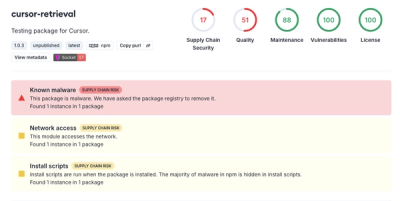
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@fp51/foldable-helpers
Advanced tools
Typescript helpers to fold on sum types
Helpers to fold on things
While we recommend using @fp51/foldable-helpers
with Typescript, it can
be used in standard Javascript apps.
First, install the library:
yarn add @fp51/foldable-helpers
createFold
You have a sum type and the type guards for each of the types. For example:
type T = A | B | C;
function isA(t: T): t is A { ... };
function isB(t: T): t is B { ... };
function isC(t: T): t is C { ... };
To create a fold function to fold on T
, use createFold
import { pipe } from 'fp-ts/es6/pipeable';
import { createFold } from '@fp51/foldable-helpers';
const foldOnT = createFold(isA, isB, isC);
const t: T = ...;
pipe(
t,
foldOnT(
(tbis) => console.log('executed when t is A', { tbis }),
(tbis) => console.log('executed when t is B', { tbis }),
(tbis) => console.log('executed when t is C', { tbis }),
),
);
createFoldObject
Classic fold is very useful but could become hard to read when we have more than 3-4 types to fold on. Because we don't have named parameters in JS/Typescript, we have to use something else.
You still have a sum type and the type guards for each of the types. For example:
type T = A | B | C;
function isA(t: T): t is A { ... };
function isB(t: T): t is B { ... };
function isC(t: T): t is C { ... };
To create a named fold function to fold on T
without loosing readability,
use createFoldObject
. You choose the name of each fold function by passing
an object.
import { pipe } from 'fp-ts/es6/pipeable';
import { createFoldObject } from '@fp51/foldable-helpers';
const foldOnT = createFoldObject({
onA: isA,
onB: isB,
onC: isC
});
const t: T = ...;
pipe(
t,
foldOnT({
onA: (tbis) => console.log('executed when t is A', { tbis }),
onB: (tbis) => console.log('executed when t is B', { tbis }),
onC: (tbis) => console.log('executed when t is C', { tbis }),
}),
);
and
When using fold you will probably encounter cases where a type is a combination
(intersection) of different guards. To reduce the boilerplate having to write
each combination by hand you can use the and
helper.
type A = { a: string };
type B = { b: number };
const isTypeA = (value: any): value is A =>
value != null && typeof value.a === 'string';
const isTypeB = (value: any): value is B =>
value != null && typeof value.b === 'number';
const oldIsTypeAAndB = (value: any): value is A & B =>
isTypeA(value) && isTypeB(value);
// isTypeAAndB :: (t: any) => t is A & B
const isTypeAAndB = and(isTypeA, isTypeB);
or
When using fold you will probably encounter cases where a type is part of an
union of different guards. To reduce the boilerplate having to write each
combination by hand you can use the or
helper.
type A = { a: string };
type B = { b: number };
const isTypeA = (value: any): value is A =>
value != null && typeof value.a === 'string';
const isTypeB = (value: any): value is B =>
value != null && typeof value.b === 'number';
const oldIsTypeAOrB = (value: any): value is A | B =>
isTypeA(value) || isTypeB(value);
// isTypeAOrB :: (t: any) => t is A | B
const isTypeAOrB = or(isTypeA, isTypeB);
not
When using createFold you need to make sure that each guard mutually excludes
the others but it can sometimes be painfull if one type depends on another,
therefore we let you use the not
operator to exclude a guard
type TypeA = { a: string };
type TypeB = { a: 'test' };
const isTypeA = (value: {a: unknown}): value is TypeA => typeof value.a === 'string';
const isTypeB = (value: TypeA): value is TypeB => value.a === 'test';
const fold = createFoldObject({
onTypeA: combineGuards(isTypeA, not(isTypeB)),
onTypeB: isTypeB,
});
FAQs
Typescript helpers to fold on sum types
The npm package @fp51/foldable-helpers receives a total of 111 weekly downloads. As such, @fp51/foldable-helpers popularity was classified as not popular.
We found that @fp51/foldable-helpers demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.