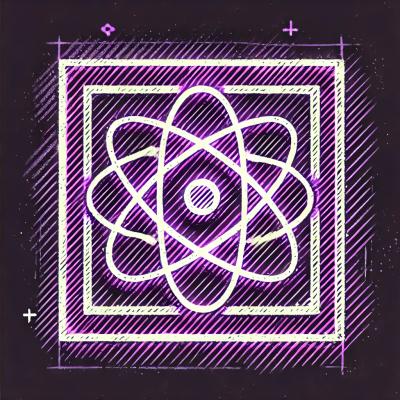
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@enmo-design/icons-vue
Advanced tools
npm install @enmo-design/icons-vue
<template>
<div>
<smile-outlined></smile-outlined>
<smile-outlined width="60" height="60"></smile-outlined>
<smile-outlined width="60" height="60" spin></smile-outlined>
<smile-outlined width="60" height="60" :rotate="45"></smile-outlined>
</div>
</template>
<script>
import { SmileOutlined } from '@enmo-design/icons';
export default {
components: { SmileOutlined }
}
</script>
Property | Description | Type | Default |
---|---|---|---|
width | The width of the svg element | string | number | '1em' |
height | The height of the svg element | string | number | '1em' |
rotate | Rotate degrees (not working in IE9) | number | - |
spin | Rotate icon with animation | boolean | false |
You can import SVG icon as an vue component by using vue cli 3 and vue-svg-loader. vue-svg-loader's options reference.
// vue.config.js
module.exports = {
chainWebpack: (config) => {
const svgRule = config.module.rule('svg')
svgRule.uses.clear()
svgRule
.use('babel-loader')
.loader('babel-loader')
.end()
.use('vue-svg-loader')
.loader('vue-svg-loader')
.options({
svgo: {
plugins: [{removeViewBox: false}]
}
})
}
}
<template>
<div>
<icon :component="MessageSvg"></icon>
<icon :component="MessageSvg" width="60" height="60"></icon>
<icon :component="MessageSvg" width="60" height="60" :rotate="45"></icon>
<icon :component="MessageSvg" width="60" height="60" spin></icon>
<icon :component="MessageSvg" width="60" height="60" @click="clickHandler"></icon>
</div>
</template>
<script>
import Icon from '@enmo-design/icons';
import MessageSvg from 'path/to/message.svg'; // path to your '*.svg' file.
export default {
components: { Icon },
data () {
return {
MessageSvg
}
}
}
</script>
Property | Description | Type | Default |
---|---|---|---|
component | SVG icon component load by vue-svg-loader | vue component | - |
width | The width of the svg element | string | number | '1em' |
height | The height of the svg element | string | number | '1em' |
rotate | Rotate degrees (not working in IE9) | number | - |
spin | Rotate icon with animation | boolean | false |
FAQs
Enmo Design Icons for Vue.
We found that @enmo-design/icons-vue demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.