)
position now comes first then value..insert*
methods now returns a this
reference so it can be chained..forEach(cb)
callback now gets called with node and position (starting from 0);.forEachReverse(cb)
callback now gets called with node and position (starting from size -1);LinkedListNode
& DoublyLinkedListNode
classes are now exported in index.FAQs
a javascript implementation of LinkedList & DoublyLinkedList
The npm package @datastructures-js/linked-list receives a total of 3,007 weekly downloads. As such, @datastructures-js/linked-list popularity was classified as popular.
We found that @datastructures-js/linked-list demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.