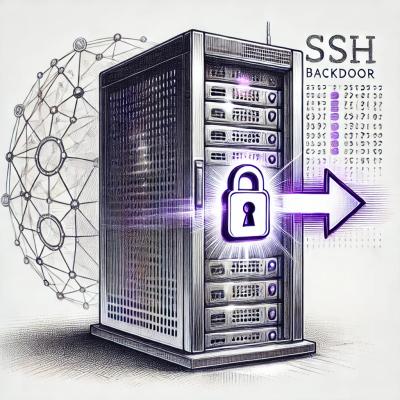
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@eld0ud/quickbooks-node-promise
Advanced tools
This library was created for Quickbooks OAuth2. It converted a lot of node-quickbooks into promises.
Will handle the authentication and auto renew Access Tokens when they are expired.
const QuickBooks = require("quickbooks-node-promise");
const qbo = new QuickBooks(appConfig, realmID);
const customers = await qbo.findCustomers({ Id: "1234" });
const customer = customer.QueryResponse.Customer[0]
console.log(`Hi my customer's name is ${customer.Name}`)
Check the example for node express setup endpoints
npm i quickbooks-node-promise
The store strategy is used to Save and Retreive token information. Both methods return a promise.
class QBStoreStrategy {
/**
* Uses a realmID to lookup the token information.
* Should return back in an object
*
* @param {number} realmID the quickbooks companyID
* @returns {object} Promise
*/
getQBToken({ realmID }) {
return new Promise((resolve) => {
// Get token infomraiton using realmID here
// Return object which includes the access_expire_timestamp & refresh_expire_timestamp
let newToken = {
realmID: my_realm_id // optional but nice to have
access_token: my_access_token,
refresh_token: my_refresh_token,
access_expire_timestamp: my_access_expire_timestamp,
refresh_expire_timestamp: my_refresh_expire_timestamp,
id_token: my_id_token // (Optional) Used only for user OpenID verification
}
resolve(newToken)
})
}
/**
* Used to store the new token information
* Will be looked up using the realmID
*
* @param {number} realmID the quickbooks companyID
* @param {object} token
* @param {string} token.access_token used to access quickbooks resource
* @param {string} token.refresh_token This should be securely stored
* @param {number} token.expires_in access_token expire time in seconds, 3600 usually
* @param {number} token.x_refresh_token_expires_in refresh_token expire time in seconds.
* @param {string} token.id_token (Optional) OpenID user token - sent only on original access, not included in refresh token
* @param {string} token.token_type This will be "Bearer"
* @param {object} access_expire_timestamp JS Date object when the access_token expires, calculated from expires_in
* @param {object} refresh_expire_timestamp JS Date object when the refresh_token expires, calculated from x_refresh_token_expires_in
* @returns {object} Promise
*/
storeQBToken({ realmID, token, access_expire_timestamp, refresh_expire_timestamp }) {
return new Promise((resolve) => {
// Store information to DB or your location here now
saveToDB({
realmID: realmID
access_token: token.access_token,
refresh_token: token.refresh_token,
access_expire_timestamp: access_expire_timestamp,
refresh_expire_timestamp: refresh_expire_timestamp,
id_token: my_id_token // (Optional) Used only for user OpenID verification
})
// Return object which includes the access_expire_timestamp & refresh_expire_timestamp
let newToken = {
realmID: my_realm_id
access_token: my_access_token,
refresh_token: my_refresh_token,
access_expire_timestamp: my_access_expire_timestamp,
refresh_expire_timestamp: my_refresh_expire_timestamp,
id_token: my_id_token // (Optional) Used only for user OpenID verification
}
resolve(newToken)
})
}
}
A config setup is needed for each app. Some values have defaults but should supply your own
// QB config
QBAppconfig = {
appKey: QB_APP_KEY,
appSecret: QB_APP_SECRET,
redirectUrl: QB_REDIRECT_URL,
minorversion: 37, /* default if ommited is 37, check for your version in the documents */
useProduction: QB_USE_PROD, /* default is false */
debug: (NODE_ENV == "production" ? false : true), /* default is false */
storeStrategy: new QBStoreStrategy(), // if ommited uses storage inside the created object
scope: [
QuickBooks.scopes.Accounting,
QuickBooks.scopes.OpenId,
QuickBooks.scopes.Profile,
QuickBooks.scopes.Email,
QuickBooks.scopes.Phone,
QuickBooks.scopes.Address
]
}
QuickBooks.scopes = {
Accounting: 'com.intuit.quickbooks.accounting',
Payment: 'com.intuit.quickbooks.payment',
Payroll: 'com.intuit.quickbooks.payroll',
TimeTracking: 'com.intuit.quickbooks.payroll.timetracking',
Benefits: 'com.intuit.quickbooks.payroll.benefits',
Profile: 'profile',
Email: 'email',
Phone: 'phone',
Address: 'address',
OpenId: 'openid',
Intuit_name: 'intuit_name'
}
Kind: global class
string
object
Token
Token
string
object
boolean
boolean
boolean
Node.js client encapsulating access to the QuickBooks V3 Rest API. An instance of this class should be instantiated on behalf of each user and company accessing the api.
Param | Description |
---|---|
appConfig | application information |
realmID | QuickBooks companyId, returned as a request parameter when the user is redirected to the provided callback URL following authentication |
string
Redirect link to Authorization Page
Kind: instance method of QuickBooks
Returns: string
- authorize Uri
object
Creates new token for the realmID from the returned authorization code received in the callback request
Kind: instance method of QuickBooks
Returns: object
- new token with expiration dates from storeStrategy
Param | Type | Description |
---|---|---|
authCode | string | The code returned in your callback as a param called "code" |
realmID | number | The company identifier in your callback as a param called "realmId" |
Save token
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
token | object | the token to send to store area |
Get token
Kind: instance method of QuickBooks
Token
Use the refresh token to obtain a new access token.
Kind: instance method of QuickBooks
Returns: Token
- returns fresh token with access_token and refresh_token
Param | Type | Description |
---|---|---|
token | Token | has the refresh_token |
Token
Use the refresh token to obtain a new access token.
Kind: instance method of QuickBooks
Returns: Token
- returns fresh token with access_token and refresh_token
Use either refresh token or access token to revoke access (OAuth2).
Kind: instance method of QuickBooks
Param | Description |
---|---|
useRefresh | boolean - Indicates which token to use: true to use the refresh token, false to use the access token. |
Validate id_token
Kind: instance method of QuickBooks
get Public Key
Kind: instance method of QuickBooks
Param |
---|
modulus |
exponent |
Get user info (OAuth2).
Kind: instance method of QuickBooks
Batch operation to enable an application to perform multiple operations in a single request. The following batch items are supported: create update delete query The maximum number of batch items in a single request is 25.
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
items | object | JavaScript array of batch items |
The change data capture (CDC) operation returns a list of entities that have changed since a specified time.
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
entities | object | Comma separated list or JavaScript array of entities to search for changes |
since | object | number | string | JS Date object, JS Date milliseconds, or string in ISO 8601 - to look back for changes until |
Uploads a file as an Attachable in QBO, optionally linking it to the specified QBO Entity.
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
filename | string | the name of the file |
contentType | string | the mime type of the file |
stream | object | ReadableStream of file contents |
entityType | object | optional string name of the QBO entity the Attachable will be linked to (e.g. Invoice) |
entityId | object | optional Id of the QBO entity the Attachable will be linked to |
Creates the Account in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
account | object | The unsaved account, to be persisted in QuickBooks |
Creates the Attachable in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
attachable | object | The unsaved attachable, to be persisted in QuickBooks |
Creates the Bill in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
bill | object | The unsaved bill, to be persisted in QuickBooks |
Creates the BillPayment in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
billPayment | object | The unsaved billPayment, to be persisted in QuickBooks |
Creates the Class in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
class | object | The unsaved class, to be persisted in QuickBooks |
Creates the CreditMemo in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
creditMemo | object | The unsaved creditMemo, to be persisted in QuickBooks |
Creates the Customer in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
customer | object | The unsaved customer, to be persisted in QuickBooks |
Creates the Department in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
department | object | The unsaved department, to be persisted in QuickBooks |
Creates the Deposit in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
deposit | object | The unsaved Deposit, to be persisted in QuickBooks |
Creates the Employee in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
employee | object | The unsaved employee, to be persisted in QuickBooks |
Creates the Estimate in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
estimate | object | The unsaved estimate, to be persisted in QuickBooks |
Creates the Invoice in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
invoice | object | The unsaved invoice, to be persisted in QuickBooks |
Creates the Item in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
item | object | The unsaved item, to be persisted in QuickBooks |
Creates the JournalCode in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
journalCode | object | The unsaved journalCode, to be persisted in QuickBooks |
Creates the JournalEntry in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
journalEntry | object | The unsaved journalEntry, to be persisted in QuickBooks |
Creates the Payment in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
payment | object | The unsaved payment, to be persisted in QuickBooks |
Creates the PaymentMethod in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
paymentMethod | object | The unsaved paymentMethod, to be persisted in QuickBooks |
Creates the Purchase in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
purchase | object | The unsaved purchase, to be persisted in QuickBooks |
Creates the PurchaseOrder in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
purchaseOrder | object | The unsaved purchaseOrder, to be persisted in QuickBooks |
Creates the RefundReceipt in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
refundReceipt | object | The unsaved refundReceipt, to be persisted in QuickBooks |
Creates the SalesReceipt in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
salesReceipt | object | The unsaved salesReceipt, to be persisted in QuickBooks |
Creates the TaxAgency in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
taxAgency | object | The unsaved taxAgency, to be persisted in QuickBooks |
Creates the TaxService in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
taxService | object | The unsaved taxService, to be persisted in QuickBooks |
Creates the Term in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
term | object | The unsaved term, to be persisted in QuickBooks |
Creates the TimeActivity in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
timeActivity | object | The unsaved timeActivity, to be persisted in QuickBooks |
Creates the Transfer in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
transfer | object | The unsaved Transfer, to be persisted in QuickBooks |
Creates the Vendor in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
vendor | object | The unsaved vendor, to be persisted in QuickBooks |
Creates the VendorCredit in QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
vendorCredit | object | The unsaved vendorCredit, to be persisted in QuickBooks |
Retrieves the Account from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Account |
Retrieves the Attachable from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Attachable |
Retrieves the Bill from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Bill |
Retrieves the BillPayment from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent BillPayment |
Retrieves the Class from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Class |
Retrieves the CompanyInfo from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent CompanyInfo |
Retrieves the CreditMemo from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent CreditMemo |
Retrieves the Customer from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Customer |
Retrieves the Department from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Department |
Retrieves the Deposit from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Deposit |
Retrieves the Employee from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Employee |
Retrieves the Estimate from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Estimate |
Retrieves an ExchangeRate from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | An object with options including the required sourcecurrencycode parameter and optional asofdate parameter. |
Retrieves the Estimate PDF from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Estimate |
Emails the Estimate PDF from QuickBooks to the address supplied in Estimate.BillEmail.EmailAddress or the specified 'sendTo' address
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Estimate |
sendTo | string | optional email address to send the PDF to. If not provided, address supplied in Estimate.BillEmail.EmailAddress will be used |
Retrieves the Invoice from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Invoice |
Retrieves the Invoice PDF from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Invoice |
Emails the Invoice PDF from QuickBooks to the address supplied in Invoice.BillEmail.EmailAddress or the specified 'sendTo' address
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Invoice |
sendTo | string | optional email address to send the PDF to. If not provided, address supplied in Invoice.BillEmail.EmailAddress will be used |
Retrieves the Item from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Item |
Retrieves the JournalCode from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent JournalCode |
Retrieves the JournalEntry from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent JournalEntry |
Retrieves the Payment from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Payment |
Retrieves the PaymentMethod from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent PaymentMethod |
Retrieves the Preferences from QuickBooks
Kind: instance method of QuickBooks
Retrieves the Purchase from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Purchase |
Retrieves the PurchaseOrder from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent PurchaseOrder |
Retrieves the RefundReceipt from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent RefundReceipt |
Retrieves the Reports from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Reports |
Retrieves the SalesReceipt from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent SalesReceipt |
Retrieves the SalesReceipt PDF from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent SalesReceipt |
Emails the SalesReceipt PDF from QuickBooks to the address supplied in SalesReceipt.BillEmail.EmailAddress or the specified 'sendTo' address
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent SalesReceipt |
sendTo | string | optional email address to send the PDF to. If not provided, address supplied in SalesReceipt.BillEmail.EmailAddress will be used |
Retrieves the TaxAgency from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent TaxAgency |
Retrieves the TaxCode from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent TaxCode |
Retrieves the TaxRate from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent TaxRate |
Retrieves the Term from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Term |
Retrieves the TimeActivity from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent TimeActivity |
Retrieves the Transfer from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Term |
Retrieves the Vendor from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent Vendor |
Retrieves the VendorCredit from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Id | string | The Id of persistent VendorCredit |
Updates QuickBooks version of Account
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
account | object | The persistent Account, including Id and SyncToken fields |
Updates QuickBooks version of Attachable
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
attachable | object | The persistent Attachable, including Id and SyncToken fields |
Updates QuickBooks version of Bill
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
bill | object | The persistent Bill, including Id and SyncToken fields |
Updates QuickBooks version of BillPayment
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
billPayment | object | The persistent BillPayment, including Id and SyncToken fields |
Updates QuickBooks version of Class
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
class | object | The persistent Class, including Id and SyncToken fields |
Updates QuickBooks version of CompanyInfo
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
companyInfo | object | The persistent CompanyInfo, including Id and SyncToken fields |
Updates QuickBooks version of CreditMemo
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
creditMemo | object | The persistent CreditMemo, including Id and SyncToken fields |
Updates QuickBooks version of Customer
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
customer | object | The persistent Customer, including Id and SyncToken fields |
Updates QuickBooks version of Department
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
department | object | The persistent Department, including Id and SyncToken fields |
Updates QuickBooks version of Deposit
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
deposit | object | The persistent Deposit, including Id and SyncToken fields |
Updates QuickBooks version of Employee
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
employee | object | The persistent Employee, including Id and SyncToken fields |
Updates QuickBooks version of Estimate
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
estimate | object | The persistent Estimate, including Id and SyncToken fields |
Updates QuickBooks version of Invoice
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
invoice | object | The persistent Invoice, including Id and SyncToken fields |
Updates QuickBooks version of Item
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
item | object | The persistent Item, including Id and SyncToken fields |
Updates QuickBooks version of JournalCode
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
journalCode | object | The persistent JournalCode, including Id and SyncToken fields |
Updates QuickBooks version of JournalEntry
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
journalEntry | object | The persistent JournalEntry, including Id and SyncToken fields |
Updates QuickBooks version of Payment
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
payment | object | The persistent Payment, including Id and SyncToken fields |
Updates QuickBooks version of PaymentMethod
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
paymentMethod | object | The persistent PaymentMethod, including Id and SyncToken fields |
Updates QuickBooks version of Preferences
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
preferences | object | The persistent Preferences, including Id and SyncToken fields |
Updates QuickBooks version of Purchase
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
purchase | object | The persistent Purchase, including Id and SyncToken fields |
Updates QuickBooks version of PurchaseOrder
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
purchaseOrder | object | The persistent PurchaseOrder, including Id and SyncToken fields |
Updates QuickBooks version of RefundReceipt
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
refundReceipt | object | The persistent RefundReceipt, including Id and SyncToken fields |
Updates QuickBooks version of SalesReceipt
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
salesReceipt | object | The persistent SalesReceipt, including Id and SyncToken fields |
Updates QuickBooks version of TaxAgency
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
taxAgency | object | The persistent TaxAgency, including Id and SyncToken fields |
Updates QuickBooks version of TaxCode
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
taxCode | object | The persistent TaxCode, including Id and SyncToken fields |
Updates QuickBooks version of TaxRate
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
taxRate | object | The persistent TaxRate, including Id and SyncToken fields |
Updates QuickBooks version of Term
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
term | object | The persistent Term, including Id and SyncToken fields |
Updates QuickBooks version of TimeActivity
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
timeActivity | object | The persistent TimeActivity, including Id and SyncToken fields |
Updates QuickBooks version of Transfer
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
Transfer | object | The persistent Transfer, including Id and SyncToken fields |
Updates QuickBooks version of Vendor
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
vendor | object | The persistent Vendor, including Id and SyncToken fields |
Updates QuickBooks version of VendorCredit
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
vendorCredit | object | The persistent VendorCredit, including Id and SyncToken fields |
Updates QuickBooks version of ExchangeRate
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
exchangeRate | object | The persistent ExchangeRate, including Id and SyncToken fields |
Deletes the Attachable from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Attachable to be deleted, or the Id of the Attachable, in which case an extra GET request will be issued to first retrieve the Attachable |
Deletes the Bill from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Bill to be deleted, or the Id of the Bill, in which case an extra GET request will be issued to first retrieve the Bill |
Deletes the BillPayment from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent BillPayment to be deleted, or the Id of the BillPayment, in which case an extra GET request will be issued to first retrieve the BillPayment |
Deletes the CreditMemo from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent CreditMemo to be deleted, or the Id of the CreditMemo, in which case an extra GET request will be issued to first retrieve the CreditMemo |
Deletes the Deposit from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Deposit to be deleted, or the Id of the Deposit, in which case an extra GET request will be issued to first retrieve the Deposit |
Deletes the Estimate from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Estimate to be deleted, or the Id of the Estimate, in which case an extra GET request will be issued to first retrieve the Estimate |
Deletes the Invoice from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Invoice to be deleted, or the Id of the Invoice, in which case an extra GET request will be issued to first retrieve the Invoice |
Deletes the JournalCode from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent JournalCode to be deleted, or the Id of the JournalCode, in which case an extra GET request will be issued to first retrieve the JournalCode |
Deletes the JournalEntry from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent JournalEntry to be deleted, or the Id of the JournalEntry, in which case an extra GET request will be issued to first retrieve the JournalEntry |
Deletes the Payment from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Payment to be deleted, or the Id of the Payment, in which case an extra GET request will be issued to first retrieve the Payment |
Deletes the Purchase from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Purchase to be deleted, or the Id of the Purchase, in which case an extra GET request will be issued to first retrieve the Purchase |
Deletes the PurchaseOrder from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent PurchaseOrder to be deleted, or the Id of the PurchaseOrder, in which case an extra GET request will be issued to first retrieve the PurchaseOrder |
Deletes the RefundReceipt from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent RefundReceipt to be deleted, or the Id of the RefundReceipt, in which case an extra GET request will be issued to first retrieve the RefundReceipt |
Deletes the SalesReceipt from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent SalesReceipt to be deleted, or the Id of the SalesReceipt, in which case an extra GET request will be issued to first retrieve the SalesReceipt |
Deletes the TimeActivity from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent TimeActivity to be deleted, or the Id of the TimeActivity, in which case an extra GET request will be issued to first retrieve the TimeActivity |
Deletes the Transfer from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Transfer to be deleted, or the Id of the Transfer, in which case an extra GET request will be issued to first retrieve the Transfer |
Deletes the VendorCredit from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent VendorCredit to be deleted, or the Id of the VendorCredit, in which case an extra GET request will be issued to first retrieve the VendorCredit |
Voids the Invoice from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
idOrEntity | object | The persistent Invoice to be voided, or the Id of the Invoice, in which case an extra GET request will be issued to first retrieve the Invoice |
Voids QuickBooks version of Payment
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
payment | object | The persistent Payment, including Id and SyncToken fields |
Finds all Accounts in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Attachables in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Bills in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all BillPayments in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Budgets in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Classs in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all CompanyInfos in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all CreditMemos in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Customers in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Departments in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Deposits in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Employees in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Estimates in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Invoices in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Items in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all JournalCodes in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all JournalEntrys in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Payments in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all PaymentMethods in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Preferencess in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Purchases in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all PurchaseOrders in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all RefundReceipts in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all SalesReceipts in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all TaxAgencys in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all TaxCodes in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all TaxRates in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Terms in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all TimeActivitys in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Transfers in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all Vendors in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all VendorCredits in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Finds all ExchangeRates in QuickBooks, optionally matching the specified criteria
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
criteria | object | (Optional) String or single-valued map converted to a where clause of the form "where key = 'value'" |
Retrieves the BalanceSheet Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the ProfitAndLoss Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the ProfitAndLossDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the TrialBalance Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the CashFlow Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the InventoryValuationSummary Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the CustomerSales Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the ItemSales Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the CustomerIncome Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the CustomerBalance Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the CustomerBalanceDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the AgedReceivables Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the AgedReceivableDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the VendorBalance Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the VendorBalanceDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the AgedPayables Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the AgedPayableDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the VendorExpenses Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the TransactionList Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the GeneralLedgerDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the TaxSummary Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the DepartmentSales Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the ClassSales Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
Retrieves the AccountListDetail Report from QuickBooks
Kind: instance method of QuickBooks
Param | Type | Description |
---|---|---|
options | object | (Optional) Map of key-value pairs passed as options to the Report |
string
Redirect link to Authorization Page
Kind: static method of QuickBooks
Returns: string
- authorize Uri
Param | Type | Description |
---|---|---|
appConfig | object | The config for your app |
object
Creates new token for the realmID from the returned authorization code received in the callback request
Kind: static method of QuickBooks
Returns: object
- new token with expiration dates from storeStrategy
Param | Type | Description |
---|---|---|
appConfig | object | The config for your app |
authCode | string | The code returned in your callback as a param called "code" |
realmID | number | The company identifier in your callback as a param called "realmId" |
boolean
Helper Method to check token expiry { set Token Object }
Kind: static method of QuickBooks
Param | Type | Description |
---|---|---|
expired_timestamp | object | number | string | JS Date object, JS Date milliseconds, or string in ISO 8601 - when token expires |
boolean
Check if access_token is valid
Kind: static method of QuickBooks
Returns: boolean
- token has expired or not
Param | Type | Description |
---|---|---|
token | object | returned from storeStrategy |
boolean
Check if there is a valid (not expired) access token
Kind: static method of QuickBooks
Returns: boolean
- token has expired or not
Param | Type | Description |
---|---|---|
token | object | returned from storeStrategy |
Save token
Kind: static method of QuickBooks
Param | Type | Description |
---|---|---|
store | storeStrategy | The store strategy class used for getting and setting the token |
info | object | the realmID and token to send to store area |
Get token
Kind: static method of QuickBooks
FAQs
Connect to QuickBooks Online API with OAuth 2
The npm package @eld0ud/quickbooks-node-promise receives a total of 35 weekly downloads. As such, @eld0ud/quickbooks-node-promise popularity was classified as not popular.
We found that @eld0ud/quickbooks-node-promise demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.