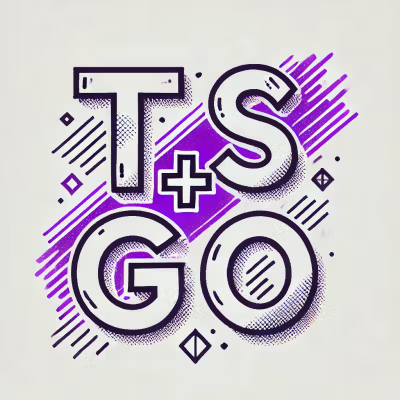
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@firebase/auth
Advanced tools
The @firebase/auth package is part of the Firebase JavaScript SDK, providing robust authentication functionality for web and mobile applications. It supports various authentication methods, including email and password, third-party providers like Google and Facebook, and more. This package helps in managing user authentication states, securing user data, and integrating with other Firebase services.
Email and Password Authentication
Allows users to sign up using their email and password. It also handles user sign-in and management of user sessions.
firebase.auth().createUserWithEmailAndPassword(email, password).then((userCredential) => { var user = userCredential.user; }).catch((error) => { var errorCode = error.code; var errorMessage = error.message; });
Social Auth Providers
Supports authentication with different social media providers such as Google, Facebook, Twitter, etc. This example uses Google for authentication.
var provider = new firebase.auth.GoogleAuthProvider(); firebase.auth().signInWithPopup(provider).then((result) => { var token = result.credential.accessToken; var user = result.user; }).catch((error) => { var errorCode = error.code; var errorMessage = error.message; });
Phone Number Authentication
Enables sign-in using a phone number with SMS verification. This method sends an SMS to the user's phone number with a verification code.
firebase.auth().signInWithPhoneNumber(phoneNumber, appVerifier).then((confirmationResult) => { var verificationCode = window.prompt('Please enter the verification code that was sent to your mobile device.'); return confirmationResult.confirm(verificationCode); }).catch((error) => { var errorCode = error.code; var errorMessage = error.message; });
Passport is an authentication middleware for Node.js. Unlike @firebase/auth which is tightly integrated with Firebase services, Passport works with any type of application and supports extensive authentication mechanisms through strategies.
Auth0 is a flexible, drop-in solution to add authentication and authorization services to your applications. It provides a broader set of features compared to @firebase/auth, including advanced user management, multi-factor authentication, and extensive integration options.
This is the authentication component for the Firebase JS SDK. It has a peer
dependency on the @firebase/app
package on NPM. This package
is included by default in the firebase
wrapper
package.
To set up a development environment to build Firebase-auth from source, you must have the following installed:
In order to run the tests, you must also have:
Download the Firebase source and its dependencies with:
git clone https://github.com/firebase/firebase-js-sdk.git
cd firebase-js-sdk
yarn install
To build the library, run:
cd packages/auth
yarn build
This will create output files in the dist/
folder.
All unit tests can be run on the command line (via Chrome and Firefox) with:
yarn test
Alternatively, the unit tests can be run manually by running
yarn run serve
Then, all unit tests can be run at: http://localhost:4000/buildtools/all_tests.html
You can also run tests individually by accessing each HTML file under
generated/tests
, for example: http://localhost:4000/generated/tests/test/auth_test.html
You need a SauceLabs account to run tests on SauceLabs.
Go to your SauceLab account, under "My Account", and copy paste the access key. Now export the following variables, in two Terminal windows:
export SAUCE_USERNAME=<your username>
export SAUCE_ACCESS_KEY=<the copy pasted access key>
Then, in one Terminal window, start SauceConnect:
./buildtools/sauce_connect.sh
Take note of the "Tunnel Identifier" value logged in the terminal, at the top. In the other terminal that has the exported variables, run the tests:
yarn test -- --saucelabs --tunnelIdentifier=<the tunnel identifier>
FAQs
The Firebase Authenticaton component of the Firebase JS SDK.
The npm package @firebase/auth receives a total of 1,590,364 weekly downloads. As such, @firebase/auth popularity was classified as popular.
We found that @firebase/auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.