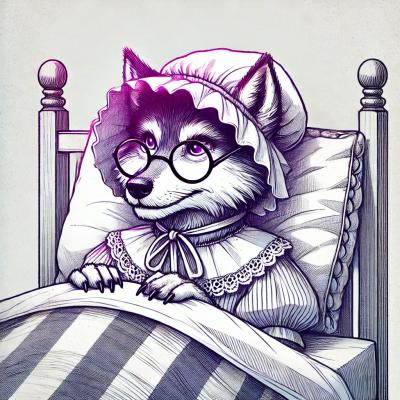
Security News
GitHub Removes Malicious Pull Requests Targeting Open Source Repositories
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
@dotcms/angular
Advanced tools
Official Angular Components library to render a dotCMS page.
@dotcms/angular
is the official Angular library designed to work seamlessly with dotCMS. This library simplifies the process of rendering dotCMS pages and integrating with the Universal Visual Editor in your Angular applications.
Install the package using npm:
npm install @dotcms/angular
Or using Yarn:
yarn add @dotcms/angular
We need to provide the information of our dotCMS instance
import { ClientConfig } from '@dotcms/client';
const DOTCMS_CLIENT_CONFIG: ClientConfig = {
dotcmsUrl: environment.dotcmsUrl,
authToken: environment.authToken,
siteId: environment.siteId
};
Add this configuration to ApplicationConfig
in your Angular app.
src/app/app.config.ts
import { InjectionToken } from '@angular/core';
import { ClientConfig, DotCmsClient } from '@dotcms/client';
export const DOTCMS_CLIENT_TOKEN = new InjectionToken<DotCmsClient>('DOTCMS_CLIENT');
export const appConfig: ApplicationConfig = {
providers: [
provideRouter(routes),
{
provide: DOTCMS_CLIENT_TOKEN,
useValue: DotCmsClient.init(DOTCMS_CLIENT_CONFIG),
}
],
};
This way, we will have access to DOTCMS_CLIENT_TOKEN
from anywhere in our application.
To interact with the client and obtain information from, for example, our pages
export class YourComponent {
private readonly client = inject(DOTCMS_CLIENT_TOKEN);
this.client.page
.get({ ...pageParams })
.then((response) => {
// Use your response
})
.catch((e) => {
const error: PageError = {
message: e.message,
status: e.status,
};
// Use the error response
})
}
For more information on how to use the dotCMS Client, you can visit the documentation
The DotcmsLayoutComponent
requires a DotCMSPageAsset
object to be passed in to it. This object represents a dotCMS page and can be fetched using the @dotcms/client
library.
The DotcmsLayoutComponent
is a crucial component for rendering dotCMS page layouts in your Angular application.
Name | Type | Description |
---|---|---|
pageAsset | DotCMSPageAsset | The object representing a dotCMS page from PageAPI response. |
components | DotCMSPageComponent | An object mapping contentlets to their respective render components. |
editor | EditorConfig | Configuration for data fetching in Edit Mode. |
In your component file (e.g., pages.component.ts
):
import { Component, signal } from '@angular/core';
import { DotCMSPageComponent, EditorConfig } from '@dotcms/angular';
@Component({
selector: 'app-pages',
templateUrl: './pages.component.html',
})
export class PagesComponent {
DYNAMIC_COMPONENTS: DotCMSPageComponent = {
Activity: import('../pages/content-types/activity/activity.component').then(
(c) => c.ActivityComponent
),
Banner: import('../pages/content-types/banner/banner.component').then(
(c) => c.BannerComponent
),
// Add other components as needed
};
components = signal(this.DYNAMIC_COMPONENTS);
editorConfig = signal<EditorConfig>({ params: { depth: 2 } });
// Assume pageAsset is fetched or provided somehow
pageAsset: DotCMSPageAsset;
}
In your template file (e.g., pages.component.html
):
<dotcms-layout
[pageAsset]="pageAsset"
[components]="components()"
[editor]="editorConfig()"
/>
This setup allows for dynamic rendering of different content types on your dotCMS pages.
If you encounter issues while using @dotcms/angular
, here are some common problems and their solutions:
Dependency Issues:
@dotcms/client
, @angular/core
, and rxjs
, are correctly installed and up-to-date. You can verify installed versions by running:
npm list @dotcms/client @angular/core rxjs
npm install
or
npm install --force
Configuration Errors:
DOTCMS_CLIENT_CONFIG
settings (URL, auth token, site ID) are correct and aligned with the environment variables. For example:
const DOTCMS_CLIENT_CONFIG: ClientConfig = {
dotcmsUrl: environment.dotcmsUrl, // Ensure this is a valid URL
authToken: environment.authToken, // Ensure auth token has the correct permissions
siteId: environment.siteId // Ensure site ID is valid and accessible
};
DOTCMS_CLIENT_TOKEN
is provided globally. Errors like NullInjectorError
usually mean the token hasn’t been properly added to the ApplicationConfig
. Verify by checking src/app/app.config.ts
.Network and API Errors:
dotcmsUrl
are successful. For CORS-related issues, ensure that your dotCMS server allows requests from your application’s domain.401 Unauthorized
errors, make sure the auth token used in DOTCMS_CLIENT_CONFIG
has appropriate permissions in dotCMS for accessing pages and content.Page and Component Rendering:
const DYNAMIC_COMPONENTS: DotCMSPageComponent = {
Activity: import('../pages/content-types/activity/activity.component').then(
(c) => c.ActivityComponent
)
};
pageAsset
objects are correctly formatted. Missing fields in pageAsset
can cause errors in DotcmsLayoutComponent
. Validate the structure by logging pageAsset
before passing it in.Common Angular Errors:
DotcmsLayoutComponent
isn’t updating as expected, you may need to call ChangeDetectorRef.detectChanges()
manually.DotCMSPageAsset
, DotCMSPageComponent
) are imported correctly from @dotcms/angular
. Type mismatches can often be resolved by verifying imports.Consult Documentation: Refer to the official dotCMS documentation and the @dotcms/angular GitHub repository. These sources often provide updates and additional usage examples.
GitHub pull requests are the preferred method to contribute code to dotCMS. Before any pull requests can be accepted, an automated tool will ask you to agree to the dotCMS Contributor's Agreement.
dotCMS comes in multiple editions and as such is dual licensed. The dotCMS Community Edition is licensed under the GPL 3.0 and is freely available for download, customization and deployment for use within organizations of all stripes. dotCMS Enterprise Editions (EE) adds a number of enterprise features and is available via a supported, indemnified commercial license from dotCMS. For the differences between the editions, see the feature page.
FAQs
Official Angular Components library to render a dotCMS page.
We found that @dotcms/angular demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
GitHub removed 27 malicious pull requests attempting to inject harmful code across multiple open source repositories, in another round of low-effort attacks.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.