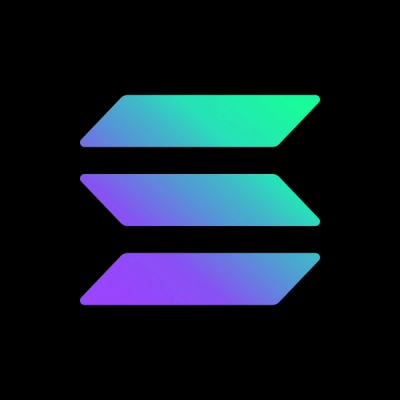
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
@flex-development/errnode
Advanced tools
Universal API for creating Node.js errors
ERR_AMBIGUOUS_ARGUMENT(reason)
ERR_ARG_NOT_ITERABLE(name)
ERR_ASYNC_CALLBACK(name)
ERR_ILLEGAL_CONSTRUCTOR()
ERR_IMPORT_ASSERTION_TYPE_FAILED(id, type)
ERR_IMPORT_ASSERTION_TYPE_MISSING(id, type)
ERR_IMPORT_ASSERTION_TYPE_UNSUPPORTED(type)
ERR_INCOMPATIBLE_OPTION_PAIR(option1, option2)
ERR_INVALID_ARG_TYPE(name, expected, actual)
ERR_INVALID_ARG_VALUE(name, value[, reason])
ERR_INVALID_MODULE_SPECIFIER(request[, reason][, base])
ERR_INVALID_PACKAGE_CONFIG(id[, base][, reason])
ERR_INVALID_PACKAGE_TARGET(dir, key, target[, internal][, base])
ERR_METHOD_NOT_IMPLEMENTED(method)
ERR_MISSING_OPTION(option)
ERR_MODULE_NOT_FOUND(id, base[, type])
ERR_NETWORK_IMPORT_DISALLOWED(specifier, base, reason)
ERR_OPERATION_FAILED(reason)
ERR_PACKAGE_IMPORT_NOT_DEFINED(specifier, base[, dir])
ERR_PACKAGE_PATH_NOT_EXPORTED(dir, subpath[, base])
ERR_UNHANDLED_ERROR([err])
ERR_UNKNOWN_ENCODING(encoding)
ERR_UNKNOWN_FILE_EXTENSION(ext, id[, suggestion])
ERR_UNKNOWN_MODULE_FORMAT(format, id)
ERR_UNSUPPORTED_DIR_IMPORT(id, base)
ERR_UNSUPPORTED_ESM_URL_SCHEME(url, supported[, windows])
This package provides a universal API for creating Node.js errors.
This package is designed to help developers build Node.js tools like ponyfills, as well as more verbose tools like
mlly
, by providing a set of utilities and constructor functions for creating Node.js errors.
Error.stackTraceLimit
to
capture larger stack traces. This is not implemented to maintain browser compatibility.This package is ESM only.
yarn add @flex-development/errnode
From Git:
yarn add @flex-development/errnode@flex-development/errnode
See Git - Protocols | Yarn for details on requesting a specific branch, commit, or tag.
import {
createNodeError,
ErrorCode,
type MessageFn,
type NodeError,
type NodeErrorConstructor
} from '@flex-development/errnode'
/**
* [`ERR_INVALID_URL`][1] schema.
*
* [1]: https://nodejs.org/api/errors.html#err_invalid_url
*
* @extends {NodeError<TypeError>}
*/
interface ErrInvalidUrl extends NodeError<TypeError> {
/**
* Error code.
*/
code: ErrorCode.ERR_INVALID_URL
/**
* URL that failed to parse.
*
* @example
* 'http://[127.0.0.1\x00c8763]:8000/'
*/
input: string
}
/**
* `ERR_INVALID_URL` model.
*
* Thrown when an invalid URL is passed to a [WHATWG][1] [`URL` constructor][2]
* or [`url.parse()`][3] to be parsed.
*
* [1]: https://nodejs.org/api/url.html#the-whatwg-url-api
* [2]: https://nodejs.org/api/url.html#new-urlinput-base
* [3]: https://nodejs.org/api/url.html#urlparseurlstring-parsequerystring-slashesdenotehost
*
* @see https://nodejs.org/api/errors.html#err_invalid_url
*
* @class
*
* @param {string} input - URL that failed to parse
* @return {ErrInvalidUrl} `TypeError` instance
*/
const ERR_INVALID_URL: NodeErrorConstructor<
TypeErrorConstructor,
MessageFn<[string]>,
ErrInvalidUrl
> = createNodeError(
ErrorCode.ERR_INVALID_URL,
TypeError,
/**
* Creates an [`ERR_INVALID_URL`][1] message.
*
* [1]: https://nodejs.org/api/errors.html#err_invalid_url
*
* @see https://github.com/nodejs/node/blob/v19.3.0/lib/internal/errors.js#L1381-L1386
*
* @this {ErrInvalidUrl}
*
* @param {string} input - URL that failed to parse
* @return {string} Error message
*/
function msg(this: ErrInvalidUrl, input: string): string {
this.input = input
return 'Invalid URL'
}
)
/**
* URL that will fail to parse.
*
* @const {string} BAD_URL
*/
const BAD_URL: string = 'http://[127.0.0.1\x00c8763]:8000/'
/**
* {@linkcode ERR_INVALID_URL} instance.
*
* @const {ErrInvalidUrl} err
*/
const err: ErrInvalidUrl = new ERR_INVALID_URL(BAD_URL)
console.error(err)
console.debug('err instanceof TypeError:', err instanceof TypeError)
...running that yields:
TypeError [ERR_INVALID_URL]: Invalid URL
at new NodeError (file:////home/runner/work/errnode/errnode/src/create-node-error.ts:103:5)
at file:////home/runner/work/errnode/errnode/scratch.ts:90:28
at ModuleJob.run (node:internal/modules/esm/module_job:193:25)
at async Promise.all (index 0)
at async ESMLoader.import (node:internal/modules/esm/loader:533:24)
at async loadESM (node:internal/process/esm_loader:91:5)
at async handleMainPromise (node:internal/modules/run_main:65:12) {
code: 'ERR_INVALID_URL',
input: 'http://[127.0.0.1\x00c8763]:8000/'
}
err instanceof TypeError: true
This package exports the following identifiers:
ERR_AMBIGUOUS_ARGUMENT
ERR_ARG_NOT_ITERABLE
ERR_ASYNC_CALLBACK
ERR_ILLEGAL_CONSTRUCTOR
ERR_IMPORT_ASSERTION_TYPE_FAILED
ERR_IMPORT_ASSERTION_TYPE_MISSING
ERR_IMPORT_ASSERTION_TYPE_UNSUPPORTED
ERR_INCOMPATIBLE_OPTION_PAIR
ERR_INVALID_ARG_TYPE
ERR_INVALID_ARG_VALUE
ERR_INVALID_MODULE_SPECIFIER
ERR_INVALID_PACKAGE_CONFIG
ERR_INVALID_PACKAGE_TARGET
ERR_METHOD_NOT_IMPLEMENTED
ERR_MISSING_OPTION
ERR_MODULE_NOT_FOUND
ERR_NETWORK_IMPORT_DISALLOWED
ERR_OPERATION_FAILED
ERR_PACKAGE_IMPORT_NOT_DEFINED
ERR_PACKAGE_PATH_NOT_EXPORTED
ERR_UNHANDLED_ERROR
ERR_UNKNOWN_ENCODING
ERR_UNKNOWN_FILE_EXTENSION
ERR_UNKNOWN_MODULE_FORMAT
ERR_UNSUPPORTED_DIR_IMPORT
ERR_UNSUPPORTED_ESM_URL_SCHEME
createNodeError
determineSpecificType
errors
There is no default export.
Constructor functions representing Node.js error codes, callable with and without the new
keyword. Constructor
arguments are used to generate error messages.
Models can be imported individually:
import {
ERR_INVALID_ARG_VALUE,
ERR_INVALID_MODULE_SPECIFIER,
ERR_INVALID_PACKAGE_CONFIG,
ERR_INVALID_PACKAGE_TARGET,
ERR_MODULE_NOT_FOUND,
ERR_NETWORK_IMPORT_DISALLOWED,
ERR_PACKAGE_IMPORT_NOT_DEFINED,
ERR_PACKAGE_PATH_NOT_EXPORTED,
ERR_UNKNOWN_FILE_EXTENSION,
ERR_UNSUPPORTED_DIR_IMPORT,
ERR_UNSUPPORTED_ESM_URL_SCHEME
} from '@flex-development/errnode'
...or all at once using the errors
export:
import { errors } from '@flex-development/errnode'
Note: This package does not export a model for every error code. Submit a feature request (or pull request if
you're up for the challenge :wink:) to add a model. For more fine-grained control, however, use
createNodeError
instead.
ERR_AMBIGUOUS_ARGUMENT(name, reason)
Thrown when a function argument is being used in a way that suggests that the function signature may be misunderstood.
{string}
name
— Name of ambiguous argument{string}
reason
— Reason name
is ambiguous{NodeError<TypeError>}
ERR_ARG_NOT_ITERABLE(name)
Thrown when an iterable argument (i.e. a value that works with for...of
loops) is required, but not provided to a
Node.js API.
{string}
name
— Name of non-iterable argument{NodeError<TypeError>}
ERR_ASYNC_CALLBACK(name)
Thrown when an attempt is made to register something that is not a function as an AsyncHooks
callback.
{string}
name
— Name of argument that must be a function{NodeError<TypeError>}
Source:
src/models/err-async-callback.ts
ERR_ILLEGAL_CONSTRUCTOR()
Thrown when an attempt is made to construct an object using a non-public constructor.
{NodeError<TypeError>}
ERR_IMPORT_ASSERTION_TYPE_FAILED(id, type)
Thrown when an import assertion has failed, preventing the specified module from being imported.
{string}
id
— Id of module that cannot be imported{string}
type
— Invalid import assertion type{NodeError<TypeError>}
ERR_IMPORT_ASSERTION_TYPE_MISSING(id, type)
Thrown when an import assertion is missing, preventing the specified module from being imported.
{string}
id
— Id of module that cannot be imported{string}
type
— Invalid import assertion type{NodeError<TypeError>}
ERR_IMPORT_ASSERTION_TYPE_UNSUPPORTED(type)
Thrown when an import assertion is not supported by a version of Node.js.
{string}
type
— Unsupported import assertion type{NodeError<TypeError>}
ERR_INCOMPATIBLE_OPTION_PAIR(option1, option2)
Thrown when an option pair is incompatible with each other and cannot be used at the same time.
{string}
option1
— Option that cannot be used{string}
option2
— Option that is incompatible with option1
{NodeError<TypeError>}
ERR_INVALID_ARG_TYPE(name, expected, actual)
Thrown when an argument of the wrong type is passed to a Node.js API.
{string}
name
— Name of invalid argument or property{OneOrMany<string>}
expected
— Expected type(s){unknown}
actual
— Value supplied by user{NodeError<TypeError>}
ERR_INVALID_ARG_VALUE(name, value[, reason])
Thrown when an invalid or unsupported value is passed for a given argument or property.
{string}
name
— Name of invalid argument or property{unknown}
value
— Value supplied by user{string?}
[reason='is invalid']
— Reason value
is invalid{NodeError<TypeError>}
ERR_INVALID_MODULE_SPECIFIER(request[, reason][, base])
Thrown when an imported module string is an invalid URL, package name, or package subpath specifier.
{string}
request
— Invalid module specifier{string?}
[reason='']
— Reason request
is invalid{string?}
[base='']
— Id of module request
was imported from{NodeError<TypeError>}
ERR_INVALID_PACKAGE_CONFIG(id[, base][, reason])
Thrown when a package.json
file fails parsing.
{string}
id
— Location of invalid package.json
file{string?}
[base='']
— Id of module being imported. May also include where module is being imported from{string?}
[reason='']
— Reason package config is invalid{NodeError<Error>}
ERR_INVALID_PACKAGE_TARGET(dir, key, target[, internal][, base])
Thrown when a package.json
"exports"
or "imports"
field contains an invalid target mapping value for
the attempted module resolution.
{string}
dir
— Id of directory containing package.json
{string}
key
— "exports"
or "imports"
key{unknown}
target
— Invalid package target{boolean?}
[internal=false]
— target
is "imports"
?{string?}
[base='']
— Id of module package.json
was imported from{NodeError<Error>}
ERR_METHOD_NOT_IMPLEMENTED(method)
Thrown when a method is required but not implemented.
{string}
method
— Method name{NodeError<Error>}
ERR_MISSING_OPTION(option)
Thrown when a required option is missing. For APIs that accept options objects, some options might be mandatory.
{string}
option
— Option name{NodeError<TypeError>}
Source:
src/models/err-missing-option.ts
ERR_MODULE_NOT_FOUND(id, base[, type])
Thrown when a module file cannot be resolved by the ECMAScript modules loader while attempting an import
operation or
when loading a program entry point.
{string}
id
— Id of missing module{string}
base
— Id of module id
was imported from{string?}
[type='package']
— Module file type{NodeError<Error>}
ERR_NETWORK_IMPORT_DISALLOWED(specifier, base, reason)
Thrown when a network module attempts to load another module that it is not allowed to load.
{string}
specifier
— Invalid module specifier{string}
base
— Id of module specifier
was imported from{string}
reason
— Reason for error{NodeError<Error>}
ERR_OPERATION_FAILED(reason)
Thrown when an operation has failed. Typically used to signal the general failure of an asynchronous operation.
{string}
reason
— Reason for operation failure{NodeError<Error>}
ERR_PACKAGE_IMPORT_NOT_DEFINED(specifier, base[, dir])
Thrown when a package.json
"imports"
field does not define the given package import specifier.
{string}
specifier
— Invalid package import specifier{string}
base
— Id of module specifier
was imported from{string?}
[dir='']
— Id of directory containing package.json
{NodeError<TypeError>}
ERR_PACKAGE_PATH_NOT_EXPORTED(dir, subpath[, base])
Thrown when a package.json
"exports"
field does not export the requested subpath.
{string}
dir
— Id of directory containing package.json
{string}
subpath
— Requested subpath{string?}
[base='']
— Id of module subpath
was imported from{NodeError<Error>}
ERR_UNHANDLED_ERROR([err])
Thrown when an unhandled error occurs.
{string?}
[err='']
— Stringified error{NodeError<Error>}
ERR_UNKNOWN_ENCODING(encoding)
Thrown when an invalid or unknown encoding option is passed to a Node.js API.
{string}
encoding
— Invalid or unknown encoding{NodeError<TypeError>}
ERR_UNKNOWN_FILE_EXTENSION(ext, id[, suggestion])
Thrown when an attempt is made to load a module with an unknown or unsupported file extension.
{string}
ext
— Unknown or unsupported file extension{string}
id
— Id of module containing ext
{string?}
[suggestion='']
— Recommended fix{NodeError<TypeError>}
ERR_UNKNOWN_MODULE_FORMAT(format, id)
Thrown when an attempt is made to load a module with an unknown or unsupported format.
{string}
format
— Unknown or unsupported format{string}
id
— Id of module with format
{NodeError<RangeError>}
ERR_UNSUPPORTED_DIR_IMPORT(id, base)
Thrown when a directory URL is import
ed.
{string}
id
— Module id of directory{string}
base
— Id of module id
was imported from{NodeError<Error>}
ERR_UNSUPPORTED_ESM_URL_SCHEME(url, supported[, windows])
Thrown when an unsupported URL scheme is used in an import
statement. URL schemes other than file
and data
are unsupported.
{URL}
url
— URL containing unsupported scheme{string[]}
supported
— Supported URL schemes{boolean?}
[windows=false]
— Windows operating system?{NodeError<Error>}
createNodeError(code, Base, message)
Creates a Node.js error constructor.
If the given error message
is a function, constructor arguments are passed to message
. If the message
is a string,
constructor arguments are passed to util.format
instead.
{ErrorCode}
code
— Node.js error code{B extends ErrorConstructor}
Base
— Error base class{M extends any[] | MessageFn | string}
message
— Error message or message function{NodeErrorConstructor<B, M>}
NodeError
constructorSource:
src/create-node-error.ts
determineSpecificType(value)
Determines the specific type of a value for type-mismatch errors.
{unknown}
value
— Value to evaluate{string}
Specific type of value
Source:
src/determine-specific-type.ts
This package is fully typed with TypeScript. It exports the following definitions:
See CONTRIBUTING.md
.
1.2.0 (2023-01-01)
1c910df
] internal: formatList
679af9d
] models: ERR_AMBIGUOUS_ARGUMENT
2404e1e
] models: ERR_ARG_NOT_ITERABLE
567b160
] models: ERR_ASYNC_CALLBACK
94d0441
] models: ERR_ILLEGAL_CONSTRUCTOR
9807b0e
] models: ERR_IMPORT_ASSERTION_TYPE_FAILED
73d9a5c
] models: ERR_IMPORT_ASSERTION_TYPE_MISSING
2f6dfec
] models: ERR_IMPORT_ASSERTION_TYPE_UNSUPPORTED
573fb2f
] models: ERR_INCOMPATIBLE_OPTION_PAIR
d3dc012
] models: ERR_INVALID_ARG_TYPE
6637aaa
] models: ERR_INVALID_ARG_VALUE
e64f15c
] models: ERR_INVALID_MODULE_SPECIFIER
9b8b755
] models: ERR_INVALID_PACKAGE_CONFIG
e162298
] models: ERR_INVALID_PACKAGE_TARGET
f5754f0
] models: ERR_METHOD_NOT_IMPLEMENTED
4ab0472
] models: ERR_MISSING_OPTION
028aa6b
] models: ERR_MODULE_NOT_FOUND
d767ad1
] models: ERR_NETWORK_IMPORT_DISALLOWED
7a72d79
] models: ERR_OPERATION_FAILED
c86086f
] models: ERR_PACKAGE_IMPORT_NOT_DEFINED
f744782
] models: ERR_PACKAGE_PATH_NOT_EXPORTED
61ed62a
] models: ERR_UNHANDLED_ERROR
d562655
] models: ERR_UNKNOWN_ENCODING
aeed292
] models: ERR_UNKNOWN_FILE_EXTENSION
0c4c702
] models: ERR_UNKNOWN_MODULE_FORMAT
c29c652
] models: ERR_UNSUPPORTED_DIR_IMPORT
9a43317
] models: ERR_UNSUPPORTED_ESM_URL_SCHEME
6d9eb1e
] ts: [NodeErrorConstructor
] allow custom util.format
args type7547e7e
] [eslint] update test file patterns56c56f6
] github: add commit scope models
22a1b6a
] github: add commit scope utils
07425e6
] github: add label scope:models
dfd99ee
] github: add label scope:utils
2ee1aed
] vscode: update settingsd453fa8
] utils: [createNodeError
] access this.code
from message
6fc7ad6
] utils: add utils
directory8ef9a81
] models: reorganize instance testsFAQs
Universal API for creating Node.js errors
The npm package @flex-development/errnode receives a total of 22 weekly downloads. As such, @flex-development/errnode popularity was classified as not popular.
We found that @flex-development/errnode demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.