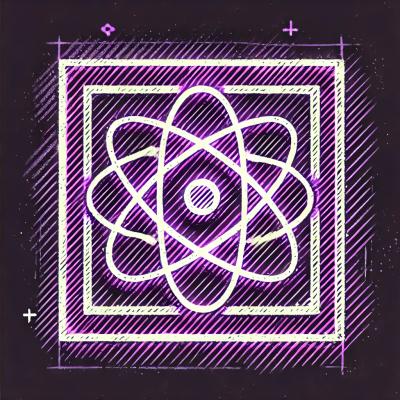
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@didomi/react
Advanced tools
Didomi React is a React component which creates a layer on top of our SDK.
$ npm install --save @didomi/react
import { DidomiSDK } from '@didomi/react';
Please note that the sooner you instanciate the component, the faster the banner will be displayed or the faster the consents will be shared with your partners and the ads displayed.
const didomiConfig = {
website: {
vendors: {
iab: {
all: true
}
}
}
}
...
<DidomiSDK
config={didomiConfig}
gdprAppliesGlobally={true}
onReady={didomi => console.log('Didomi SDK is loaded and ready', didomi)}
onConsentChanged={cwtToken => console.log('A consent has been given/withdrawn', cwtToken)}
onNoticeShown={() => console.log('Didomi Notice Shown')}
onNoticeHidden={() => console.log('Didomi Notice Hidden')}
/>
Name | Type | Default | Description |
---|---|---|---|
config | object | {} | Configuration of the SDK, please go below to see the configuration object structure |
gdprAppliesGlobally | boolean | true | The banner should display to all users no matter where they are located. If you are a non EU-based company then you are only required to collect consent and show the banner to EU visitors and can configure the banner to do so by changing the gdprAppliesGlobally variable to false in the tag above (that variable is separate from the window.didomiConfig variable). Please note that if you are an EU-based company then you must collect consent and display the banner to all visitors, no matter where they are from. |
onReady | function | Called when the SDK is loaded. Pass the Didomi object as parameter. Please see the https://developers.didomi.io/cmp/web-sdk/reference for more information about what you can do with the Didomi object | |
onConsentChanged | function | Called when a consent is given or withdrawn by the user. Pass the CWT Token as parameter. | |
onNoticeShown | function | Called when the notice is shown | |
onNoticeHidden | function | Called when the notice is hidden |
This is the structure of the configuration object. For more information, please visit our SDK documentation
{
website: {
ignoreCountry: true,
privacyPolicyURL: 'http://example.com',
name: 'Example',
apiKey: '<Your API key>',
logoUrl: 'http://logo.png',
vendors: {
iab: { // You either choose the option 'all', with optionaly 'exclude', or the 'include' option where you add the vendors manually
all: true,
exclude: [1],
// OR
include: [3],
},
didomi: ['google'],
custom: [
{
id: 'custom-vendor', // Unique ID for the vendor
name: 'Custom Vendor', // Display name of the vendor
purposeIds: ['cookies'], // List of purposes that you want to collect consent for, for this vendor
policyUrl: 'http://www.vendor.com/privacy-policy' // URL to the privacy policy of the vendor
}
]
},
customPurposes: [
{
id: 'my_custom_purpose',
name: {
en: 'My custom purpose',
},
description: {
en: 'Description',
}
}
]
},
languages: {
enabled: ['en', 'fr', 'es', 'nl', 'ca', 'it', 'de', 'pt'], // List of languages that visitors can use
default: 'fr', // Default language to use if the visitor uses a language that is not enabled
},
notice: {
position: 'popup',
closeOnClick: true,
daysBeforeShowingAgain: 0,
content: {
popup: {
en: 'Text',
},
notice: {
en: 'Text',
},
dismiss: {
en: 'Text',
},
learnMore: {
en: 'Text',
}
}
},
preferences: {
defaultChoice: true,
content: {
text: {
en: 'Text',
},
title: {
en: 'Text',
},
disagreeToAll: {
en: 'Text',
},
agreeToAll: {
en: 'Text',
},
save: {
en: 'Text',
},
textVendors: {
en: 'Text'
},
subTextVendors: {
en: 'Text'
}
},
information: {
enable: true,
content: {
text: {
en: 'Text',
},
learnMore: {
en: 'Text',
},
agreeAndClose: {
en: 'Text',
}
}
}
},
theme: {
color: '#3F51B5', // Principal color used by the SDK
font: 'Arial', // Font used by the SDK
buttons: {
regularButtons: { // Learn more/disagree/disagree to all buttons.
backgroundColor: '#eeeeee',
textColor: '#999999',
borderColor: 'rgba(34, 34, 34, 0.2)',
borderWidth: '1px',
borderRadius: '0px',
},
highlightButtons: { // Agree/save/agree to all buttons.
backgroundColor: 'rgb(194, 39, 45)',
textColor: '#ffffff',
borderColor: 'rgba(194, 39, 45, 0.3)',
borderWidth: '1px',
borderRadius: '0px',
}
}
},
tagManager: {
provider: 'gtm'
},
integrations: {
vendors: { // Setup the integration with Google (ask Didomi to share consent with Google tags)
google: {
enable: true,
eprivacy: true
}
},
refreshOnConsent: true // by default, ads are reloaded after consent is given
}
import React, { Component } from 'react'
import { DidomiSDK } from '@didomi/react'
/**
* This is the configuration object that will set the Didomi SDK
*/
const didomiConfig = {
website: {
name: 'Didomi',
apiKey: 'API_KEY',
vendors: {
iab: {
all: true
}
}
},
notice: {
position: 'bottom'
},
languages: {
enabled: ['fr', 'en', 'es'],
default: 'fr'
}
}
class DidomiDemo extends Component {
constructor(props) {
super(props);
this.didomiObject = {};
}
/**
* Called once we have the callback from the SDK informing that Didoni is loaded and ready
*/
onDidomiReady(didomi) {
this.didomiObject = didomi;
console.log('Didomi Ready - Is the consent required ? : ', this.didomiObject.isConsentRequired());
console.log('Didomi Ready - Do we have the consent for the vendor IAB 1 : ', this.didomiObject.getUserConsentStatusForVendor(1));
console.log('Didomi Ready - Do we have the consent for the vendor IAB 1 and the purpose cookies : ', this.didomiObject.getUserConsentStatus('cookies', 1));
}
/**
* Called everytime the consent changes
*/
consentHasChanged(cwtToken) {
console.log('Didomi Consent Changed - cwtToken : ', cwtToken);
console.log('Didomi Consent Changed - Is the consent required ? : ', this.didomiObject.isConsentRequired());
console.log('Didomi Consent Changed - Do we have the consent for the vendor IAB 1 : ', this.didomiObject.getUserConsentStatusForVendor(1));
console.log('Didomi Consent Changed - Do we have the consent for the vendor IAB 1 and the purpose cookies : ', this.didomiObject.getUserConsentStatus('cookies', 1));
}
render() {
return <div>
<h1>Didomi React Demo</h1>
<DidomiSDK
config={didomiConfig}
gdprAppliesGlobally={true}
onReady={this.onDidomiReady.bind(this)}
onConsentChanged={this.consentHasChanged.bind(this)}
/>
<button onClick={() => this.didomiObject.setUserAgreeToAll()}>Set Agree to All</button>
</div>
}
}
See Documentation
MIT
FAQs
didomi-react-test React component
The npm package @didomi/react receives a total of 19,762 weekly downloads. As such, @didomi/react popularity was classified as popular.
We found that @didomi/react demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.