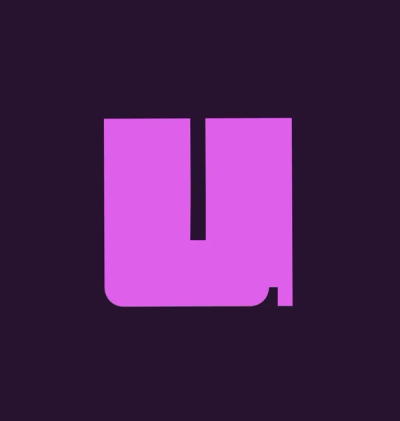
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
@endo/trampoline
Advanced tools
Multicolor trampolining using generators
@endo/trampoline is a utility library which helps share code between synchronous and asynchronous variations of the same algorithm.
import { asyncTrampoline, syncTrampoline } from '@endo/trampoline';
/**
* This function "reads a file synchronously" and returns "a list of its imports"
*
* @param {string} filepath Source file path
* @returns {string[]} List of imports found in source
*/
const findImportsSync = filepath => {
// read a file, parse it for imports, return a list of import specifiers
// (synchronously)
// ...
};
/**
* This function "reads a file asynchronously" and returns "a list of its imports"
*
* @param {string} filepath Source file path
* @returns {Promise<string[]>} List of imports found in source
*/
const findImportsAsync = async filepath => {
// read a file, parse it for imports, return a list of import specifiers
// (asynchronously)
// ...
};
/**
* Recursively crawls a dependency tree to find all dependencies
*
* @template {string[] | Promise<string[]>} TResult Type of result (list of imports)
* @param {(filepath: string) => TResult} finder Function which reads a file and returns its imports
* @param {string} filename File to start from; entry point
* @returns {Generator<TResult, string[], string[]>} Generator yielding list of imports
*/
function* findAllImports(finder, filename) {
// it doesn't matter if finder is sync or async!
let specifiers = yield finder(filename);
// pretend there's some de-duping, caching,
// scrubbing, etc. happening here
for (const specifier of specifiers) {
// it's okay to be recursive
specifiers = [...specifiers, ...(yield* findAllImports(finder, specifier))];
}
return specifiers;
}
// results are an array of all imports found in some.js' dependency tree
const asyncResult = await asyncTrampoline(
findAllImports,
findImports,
'./some.js',
);
// same thing, but synchronously
const syncResult = syncTrampoline(
findAllImports,
findImportsAsync,
'./some.js',
);
asyncResult === syncResult; // true
In the above example, @endo/trampoline allows us to re-use the operations in loadRecursive()
for both sync and async execution. An implementation without @endo/trampoline would need to duplicate the operations into two (2) discrete recursive functions—a synchronous-colored function and an asynchronous-colored function. Over time, this situation commonly leads to diverging implementations. If that doesn't sound like a big deal for whatever you're trying to do here, then you probably don't need @endo/trampoline.
The pattern exposed by this library—known as trampolining—helps manage control flow in a way that avoids deep recursion and potential stack overflows.
@endo/trampoline provides the trampolining pattern, but in such a way that a consumer can execute either synchronous or asynchronous operations paired with operations common to both.
In other words, @endo/trampoline can help reduce code duplication when operations must be executed in both sync and async contexts.
The usual sort of thing:
npm install @endo/trampoline
Apache-2.0
By using this library, you agree to indemnify and hold harmless the authors of @endo/trampoline
from any and all losses, liabilities and risk of bodily injury including but not limited to broken bones, sprains, bruises or other hematomas, fibromas, teratomas, mesotheliomas, cooties, bubonic plague, psychic trauma or warts due to the inherent danger of trampolining.
FAQs
Multicolor trampolining for recursive operations
The npm package @endo/trampoline receives a total of 3,066 weekly downloads. As such, @endo/trampoline popularity was classified as popular.
We found that @endo/trampoline demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.