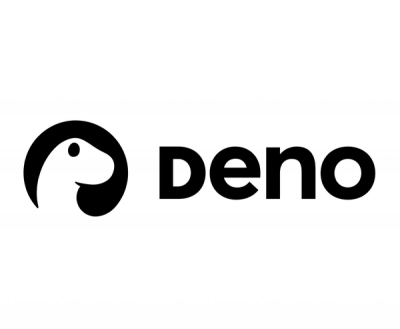
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@fireact.dev/core
Advanced tools
Core components and utilities for Fireact applications.
npm install @fireact.dev/core
Install the required peer dependencies:
npm install firebase react-router-dom i18next react-i18next @headlessui/react@^1.7.15 @heroicons/react tailwindcss i18next-browser-languagedetector
Note: Make sure to use @headlessui/react version ^1.7.15 as it's a required peer dependency.
npx tailwindcss init
tailwind.config.js
:/** @type {import('tailwindcss').Config} */
module.exports = {
content: [
"./src/**/*.{js,jsx,ts,tsx}",
"./node_modules/@fireact.dev/core/dist/**/*.{js,mjs}"
],
theme: {
extend: {},
},
plugins: [],
}
src/index.css
:@tailwind base;
@tailwind components;
@tailwind utilities;
Create src/config.json
with your Firebase configuration:
{
"firebase": {
"apiKey": "your-api-key",
"authDomain": "your-auth-domain",
"projectId": "your-project-id",
"storageBucket": "your-storage-bucket",
"messagingSenderId": "your-messaging-sender-id",
"appId": "your-app-id"
},
"pages": {
"home": "/",
"dashboard": "/dashboard",
"profile": "/profile",
"editName": "/edit-name",
"editEmail": "/edit-email",
"changePassword": "/change-password",
"deleteAccount": "/delete-account",
"signIn": "/signin",
"signUp": "/signup",
"resetPassword": "/reset-password"
},
"socialLogin": {
"google": false,
"microsoft": false,
"facebook": false,
"apple": false,
"github": false,
"twitter": false,
"yahoo": false
}
}
Note: The ConfigProvider will automatically initialize Firebase using this configuration.
To enable social login providers:
true
in the socialLogin
section of your config.jsonsrc/
i18n/
locales/
en.ts
zh.ts
Download the base language files from: https://github.com/chaoming/fireact/tree/main/src/i18n/locales
Adding or modifying labels: Each language file (e.g., en.ts) follows this structure:
export default {
email: "Email",
password: "Password",
// Add more labels as needed
}
fr.ts
for French)i18n
.use(LanguageDetector)
.use(initReactI18next)
.init({
resources: {
en: {
translation: en
},
zh: {
translation: zh
},
fr: {
translation: fr // Add your new language here
}
},
fallbackLng: 'en',
interpolation: {
escapeValue: false
}
});
import { useTranslation } from 'react-i18next';
function YourComponent() {
const { t } = useTranslation();
return (
<div>
<h1>{t('common.title')}</h1>
<p>{t('common.description')}</p>
</div>
);
}
src/main.tsx
:import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App';
import './index.css';
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
<App />
</React.StrictMode>
);
src/App.tsx
:import { BrowserRouter as Router, Routes, Route, Navigate } from 'react-router-dom';
import {
AuthProvider,
ConfigProvider,
LoadingProvider,
PublicLayout,
AuthenticatedLayout,
SignIn,
SignUp,
ResetPassword,
Dashboard,
Profile,
EditName,
EditEmail,
ChangePassword,
DeleteAccount,
DesktopMenuItems,
MobileMenuItems,
Logo
} from '@fireact.dev/core';
import config from './config.json';
import i18n from 'i18next';
import { initReactI18next } from 'react-i18next';
import LanguageDetector from 'i18next-browser-languagedetector';
import en from './i18n/locales/en';
import zh from './i18n/locales/zh';
// Initialize i18next
i18n
.use(LanguageDetector)
.use(initReactI18next)
.init({
resources: {
en: {
translation: en
},
zh: {
translation: zh
}
},
fallbackLng: 'en',
interpolation: {
escapeValue: false
}
});
function App() {
return (
<Router>
<ConfigProvider config={config}>
<AuthProvider>
<LoadingProvider>
<Routes>
<Route element={
<AuthenticatedLayout
desktopMenuItems={<DesktopMenuItems />}
mobileMenuItems={<MobileMenuItems />}
logo={<Logo className="w-10 h-10" />}
/>
}>
<Route path={config.pages.home} element={<Navigate to={config.pages.dashboard} />} />
<Route path={config.pages.dashboard} element={<Dashboard />} />
<Route path={config.pages.profile} element={<Profile />} />
<Route path={config.pages.editName} element={<EditName />} />
<Route path={config.pages.editEmail} element={<EditEmail />} />
<Route path={config.pages.changePassword} element={<ChangePassword />} />
<Route path={config.pages.deleteAccount} element={<DeleteAccount />} />
</Route>
<Route element={<PublicLayout logo={<Logo className="w-20 h-20" />} />}>
<Route path={config.pages.signIn} element={<SignIn />} />
<Route path={config.pages.signUp} element={<SignUp />} />
<Route path={config.pages.resetPassword} element={<ResetPassword />} />
</Route>
</Routes>
</LoadingProvider>
</AuthProvider>
</ConfigProvider>
</Router>
);
}
export default App;
npm install -g firebase-tools
firebase login
firebase init
Select the following options:
npm run build
firebase deploy
Your app will be available at:
MIT
FAQs
A comprehensive React component library and utility collection for building Firebase-powered applications. Features authentication components, Firebase integration utilities, and TailwindCSS-styled UI elements.
We found that @fireact.dev/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.