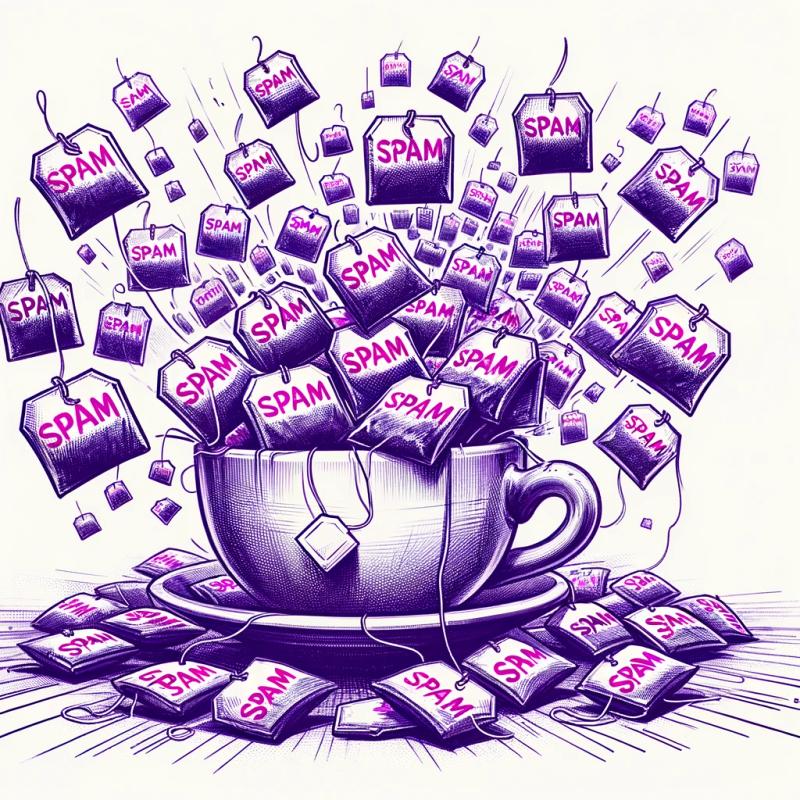
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@desmart/queue
Advanced tools
Readme
This package provides a unified API across a variety of different queue backends.
npm i @desmart/queue
const { manager, job } = require('@desmart/queue')
const { syncConnector } = require('@desmart/queue/connector')
const queue = manager(syncConnector())
// syncConnector will dispatch job immediately
// in this case it's required to attach listeners before job is pushed to queue
queue.handle('example.job', ({ name, queue, payload, attempts }) => {
console.log(name) // example.job
console.log(queue) // default
console.log(payload) // { foo: 'bar' }
console.log(attempts) // 1
})
queue.push(job.of('example.job', { foo: 'bar' }))
Job contains information regarding task that should be handled:
name
(String) name of the jobqueue
(String) name of the queue on which message had been sentattempts
(Number)payload
(Object)remove()
(Function) remove message from the queue backend; has to be triggered once job is processedrelease(delay = 0)
(Function) put job back to queue backend; optionally it can be delayed by given number of secondsTo create Job instance you can use .of()
static method:
const { job } = require('@desmart/queue')
job.of(name, payload, queue)
payload
and queue
are optional. By default job will use default
queue.
Each job received by queue backend will be dispatched to it's handler. Job can have only one dedicated handler.
queue.handle('example.job', ({ name, queue, payload, attempts }) => {
console.log(name) // example.job
console.log(queue) // default
console.log(payload) // { foo: 'bar' }
console.log(attempts) // 1
})
Every job can be pushed through manager to queue backend:
queue.push(job.of('example.job', { foo: 'bar' }))
By default manager will not listen for incoming jobs.
To start listening for new jobs it's required to call listen()
method.
Listener will wait for new queue messages, convert them to Job
object and pass it to bound handler.
By default listener will check for messages in default
queue.
manager.listen(queue)
Queue manager uses connectors to handle various queue backends.
This package provides two basic connectors:
syncConnector
fires immediately pushed to queue jobnullConnector
drops all pushed to queue jobsEach connector has to implement following methods:
onJob(fn)
accepts callback which should receive Job
instance once new message is retrieved from backendpush(job)
push job to queue backendIt's possible to extend behaviour of manager with middlewares.
Middleware is a function which should accept job
and next
callback. It's triggered once a job is fetched from backend and redirected to handler.
Through middleware it's possible to modify job (note that job is immutable), or do some other stuff. Don't forget to call next
once you want to pass control to another middleware.
Every middleware should (if possible) return the result of next()
. Remember also that other middlewares may return a Promise so async/await
may be useful here.
const { manager, job } = require('@desmart/queue')
const { autoCommit } = require('@desmart/queue/middleware')
const { syncConnector } = require('@desmart/queue/connector')
const queue = manager(syncConnector())
queue.use(autoCommit())
// each handle will be converted to terminating middleware - add them after all middlewares
queue.handle('job', () => {})
Package comes with some bundled middlewares. They can be imported from @desmart/queue/middleware
module.
autoCommit
const { autoCommit } = require('@desmart/queue/middleware')
queue.use(autoCommit({
exponential: true,
maxDelay: 6 * 3600
}))
Waits for job to finish and removes it from queue. If job failed it will be released back to queue.
This will works only when job handler returns a Promise.
Job is released with exponential delay. After first attempt it will be released without a delay, with second attempt it will be delayed by 5 seconds, later by 15 seconds and so on.. By defualt, after multiple fails, job will be delayed by 6 hours.
Available options:
exponential
(Boolean) [true
] should failed job be released with exponential delaymaxDelay
(Integer) [21600
] maximum delay for failed jobs; used only when exponential == true
maxAttempts
const { maxAttempts } = require('@desmart/queue/middleware')
queue.use(maxAttempts(max = 3))
Removes automatically a job which failed more than max
times.
debug
const { debug } = require('@desmart/queue/middleware')
queue.use(debug())
Small utility which uses debug to print information about processed job status.
This middleware has to be used before autoCommit
.
If you're planning to contribute to the package please make sure to adhere to following conventions.
npm run lint
to check if there are any linting errorsWe're not looking back! You are encouraged to use all features from ES6.
This package follows functional approach - if possible use pure functions, avoid classes etc.
FAQs
Message Queue client abstraction
The npm package @desmart/queue receives a total of 4 weekly downloads. As such, @desmart/queue popularity was classified as not popular.
We found that @desmart/queue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.