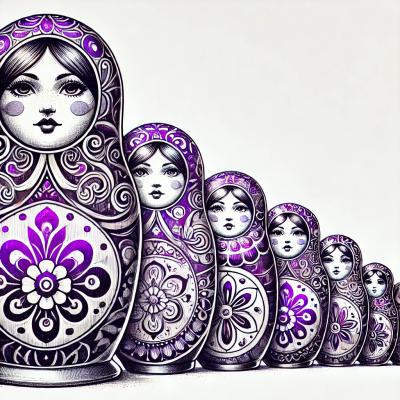
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@fastify/cookie
Advanced tools
@fastify/cookie is a Fastify plugin that provides cookie parsing and serialization capabilities. It allows you to easily handle cookies in your Fastify applications, including setting, getting, and deleting cookies.
Setting a Cookie
This feature allows you to set a cookie in the client's browser. The code sample demonstrates how to set a cookie with various options such as domain, path, secure, httpOnly, and sameSite.
const fastify = require('fastify')();
const fastifyCookie = require('@fastify/cookie');
fastify.register(fastifyCookie);
fastify.get('/set-cookie', (request, reply) => {
reply
.setCookie('myCookie', 'cookieValue', {
domain: 'example.com',
path: '/',
secure: true,
httpOnly: true,
sameSite: 'Strict'
})
.send({ hello: 'world' });
});
fastify.listen(3000, err => {
if (err) throw err;
console.log('Server listening on http://localhost:3000');
});
Getting a Cookie
This feature allows you to retrieve a cookie from the client's request. The code sample demonstrates how to access a cookie named 'myCookie' from the request object.
const fastify = require('fastify')();
const fastifyCookie = require('@fastify/cookie');
fastify.register(fastifyCookie);
fastify.get('/get-cookie', (request, reply) => {
const myCookie = request.cookies.myCookie;
reply.send({ myCookie });
});
fastify.listen(3000, err => {
if (err) throw err;
console.log('Server listening on http://localhost:3000');
});
Deleting a Cookie
This feature allows you to delete a cookie from the client's browser. The code sample demonstrates how to clear a cookie named 'myCookie' with a specified path.
const fastify = require('fastify')();
const fastifyCookie = require('@fastify/cookie');
fastify.register(fastifyCookie);
fastify.get('/delete-cookie', (request, reply) => {
reply
.clearCookie('myCookie', { path: '/' })
.send({ hello: 'world' });
});
fastify.listen(3000, err => {
if (err) throw err;
console.log('Server listening on http://localhost:3000');
});
cookie-parser is a middleware for Express that parses cookies attached to the client request object. It provides similar functionality to @fastify/cookie but is designed for use with Express applications.
cookies is a general-purpose cookie handling library for Node.js. It provides methods for setting, getting, and deleting cookies, and can be used with various web frameworks, including Express and Koa.
koa-cookie is a middleware for Koa that provides cookie parsing and serialization capabilities. It offers similar functionality to @fastify/cookie but is specifically designed for Koa applications.
A plugin for Fastify that adds support for reading and setting cookies.
This plugin's cookie parsing works via Fastify's onRequest
hook. Therefore,
you should register it prior to any other onRequest
hooks that will depend
upon this plugin's actions.
@fastify/cookie
v2.x
supports both Fastify@1 and Fastify@2.
@fastify/cookie
v3 only supports Fastify@2.
npm i @fastify/cookie
or
yarn add @fastify/cookie
const fastify = require('fastify')()
fastify.register(require('@fastify/cookie'), {
secret: "my-secret", // for cookies signature
parseOptions: {} // options for parsing cookies
})
fastify.get('/', (req, reply) => {
const aCookieValue = req.cookies.cookieName
// `reply.unsignCookie()` is also available
const bCookie = req.unsignCookie(req.cookies.cookieSigned);
reply
.setCookie('foo', 'foo', {
domain: 'example.com',
path: '/'
})
.cookie('baz', 'baz') // alias for setCookie
.setCookie('bar', 'bar', {
path: '/',
signed: true
})
.send({ hello: 'world' })
})
import type { FastifyCookieOptions } from '@fastify/cookie'
import cookie from '@fastify/cookie'
import fastify from 'fastify'
const app = fastify()
app.register(cookie, {
secret: "my-secret", // for cookies signature
parseOptions: {} // options for parsing cookies
} as FastifyCookieOptions)
secret
(String
| Array
| Object
):
String
can be passed to use as secret to sign the cookie using cookie-signature
.Array
can be passed if key rotation is desired. Read more about it in Rotating signing secret.Object
. Read more about it in Custom cookie signer.parseOptions
: An Object
to pass as options to cookie parse.
Cookies are parsed in the onRequest
Fastify hook and attached to the request
as an object named cookies
. Thus, if a request contains the header
Cookie: foo=foo
then, within your handler, req.cookies.foo
would equal
'foo'
.
You can pass options to the cookie parse by setting an object named parseOptions
in the plugin config object.
The method setCookie(name, value, options)
, and its alias cookie(name, value, options)
, are added to the reply
object
via the Fastify decorateReply
API. Thus, in a request handler,
reply.setCookie('foo', 'foo', {path: '/'})
will set a cookie named foo
with a value of 'foo'
on the cookie path /
.
name
: a string name for the cookie to be setvalue
: a string value for the cookieoptions
: an options object as described in the cookie serialize documentation
with a extra param "signed" for signed cookieFollowing are some of the precautions that should be taken to ensure the integrity of an application:
options.httpOnly
cookies to prevent attacks like XSS.options.signed
) to ensure they are not getting tampered with on client-side by an attacker.__Host-
Cookie Prefix to avoid Cookie Tossing attacks.The method clearCookie(name, options)
is added to the reply
object
via the Fastify decorateReply
API. Thus, in a request handler,
reply.clearCookie('foo', {path: '/'})
will clear a cookie named foo
on the cookie path /
.
name
: a string name for the cookie to be clearedoptions
: an options object as described in the cookie serialize
documentation. Its optional to pass options
objectThe method parseCookie(cookieHeader)
is added to the fastify
instance
via the Fastify decorate
API. Thus, fastify.parseCookie('sessionId=aYb4uTIhdBXC')
will parse the raw cookie header and return an object { "sessionId": "aYb4uTIhdBXC" }
.
Key rotation is when an encryption key is retired and replaced by generating a new cryptographic key. To implement rotation, supply an Array
of keys to secret
option.
Example:
fastify.register(require('@fastify/cookie'), {
secret: [key1, key2]
})
The plugin will always use the first key (key1
) to sign cookies. When parsing incoming cookies, it will iterate over the supplied array to see if any of the available keys are able to decode the given signed cookie. This ensures that any old signed cookies are still valid.
Note:
secret
array.Example:
fastify.get('/', (req, reply) => {
const result = reply.unsignCookie(req.cookies.myCookie)
if (result.valid && result.renew) {
// Setting the same cookie again, this time plugin will sign it with a new key
reply.setCookie('myCookie', result.value, {
domain: 'example.com', // same options as before
path: '/',
signed: true
})
}
})
The secret
option optionally accepts an object with sign
and unsign
functions. This allows for implementing a custom cookie signing mechanism. See the following example:
Example:
fastify.register(require('@fastify/cookie'), {
secret: {
sign: (value) => {
// sign using custom logic
return signedValue
},
unsign: (value) => {
// unsign using custom logic
return {
valid: true, // the cookie has been unsigned successfully
renew: false, // the cookie has been unsigned with an old secret
value: 'unsignedValue'
}
}
}
})
The method unsignCookie(value)
is added to the fastify
instance and the reply
object
via the Fastify decorate
& decorateReply
APIs. Using it on a signed cookie will call the
the provided signer's (or the default signer if no custom implementation is provided) unsign
method on the cookie.
Example:
fastify.register(require('@fastify/cookie'), { secret: 'my-secret' })
fastify.get('/', (req, rep) => {
if (fastify.unsign(req.cookie.foo).valid === false) {
rep.send('cookie is invalid')
return
}
rep.send('cookie is valid')
})
FAQs
Plugin for fastify to add support for cookies
The npm package @fastify/cookie receives a total of 241,069 weekly downloads. As such, @fastify/cookie popularity was classified as popular.
We found that @fastify/cookie demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 20 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.