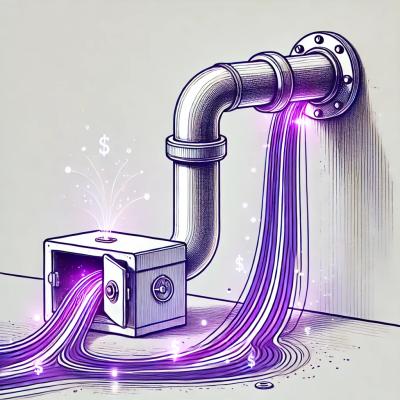
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@elastic/app-search-javascript
Advanced tools
A first-party JavaScript client for building excellent, relevant search experiences with Elastic App Search.
The easiest way to install this client is to simply include the built distribution from the jsDelivr CDN.
<script src="https://cdn.jsdelivr.net/npm/@elastic/app-search-javascript@8.13.0/dist/elastic_app_search.umd.js"></script>
This will make the client available globally at:
window.ElasticAppSearch;
This package can also be installed with npm
or yarn
.
npm install --save @elastic/app-search-javascript
The client could then be included into your project like follows:
// CommonJS
var ElasticAppSearch = require("@elastic/app-search-javascript");
// ES
import * as ElasticAppSearch from "@elastic/app-search-javascript";
This client is versioned and released alongside App Search.
To guarantee compatibility, use the most recent version of this library within the major version of the corresponding App Search implementation.
For example, for App Search 7.3
, use 7.3
of this library or above, but not 8.0
.
If you are using the SaaS version available on swiftype.com of App Search, you should use the version 7.5.x of the client.
The client is compatible with all modern browsers.
Note that this library depends on the Fetch API.
This is not supported by Internet Explorer. If you need backwards compatibility for Internet Explorer, you'll need to polyfill the Fetch API with something like https://github.com/github/fetch.
Using this client assumes that you have already an instance of Elastic App Search up and running.
The client is configured using the searchKey
, endpointBase
, and engineName
parameters.
var client = ElasticAppSearch.createClient({
searchKey: "search-mu75psc5egt9ppzuycnc2mc3",
endpointBase: "http://127.0.0.1:3002",
engineName: "favorite-videos"
});
* Please note that you should only ever use a Public Search Key within Javascript code on the browser. By default, your account should have a Key prefixed with search-
that is read-only. More information can be found in the documentation.
When using the SaaS version available on swiftype.com of App Search, you can configure the client using your hostIdentifier
instead of the endpointBase
parameter.
The hostIdentifier
can be found within the Credentials menu.
var client = ElasticAppSearch.createClient({
hostIdentifier: "host-c5s2mj",
searchKey: "search-mu75psc5egt9ppzuycnc2mc3",
engineName: "favorite-videos"
});
Option | Required | Description |
---|---|---|
hostIdentifier | No | Your Host Identifier, should start with host- . Required unless explicitly setting endpointBase |
searchKey | No | Your Public Search Key. It should start with search- .NOTE: This is not technically required, but in 99% of cases it should be provided. There is a small edge case for not providing this, mainly useful for internal App Search usage, where this can be ommited in order to leverage App Search's session based authentication. |
engineName | Yes | |
endpointBase | No | Overrides the base of the App Search API endpoint completely. Useful when proxying the App Search API, developing against a local server, or a Self-Managed or Cloud Deployment. Ex. "http://localhost:3002" |
cacheResponses | No | Whether or not API responses should be cached. Default: true . |
additionalHeaders | No | An Object with keys and values that will be converted to header names and values on all API requests |
This client is a thin interface to the Elastic App Search API. Additional details for requests and responses can be found in the documentation.
For the query term lion
, a search call is constructed as follows:
var options = {
search_fields: { title: {} },
result_fields: { id: { raw: {} }, title: { raw: {} } }
};
client
.search("lion", options)
.then(resultList => {
resultList.results.forEach(result => {
console.log(`id: ${result.getRaw("id")} raw: ${result.getRaw("title")}`);
});
})
.catch(error => {
console.log(`error: ${error}`);
});
Note that options
supports all options listed here: https://swiftype.com/documentation/app-search/guides/search.
In addition to the supported options above, we also support the following fields:
Name | Type | Description |
---|---|---|
disjunctiveFacets | Array[String] | An array of field names. Every field listed here must also be provided as a facet in the facet field. It denotes that a facet should be considered disjunctive. When returning counts for disjunctive facets, the counts will be returned as if no filter is applied on this field, even if one is applied. |
disjunctiveFacetsAnalyticsTags | Array[String] | Used in conjunction with the disjunctiveFacets parameter. Queries will be tagged with "Facet-Only" in the Analytics Dashboard unless specified here. |
Response
The search method returns the response wrapped in a ResultList
type:
ResultList {
rawResults: [], // List of raw `results` from JSON response
rawInfo: { // Object wrapping the raw `meta` property from JSON response
meta: {}
},
results: [ResultItem], // List of `results` wrapped in `ResultItem` type
info: { // Currently the same as `rawInfo`
meta: {}
}
}
ResultItem {
getRaw(fieldName), // Returns the HTML-unsafe raw value for a field, if it exists
getSnippet(fieldName) // Returns the HTML-safe snippet value for a field, if it exists
}
var options = {
size: 3,
types: {
documents: {
fields: ["name"]
}
}
};
client
.querySuggestion("cat", options)
.then(response => {
response.results.documents.forEach(document => {
console.log(document.suggestion);
});
})
.catch(error => {
console.log(`error: ${error}`);
});
It is possible to run multiple queries at once using the multiSearch
method.
To search for the term lion
and tiger
, a search call is constructed as follows:
var options = {
search_fields: { name: {} },
result_fields: { id: { raw: {} }, title: { raw: {} } }
};
client
.multiSearch([{ query: "node", options }, { query: "java", options }])
.then(allResults => {
allResults.forEach(resultList => {
resultList.results.forEach(result => {
console.log(
`id: ${result.getRaw("id")} raw: ${result.getRaw("title")}`
);
});
});
})
.catch(error => {
console.log(`error: ${error}`);
});
client
.click({
query: "lion",
documentId: "1234567",
requestId: "8b55561954484f13d872728f849ffd22",
tags: ["Animal"]
})
.catch(error => {
console.log(`error: ${error}`);
});
Clickthroughs can be tracked by binding client.click
calls to click events on individual search result links.
The following example shows how this can be implemented declaratively by annotating links with class and data attributes.
document.addEventListener("click", function(e) {
const el = e.target;
if (!el.classList.contains("track-click")) return;
client.click({
query: el.getAttribute("data-query"),
documentId: el.getAttribute("data-document-id"),
requestId: el.getAttribute("data-request-id"),
tags: [el.getAttribute("data-tag")]
});
});
<a
href="/items/1234567"
class="track-click"
data-request-id="8b55561954484f13d872728f849ffd22"
data-document-id="1234567"
data-query="lion"
data-tag="Animal"
>
Item 1
</a>
The specs in this project use node-replay to capture responses.
The responses are then checked against Jest snapshots.
To capture new responses and update snapshots, run:
nvm use
REPLAY=record npm run test -u
To run tests:
nvm use
npm run test
You will probably want to install a node version manager, like nvm.
We depend upon the version of node defined in .nvmrc.
To install and use the correct node version with nvm:
nvm install
Install dependencies:
nvm use
npm install
Build artifacts in dist
directory:
# This will create files in the `dist` directory
npm run build
Add an index.html
file to your dist
directory
<html>
<head>
<script src="elastic_app_search.umd.js"></script>
</head>
<body>
</body>
</html>
Run dev server:
# This will serve files in the `dist` directory
npm run dev
Navigate to http://127.0.0.1:8080 and execute JavaScript commands through the browser Dev Console.
nvm use
npm run build
nvm use
npm run publish
App Search has a first-party Node.js client which supports write operations like indexing.
If something is not working as expected, please open an issue.
Your best bet is to read the documentation.
You can checkout the Elastic App Search community discuss forums.
We welcome contributors to the project. Before you begin, a couple notes...
Thank you to all the contributors!
FAQs
Javascript client for the Elastic App Search Api
The npm package @elastic/app-search-javascript receives a total of 13,260 weekly downloads. As such, @elastic/app-search-javascript popularity was classified as popular.
We found that @elastic/app-search-javascript demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 62 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.