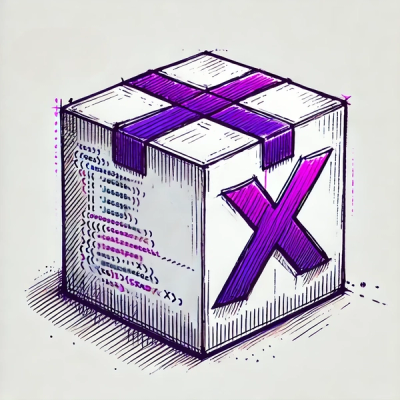
Security News
pnpm 10.0.0 Blocks Lifecycle Scripts by Default
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
@descope/node-sdk
Advanced tools
Use the Descope NodeJS SDK for NodeJS/Express to quickly and easily add user authentication to your application or website. If you need more background on how the ExpresSDKs work, click here.
This section will show you how to implement user authentication using a one-time password (OTP). A typical four step flow for OTP authentictaion is shown below.
flowchart LR
signup[1. customer sign-up]-- customer gets OTP -->verify[3. customer verification]
signin[2. customer sign-in]-- customer gets OTP -->verify
verify-- access private API -->validate[4. session validation]
Replace any instance of <ProjectID>
in the code below with your company's Project ID, which can be found in the Descope console.
Run the following commands in your project
These commands will add the Descope NodeJS SDK as a project dependency.
npm i --save @descope/node-sdk
Import and initialize the ExpresSDK for NodeJS client in your source code
import DescopeClient from '@descope/node-sdk';
const descopeClient = DescopeClient({ projectId: <ProjectID> });
or
```javascript
const sdk = require('@descope/node-sdk');
const descopeClient = sdk({ projectId: <ProjectID> });
```
In your sign-up route for OTP (for example, myapp.com/signup
) generate a sign-up request and send the OTP verification code via the selected delivery method. In the example below an email is sent to "mytestmail@test.com". In additon, optional user data (for exmaple, a custom username in the code sample below) can be gathered during the sign-up process.
await descopeClient.otp.signUp.email("mytestmail@test.com");
In your sign-in route for OTP (for exmaple, myapp.com/login
) generate a sign-in request send the OTP verification code via the selected delivery method. In the example below an email is sent to "mytestmail@test.com".
await descopeClient.otp.signIn.email("mytestmail@test.com");
In your verify customer route for OTP (for example, myapp.com/verify
) verify the OTP from either a customer sign-up or sign-in. The VerifyCode function call will write the necessary tokens and cookies to the response writer (w
), which will be used by the NodeJS client to validate each session interaction.
const out = await descopeClient.otp.verify.email(identifier, code);
if (out.data.cookies) {
res.set('Set-Cookie', out.data.cookies);
}
Session validation checks to see that the visitor to your website or application is who they say they are, by comparing the value in the validation variables against the session data that is already stored.
const out = await descopeClient.validateSession(session_jwt, refresh_jwt);
if (out?.cookies) {
res.set('Set-Cookie', out.cookies);
}
This section will help you implement user authentication using Magiclinks. A typical four step flow for OTP authentictaion is shown below.
flowchart LR
signup[1. customer sign-up]-- customer gets MagicLink -->verify[3. MagicLink verification]
signin[2. customer sign-in]-- customer gets MagicLink -->verify
verify-- access private API -->validate[4. session validation]
Replace any instance of <ProjectID>
in the code below with your company's Project ID, which can be found in the Descope console.
Run the following commands in your project
These commands will add the Descope NodeJS SDK as a project dependency.
npm i --save @descope/node-sdk
Import and initialize the ExpresSDK for NodeJS client in your source code
import DescopeClient from '@descope/node-sdk';
const descopeClient = DescopeClient({ projectId: <ProjectID> });
or
```javascript
const sdk = require('@descope/node-sdk');
const descopeClient = sdk({ projectId: <ProjectID> });
```
In your sign-up route using magic link (for example, myapp.com/signup
) generate a sign-up request and send the magic link via the selected delivery method. In the example below an email is sent to "mytestmail@test.com" containing the magic link and the link will automatically return back to the provided URL ("https://mydomain.com/verify"). In additon, optional user data (for exmaple, a custom username in the code sample below) can be gathered during the sign-up process.
await descopeClient.magiclink.signUp.email("mytestmail@test.com", { name: "custom name" })
In your sign-in route using magic link (for exmaple, myapp.com/login
) generate a sign-in request send the magic link via the selected delivery method. In the example below an email is sent to "mytestmail@test.com" containing the magic link and the link will automatically return back to the provided URL ("https://mydomain.com/verify").
await descopeClient.magiclink.signIn.email("mytestmail@test.com")
In your verify customer route for magic link (for example, mydomain.com/verify
) verify the token from either a customer sign-up or sign-in.
const out = await descopeClient.magiclink.verify(token)
if (out.data.cookies) {
res.set('Set-Cookie', out.data.cookies)
}
Session validation checks to see that the visitor to your website or application is who they say they are, by comparing the value in the validation variables against the session data that is already stored.
const out = await descopeClient.validateSession(session_jwt, refresh_jwt)
if (out?.cookies) {
res.set('Set-Cookie', out.cookies)
}
In the example below, we assume using the Descope builtin oauth provider, in that case, we dont need to define any specific application details.
Replace any instance of <ProjectID>
in the code below with your company's Project ID, which can be found in the Descope console.
Run the following commands in your project
These commands will add the Descope NodeJS SDK as a project dependency.
npm i --save @descope/node-sdk
Import and initialize the ExpresSDK for NodeJS client in your source code
import DescopeClient from '@descope/node-sdk';
const descopeClient = DescopeClient({ projectId: <ProjectID> });
or
```javascript
const sdk = require('@descope/node-sdk');
const descopeClient = sdk({ projectId: <ProjectID> });
```
In your OAuth start flow (for example, myapp.com/login-with-facebook
) generate a url to redirect the user to. In the example below the login
const out = await descopeClient.oauth.start.facebook();
return out.data.url;
In your exchange for any of the oauth provider (for example, mydomain.com/exchange
) verify the code from the provider by using the exchange method.
const code = req.query.code
const out = await descopeClient.oauth.exchnage(code);
if (out.data.cookies) {
res.set('Set-Cookie', out.data.cookies);
}
Session validation checks to see that the visitor to your website or application is who they say they are, by comparing the value in the validation variables against the session data that is already stored.
const out = await descopeClient.validateSession(session_jwt, refresh_jwt);
if (out?.cookies) {
res.set('Set-Cookie', out.cookies);
}
Instantly run the end-to-end ExpresSDK for NodeJS examples, as shown below. The source code for these examples are in the folder GitHub node-sdk/examples folder.
Run the following commands in your project. Replace any instance of <ProjectID>
in the code below with your company's Project ID, which can be found in the Descope console.
This commands will add the Descope NodeJS SDK as a project dependency, clone the SDK repository locally, and set the DESCOPE_PROJECT_ID
.
git clone github.com/descope/node-sdk
export DESCOPE_PROJECT_ID=<ProjectID>
TL;DR: Run npm run quick
Run this command in the root of the project to build the examples.
npm i
npm run build
cd examples/es6
npm i
npm start
Run a specific example
npm i
npm run build
cd examples/commonjs
npm i
npm start
The Descope ExpresSDK for Go is licensed for use under the terms and conditions of the MIT license Agreement.
FAQs
Node.js library used to integrate with Descope
The npm package @descope/node-sdk receives a total of 17,217 weekly downloads. As such, @descope/node-sdk popularity was classified as popular.
We found that @descope/node-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.