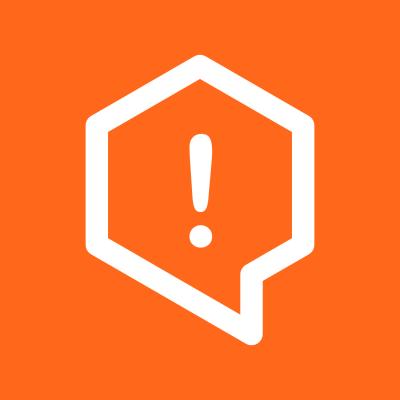
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@fingerprintjs/fingerprintjs-pro-server-api
Advanced tools
Node.js wrapper for FingerprintJS Sever API
Node.js wrapper for FingerprintJS Server API
Install package using npm
npm i @fingerprintjs/fingerprintjs-pro-server-api
Or install package using yarn
yarn add @fingerprintjs/fingerprintjs-pro-server-api
import { FingerprintJsServerApiClient, Region } from '@fingerprintjs/fingerprintjs-pro-server-api';
// Init client with the given region and the secret api_key
const client = new FingerprintJsServerApiClient({region: Region.Global, apiKey: "<api_key>"});
// Get visitor history
client.getVisitorHistory("<visitorId>").then(visitorHistory => {
console.log(visitorHistory);
});
const visit = visitWebhookBody as unknown as VisitWebhook;
FingerprintJsServerApiClient({region: Region, apiKey: string})
constructorCreates an instance of the client.
const client = new FingerprintJsServerApiClient({region: Region.EU, apiKey: "<api_key>"});
region: Region
- a region of the server, possible value Region.EU
or Region.Global
apiKey: string
- secret API key from the FingerprintJS dashboardfetch?: typeof fetch
- optional implementation of fetch
function (defaults to node-fetch
)client.getVisitorHistory(visitorId: string, filter?: VisitorHistoryFilter): Promise<VisitorsResponse>
Gets history for the given visitor and given filter, returns a promise with visitor history response.
client.getVisitorHistory("<visitorId>", filter)
.then(visitorHistory => {
console.log(visitorHistory);
})
.catch(error => {
if (error.status === 403) {
console.log(error.error);
} else if (error.status === 429) {
retryLater(error.retryAfter); // this function needs to be implemented on your side
}
});
visitorId: string
- identifier of the visitorfilter?: VisitorHistoryFilter
- visitor history filter, more info in the API documentationPromise<VisitorsResponse>
- promise with visitor history responseVisitorHistoryFilter
Filter for querying API - see query parameters.
const filter = {
request_id: "<request_id>",
linked_id: "<linked_id>",
limit: 5,
before: "<timeStamp>"
};
request_id: string
- filter events by requestIdlinked_id: string
- filter events by custom identifierlimit: number
- limit scanned resultsbefore: number
- used to paginate resultsVisitorsResponse
responseFind more info in the API documentation
{
"visitorId": "Ibk1527CUFmcnjLwIs4A9",
"visits": [
{
"requestId": "0KSh65EnVoB85JBmloQK",
"incognito": true,
"linkedId": "somelinkedId",
"time": "2019-05-21T16:40:13Z",
// timestamp of the event with millisecond precision
"timestamp": 1582299576512,
"url": "https://www.example.com/login",
"ip": "61.127.217.15",
"ipLocation": {
"accuracyRadius": 10,
"latitude": 49.982,
"longitude": 36.2566,
"postalCode": "61202",
"timezone": "Europe/Dusseldorf",
"city": {
"name": "Dusseldorf"
},
"continent": {
"code": "EU",
"name": "Europe"
},
"country": {
"code": "DE",
"name": "Germany"
},
"subdivisions": [
{
"isoCode": "63",
"name": "North Rhine-Westphalia"
}
],
},
"browserDetails": {
"browserName": "Chrome",
"browserMajorVersion": "74",
"browserFullVersion": "74.0.3729",
"os": "Windows",
"osVersion": "7",
"device": "Other",
"userAgent": "Mozilla/5.0 (Windows NT 6.1; Win64; x64) ....",
},
"confidence": {
"score": 0.97
},
"visitorFound": true,
"firstSeenAt": {
"global": "2022-03-16T11:26:45.362Z",
"subscription": "2022-03-16T11:31:01.101Z"
},
"lastSeenAt": {
"global": "2022-03-16T11:28:34.023Z",
"subscription": null
}
}
],
// optional, if more results are available for pagination.
"lastTimestamp": 1582299576512
}
client.getEvent(requestId: string): Promise<EventResponse>
Get events with all the information from each activated product - Bot Detection and Identification.
client.getEvent("<requestId>")
.then(eventInfo => {
console.log(eventInfo);
})
.catch(error => {
if (error.status === 403 || error.status === 404) {
console.log(error.code, error.message);
}
});
requestId: string
- identifier of the eventPromise<EventResponse>
- promise with event responseEventResponse
responseFind more info in the API documentation.
{
"products": {
"identification": {
"data": {
"visitorId": "Ibk1527CUFmcnjLwIs4A9",
"requestId": "0KSh65EnVoB85JBmloQK",
"incognito": true,
"linkedId": "somelinkedId",
"time": "2019-05-21T16:40:13Z",
"timestamp": 1582299576512,
"url": "https://www.example.com/login",
"ip": "61.127.217.15",
"ipLocation": {
"accuracyRadius": 10,
"latitude": 49.982,
"longitude": 36.2566,
"postalCode": "61202",
"timezone": "Europe/Dusseldorf",
"city": {
"name": "Dusseldorf"
},
"continent": {
"code": "EU",
"name": "Europe"
},
"country": {
"code": "DE",
"name": "Germany"
},
"subdivisions": [
{
"isoCode": "63",
"name": "North Rhine-Westphalia"
}
],
},
"browserDetails": {
"browserName": "Chrome",
"browserMajorVersion": "74",
"browserFullVersion": "74.0.3729",
"os": "Windows",
"osVersion": "7",
"device": "Other",
"userAgent": "Mozilla/5.0 (Windows NT 6.1; Win64; x64) ....",
},
"confidence": {
"score": 0.97
},
"visitorFound": true,
"firstSeenAt": {
"global": "2022-03-16T11:26:45.362Z",
"subscription": "2022-03-16T11:31:01.101Z"
},
"lastSeenAt": {
"global": "2022-03-16T11:28:34.023Z",
"subscription": null
}
}
},
"botd": {
"data": {
"bot": {
"result": "notDetected"
},
"url": "https://example.com/login",
"ip": "61.127.217.15",
"time": "2019-05-21T16:40:13Z"
}
}
}
}
getEvent
and getVisitorHistory
methods return generic types Promise<EventResponse>
and Promise<VisitorsResponse>
and throw EventError
and VisitorsError
.
You can use typeguards to narrow error types as in example below.
import { isVisitorsError, isEventError } from '@fingerprintjs/fingerprintjs-pro-server-api';
client
.getVisitorHistory("<visitorId>", filter)
.then(result => console.log(result))
.catch(err => {
if (isVisitorsError(err)) {
if (err.code === 429) {
// VisitorsError429 type
retryLater(err.retryAfter); // this function needs to be implemented on your side
} else {
console.log('error: ', err.error)
}
} else {
console.log('unknown error: ', err)
}
});
client
.getEvent("<requestId>")
.then(result => console.log(result))
.catch(err => {
if (isEventError(err)) {
console.log(`error ${err.code}: `, err.error.message)
} else {
console.log('unknown error: ', err)
}
});
FAQs
Node.js wrapper for FingerprintJS Sever API
We found that @fingerprintjs/fingerprintjs-pro-server-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.