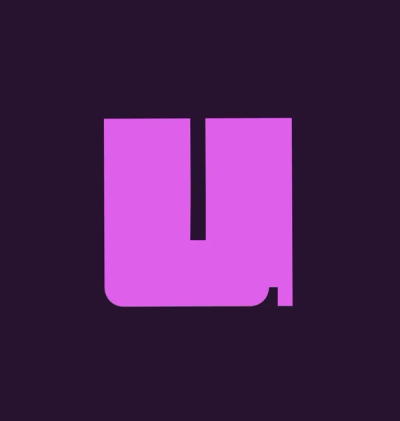
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@domoinc/ryuu-proxy
Advanced tools
a middleware that provides a proxy for local domo app development
Simple middleware to add to a local development server while developing Domo Apps. The middleware will intercept any calls to /data/v{d}
, /sql/v{d}
, /dql/v{d}
or /domo/.../v{d}
, proxy an authenticated request to the Domo App service, and pipe the response back so that you can develop your Domo App locally and still get request data from Domo.
npm install @domoinc/ryuu-proxy --save-dev
This library leverages the last login session from your Domo CLI. If that session is no longer active or doesn't exist then the proxy won't work. Be sure that you've logged in before you start working:
$ domo login
const { Proxy } = require('@domoinc/ryuu-proxy');
const manifest = require('./path/to/app/manifest.json');
const config = { manifest };
// use `proxy` in your development server
const proxy = new Proxy(config);
The proxy constructor expects a config
object. Certain properties are required and others are optional.
manifest
: The parsed contents of a project's manifest.json file. domo publish
have been run at least once to ensure the manifest.json
file has an id
propertymanifest.proxyId
: An advanced property required for projects leveraging DQL, writebacks, or Oauth. If you are unsure of whether or not you need this, you most likely don't. To get a proxyId, see "Getting a proxyId" belowThis library comes with a simple wrapper for Express/Connect middleware.
const app = express();
app.use(proxy.express());
For other frameworks, the library exposes the necessary functions to create a stream to pipe back to your server. You'll need to handle this stream as your server would expect. The only thing that stream()
expects is a standard Node Request which most server frameworks extend in some way.
// koa
app.use(async (ctx, next) => {
await proxy
.stream(ctx.req)
.then(data => ctx.body = ctx.req.pipe(data))
.catch(next);
});
// express
app.use((req, res, next) => {
proxy
.stream(req)
.then(stream => stream.pipe(res))
.catch(() => next());
});
// node http
const server = http.createServer((req, res) => {
if (req.url === '/') {
loadHomePage(res);
} else {
proxy
.stream(req)
.then(stream => stream.pipe(res));
}
});
Ignoring the errors will cause the proxy to fail silently and the proxy request will return a 404
error. If you'd like a little more detail on the errors you can expose them in the response:
// koa
app.use(async (ctx, next) => {
await proxy
.stream(ctx.req)
.then(data => ctx.body = ctx.req.pipe(data))
.catch((err) => {
if (err.name === 'DomoException') {
ctx.status = err.status || err.statusCode || 500;
ctx.body = err;
} else {
next();
}
});
});
// express / connect
app.use((req, res, next) => {
proxy
.stream(req)
.then(stream => stream.pipe(res))
.catch(err => {
if (err.name === 'DomoException') {
res.status(err.status || err.statusCode || 500).json(err);
} else {
next();
}
});
});
// http
const server = http.createServer((req, res) => {
if (req.url === '/') {
loadHomePage();
} else {
proxy
.stream(req)
.then(stream => stream.pipe(res))
.catch(err => {
if (err.name === 'DomoException') {
res.writeHead(err.status || err.statusCode || 500);
res.end(JSON.stringify(err));
}
});
}
});
Apps using DQL, writeback, or oAuth features are required to supply an proxyId as part of the proxy configuration. This allows the proxy to know how to properly route requests. The proxyId can be found as part of the URL for the iframe in which your app is displayed. It will be of the form XXXXXXXX-XXXX-4XXX-XXXX-XXXXXXXXXXXX
. To find the ID:
domo publish
<iframe>
that contains your app's code. The URL should be of the form //{HASH}.domoapps.prodX.domo.com?userId=...
//
and .domoapps
. That is your app's proxyId
proxyId
s tie apps to cards. If you delete the card from which you retrieved the proxyId, you will have to get a new one from another card created from your app design.
FAQs
a middleware that provides a proxy for local domo app development
The npm package @domoinc/ryuu-proxy receives a total of 373 weekly downloads. As such, @domoinc/ryuu-proxy popularity was classified as not popular.
We found that @domoinc/ryuu-proxy demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.