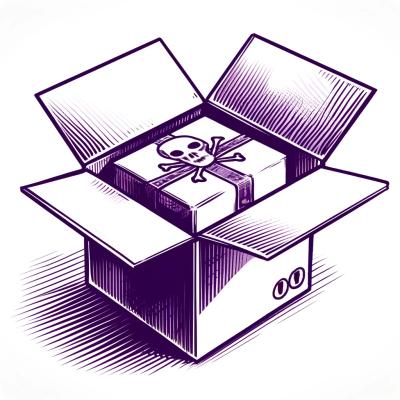
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@fluree/crypto-base
Advanced tools
A collection of Javascript cryptography functions for Fluree.
npm install @fluree/crypto-base
string
string
Normalizes string using the NKFC standard to ensure consistent hashing.
const normalApple = crypto.normalize_string("\u0041\u030apple")
For example, we can see that:
crypto.sha2_256("\u0041\u030apple")
results in 6e9288599c1ff90127459f82285327c83fa0541d8b7cd215d0cd9e587150c15f
.
While using the normalized version of the string
crypto.sha2_256_normalize(normalApple)
results in 58acf888b520fe51ecc0e4e5eef46c3bea3ca7df4c11f6719a1c2471bbe478bf
.
string
or byte-array
byte-array
This functions normalizes a string and returns a byte-array. If it is already a byte-array, it returns itself.
For example:
crypto.string__GT_byte_array("hi there")
results in [104, 105, 32, 116, 104, 101, 114, 101]
.
byte-array
byte-array
This functions takes a byte-array and returns a string.
crypto.byte_array__GT_string([104, 105, 32, 116, 104, 101, 114, 101])
results in hi there
.
none
or private-key-as-hex-string
{ private: "private-key-as-hex-string", public: "public-key-as-hex-string" }
This function will return a map with a public and private key:
crypto.generate_key_pair();
will return a valid public-private key pair.
For example, this might return:
{ private: "6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2", public: "02991719b37817f6108fc8b0e824d3a9daa3d39bc97ecfd4f8bc7ef3b71d4c6391"}
.
You can also call this function with a private key already provided.
crypto.generate_key_pair("6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2");
This will return:
{ private: "6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2", public: "02991719b37817f6108fc8b0e824d3a9daa3d39bc97ecfd4f8bc7ef3b71d4c6391"}
.
private-key-as-hex-string
public-key-as-hex-string
Given a private key, this returns a public key:
crypto.pub_key_from_private("6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2");
This will return: 02991719b37817f6108fc8b0e824d3a9daa3d39bc97ecfd4f8bc7ef3b71d4c6391
;
private-key-as-hex-string
account-id
Given a private key, this will return an account id:
crypto.account_id_from_private("6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2");
This will return TfGvAdKH2nRdV4zP4yBz4kJ2R9WzYHDe2EV
.
public-key-as-hex-string
account-id
Given a public key, this will return an account id:
crypto.account_id_from_public("02991719b37817f6108fc8b0e824d3a9daa3d39bc97ecfd4f8bc7ef3b71d4c6391");
This will return TfGvAdKH2nRdV4zP4yBz4kJ2R9WzYHDe2EV
.
message, private-key-as-hex-string
signature
Given a message and a private key, this will return a signature.
const message = "hi there";
const privateKey = "6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2";
crypto.sign_message(message, privateKey);
This returns:
1b3046022100cbd32e463567fefc2f120425b0224d9d263008911653f50e83953f47cfbef3bc022100fcf81206277aa1b86d2667b4003f44643759b8f4684097efd92d56129cd89ea8
public-key-as-hex-string, message, signature
true
or false
Given a public key, message, and a signature, this function will return true or false.
const message = "hi there";
const privateKey = "6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2";
const publicKey = "02991719b37817f6108fc8b0e824d3a9daa3d39bc97ecfd4f8bc7ef3b71d4c6391";
const signature = crypto.sign_message(message, privateKey);
crypto.verify_signature(publicKey, message, signature);
This returns true
.
message, signature
true
or false
const message = "hi there";
const signature = crypto.sign_message(message, "6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2");
crypto.pub_key_from_message(message, signature);
This returns 02991719b37817f6108fc8b0e824d3a9daa3d39bc97ecfd4f8bc7ef3b71d4c6391
.
message, signature
true
or false
const message = "hi there";
const signature = crypto.sign_message(message, "6a5f415f49986006815ae7887016275aac8ffb239f9a2fa7172300578582b6c2");
crypto.account_id_from_message(message, signature);
This returns TfGvAdKH2nRdV4zP4yBz4kJ2R9WzYHDe2EV
.
string or byte-array
or string or byte-array, output-format
or input in input format, output-format, input-format
hex-string
.Valid input and output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha2_256("hi");
returns:
8f434346648f6b96df89dda901c5176b10a6d83961dd3c1ac88b59b2dc327aa4
.
string
or string, output-format
hex-string
.Valid output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha2_256_normalize("hi")
returns:
8f434346648f6b96df89dda901c5176b10a6d83961dd3c1ac88b59b2dc327aa4
.
string or byte-array
or string or byte-array, output-format
or input in input format, output-format, input-format
hex-string
.Valid input and output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha2_512("hi");
returns:
150a14ed5bea6cc731cf86c41566ac427a8db48ef1b9fd626664b3bfbb99071fa4c922f33dde38719b8c8354e2b7ab9d77e0e67fc12843920a712e73d558e197
.
string
or string, output-format
hex-string
.Valid output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha2_512_normalize("hi");
returns:
150a14ed5bea6cc731cf86c41566ac427a8db48ef1b9fd626664b3bfbb99071fa4c922f33dde38719b8c8354e2b7ab9d77e0e67fc12843920a712e73d558e197
.
string or byte-array
or string or byte-array, output-format
hex-string
.Valid output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha3_256("hi");
returns:
crypto
b39c14c8da3b23811f6415b7e0b33526d7e07a46f2cf0484179435767e4a8804
.
string
or string, output-format
hex-string
.Valid output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha3_256_normalize("hi");
returns:
b39c14c8da3b23811f6415b7e0b33526d7e07a46f2cf0484179435767e4a8804
.
string or byte-array
or string or byte-array, output-format
hex-string
.Valid output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha3_512("hi");
returns:
154013cb8140c753f0ac358da6110fe237481b26c75c3ddc1b59eaf9dd7b46a0a3aeb2cef164b3c82d65b38a4e26ea9930b7b2cb3c01da4ba331c95e62ccb9c3
.
string
or string, output-format
hex string
.Valid output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.sha3_512_normalize("hi");
returns:
154013cb8140c753f0ac358da6110fe237481b26c75c3ddc1b59eaf9dd7b46a0a3aeb2cef164b3c82d65b38a4e26ea9930b7b2cb3c01da4ba331c95e62ccb9c3
.
string
or string, output-format
or string, output-format, input-format
hex-string
.Valid input or output formats: hex
, bytes
, base64
, base58
, string
.
This function: crypto.ripemd_160("hi");
returns 242485ab6bfd3502bcb3442ea2e211687b8e4d89
.
message, iv, key
or message, iv, key, output-format
hex-string
.Valid output formats: hex
, base64
.
const initialization_vector = [6, 224, 71, 170, 241, 204, 115, 21, 30, 8, 46, 223, 106, 207, 55, 42];
const message = "hi";
const key = "there";
crypto.aes_encrypt(message, initialization_vector, key);
This returns: 668cd07d1a17cc7a8a0390cf017ac7ef
.
x, iv, key
or x, iv, key, output-format
or x, iv, key, output-format, input-format
hex-string
.const initialization_vector = [6, 224, 71, 170, 241, 204, 115, 21, 30, 8, 46, 223, 106, 207, 55, 42];
const message = "hi";
const key = "there";
const encrypted = crypto.aes_encrypt(message, initialization_vector, key);
crypto.aes_decrypt(encrypted, initialization_vector, key);
This returns: hi
.
message
or message, salt
or message, salt, n
or message, salt, n, r, p
or message, salt, n, r, p, dk-len
hex-string
.The arguments by default:
salt
: 16 random bytesn
: 32,768r
: 8p
: 1dk-len
: 32const salt = [172, 28, 242, 108, 175, 130, 214, 6, 249, 61, 244, 178, 34, 8, 13, 178];
crypto.scrypt_encrypt("hi", salt)
This results in 57f93bcf926c31a9e2d2129da84bfca51eb9447dfe1749b62598feacaad657d4
.
message, encrypted, salt
or message, encrypted, salt, n, r, p
true
or false
The arguments by default:
n
: 32,768r
: 8p
: 1const salt = [172, 28, 242, 108, 175, 130, 214, 6, 249, 61, 244, 178, 34, 8, 13, 178];
crypto.scrypt_encrypt("hi", salt, 32768, 8, 1)
crypto.scrypt_check("hi", "57f93bcf926c31a9e2d2129da84bfca51eb9447dfe1749b62598feacaad657d4", salt, 32768, 8, 1)
FAQs
Base cryptography functions that are used in Fluree
The npm package @fluree/crypto-base receives a total of 207 weekly downloads. As such, @fluree/crypto-base popularity was classified as not popular.
We found that @fluree/crypto-base demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.