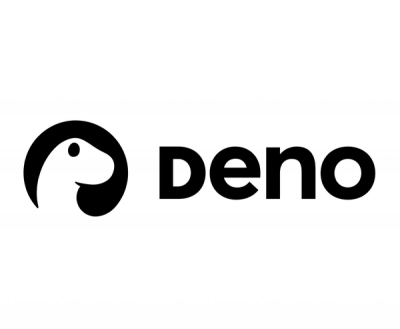
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@es-git/save-as-mixin
Advanced tools
This is part of the [ES-Git](https://github.com/es-git/es-git) project.
This is part of the ES-Git project.
npm install --save @es-git/save-as-mixin
Mix this in with an IObjectRepo.
This mixin makes it easier to save objects to the repo.
import objectsMixin from '@es-git/objects-mixin';
import saveAsMixin from '@es-git/save-as-mixin';
import MemoryRepo from '@es-git/memory-repo';
const Repo = mix(MemoryRepo)
.with(objectsMixin)
.with(saveAsMixin);
const repo = new Repo();
const hash = await repo.saveText('hello');
const tree = await repo.saveTree({
'file1.txt': { mode: Mode.File, hash }
});
interface ISaveAsRepo {
saveBlob(blob : Uint8Array) : Promise<Hash>
saveText(text : string) : Promise<Hash>
saveTree(tree : TreeBody) : Promise<Hash>
saveCommit(commit : CommitBody) : Promise<Hash>
saveTag(tag : TagBody) : Promise<Hash>
}
type Hash = string;
type TreeBody = {
[key : string] : ModeHash
}
type ModeHash = {
readonly mode : Mode
readonly hash : string
}
type CommitBody = {
readonly tree : string
readonly parents : string[]
readonly author : Person
readonly committer : Person
readonly message : string
}
type Person = {
readonly name : string
readonly email : string
readonly date : Date | SecondsWithOffset
}
type SecondsWithOffset = {
readonly seconds : number
readonly offset : number
}
type TagBody = {
readonly object : string
readonly type : string
readonly tag : string
readonly tagger : Person
readonly message : string
}
FAQs
This is part of the [ES-Git](https://github.com/es-git/es-git) project.
The npm package @es-git/save-as-mixin receives a total of 10 weekly downloads. As such, @es-git/save-as-mixin popularity was classified as not popular.
We found that @es-git/save-as-mixin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.