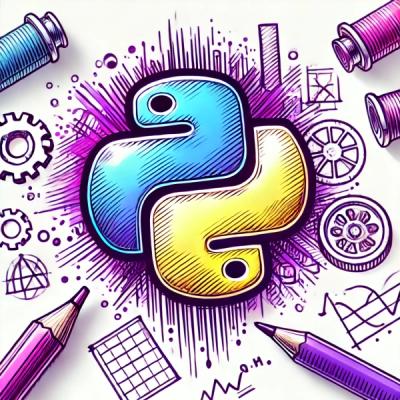
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
@fastify/bearer-auth
Advanced tools
@fastify/bearer-auth provides a simple request hook for the Fastify web framework.
'use strict'
const fastify = require('fastify')()
const bearerAuthPlugin = require('@fastify/bearer-auth')
const keys = new Set(['a-super-secret-key', 'another-super-secret-key'])
fastify.register(bearerAuthPlugin, {keys})
fastify.get('/foo', (req, reply) => {
reply.send({authenticated: true})
})
fastify.listen({port: 8000}, (err) => {
if (err) {
fastify.log.error(err.message)
process.exit(1)
}
fastify.log.info('http://127.0.0.1:8000/foo')
})
@fastify/bearer-auth exports a standard Fastify plugin. This allows you to register the plugin within scoped paths. Therefore, you could have some paths that are not protected by the plugin and others that are. See the Fastify documentation and examples for more details.
When registering the plugin you must specify a configuration object:
keys
: A Set
or array with valid keys of type string
(required)function errorResponse (err) {}
: method must synchronously return the content body to be
sent to the client (optional)contentType
: If the content to be sent is anything other than
application/json
, then the contentType
property must be set (optional)bearerType
: string specifying the Bearer string (optional)function auth (key, req) {}
: this function will test if key
is a valid token.
The function must return a literal true
if the key is accepted or a literal
false
if rejected. The function may also return a promise that resolves to
one of these values. If the function returns or resolves to any other value,
rejects, or throws, a HTTP status of 500
will be sent. req
is the Fastify
request object. If auth
is a function, keys
will be ignored. If auth
is
not a function, or undefined
, keys
will be used.addHook
: If false
, this plugin will not register onRequest
hook automatically,
instead it provide two decorations fastify.verifyBearerAuth
and
fastify.verifyBearerAuthFactory
for you.verifyErrorLogLevel
: An optional string specifying the log level when there is a verification error.
It must be a valid log level supported by fastify, otherwise an exception will be thrown
when registering the plugin. By default, this option is set to error
.The default configuration object is:
{
keys: new Set(),
contentType: undefined,
bearerType: 'Bearer',
errorResponse: (err) => {
return {error: err.message}
},
auth: undefined,
addHook: true
}
Internally, the plugin registers a standard Fastify preHandler hook,
which will inspect the request's headers for an authorization
header with the
format bearer key
. The key
will be matched against the configured keys
object via a constant time algorithm to prevent against timing-attacks. If the authorization
header is missing,
malformed, or the key
does not validate then a 401 response will be sent with
a {error: message}
body; no further request processing will be performed.
@fastify/auth
This plugin can integrate with @fastify/auth
by following this example:
const fastify = require('fastify')()
const auth = require('@fastify/auth')
const bearerAuthPlugin = require('@fastify/bearer-auth')
const keys = new Set(['a-super-secret-key', 'another-super-secret-key'])
async function server() {
await fastify
.register(auth)
.register(bearerAuthPlugin, { addHook: false, keys, verifyErrorLogLevel: 'debug' })
.decorate('allowAnonymous', function (req, reply, done) {
if (req.headers.authorization) {
return done(Error('not anonymous'))
}
return done()
})
fastify.route({
method: 'GET',
url: '/multiauth',
preHandler: fastify.auth([
fastify.allowAnonymous,
fastify.verifyBearerAuth
]),
handler: function (_, reply) {
reply.send({ hello: 'world' })
}
})
await fastify.listen({port: 8000})
}
server()
By passing { addHook: false }
in the options, the verifyBearerAuth
hook, instead of
immediately replying on error (reply.send(someError)
), invokes done(someError)
. This
will allow fastify.auth
to continue with the next authentication scheme in the hook list.
Note that by setting { verifyErrorLogLevel: 'debug' }
in the options, @fastify/bearer-auth
will emit all verification error logs at the debug
level. Since it is not the only authentication method here, emitting verification error logs at the error
level may be not appropriate here.
If verifyBearerAuth
is the last hook in the list, fastify.auth
will reply with Unauthorized
.
FAQs
A Bearer authentication plugin for Fastify
We found that @fastify/bearer-auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.