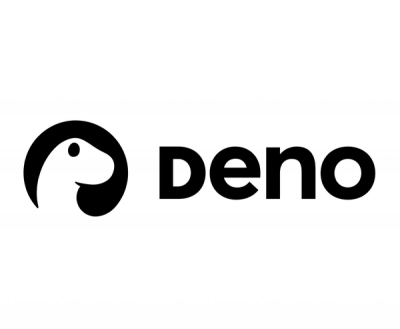
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@erickmerchant/framework
Advanced tools
This scoped package is my personal framework. I wrote it to understand how modern javascript frameworks do things. It's meant to be used with browserify.
This example uses yo-yo (which uses hyperx), but you should be able to use diffHTML and possibly other solutions.
const framework = require('@erickmerchant/framework')
const html = require('yo-yo')
const diff = html.update
const target = document.querySelector('main')
framework({target, store, component, diff})(init)
function init ({dispatch}) {
dispatch('increment')
}
function store (state = 0, action) {
switch (action) {
case 'increment': return state + 1
case 'decrement': return state - 1
}
return state
}
function component ({state, dispatch}) {
return html`<main>
<output>${state}</output>
<br>
<button onclick=${decrement}>--</button>
<button onclick=${increment}>++</button>
</main>`
function decrement () {
dispatch('decrement')
}
function increment () {
dispatch('increment')
}
}
framework({target, store, component, diff, raf})
The function exported by this module.
Returns a function. See init
This is what is returned from the store. It can be anything from simply a number like in the example, to a complex object.
dispatch(...arguments)
Initializes a change in state and causes a render
next(({target, dispatch}) => { ... })
A convenient helper to pass a callback to process.nextTick. Can be used to manipulate the page in some way after a render. For example scrolling to a specific element
init(({target, dispatch}) => { ... })
store(state, ...arguments)
Called initially with zero arguments, it should return the default/initial state.
component({state, dispatch, next})
Should return the element to pass to diff
diff(target, element)
A module to create a store from other stores where each is responsible for one property in the state.
A module to do routing inside your components.
The source code can be found here
FAQs
A front-end framework.
The npm package @erickmerchant/framework receives a total of 2 weekly downloads. As such, @erickmerchant/framework popularity was classified as not popular.
We found that @erickmerchant/framework demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.